Are you interested in using the ArcGIS API for Python to automate your ArcGIS Online or ArcGIS Enterprise workflow, but are finding the number of different components and software options daunting? The ArcGIS API for Python can seem overwhelming due to the complexity and number of install options, as well as the possibility of different Python environments. The ArcGIS API for Python also integrates third-party products, such as Anaconda and Jupyter Notebook, that may be new to many.
However, the ArcGIS API for Python can be very useful and intuitive, especially when best practices are followed. It can also greatly simplify your scripts. Here is an example script for moving content (reassigning) from one user to another that does not make use of the ArcGIS API for Python. This entails creating text strings for URL requests for every action you want to perform.
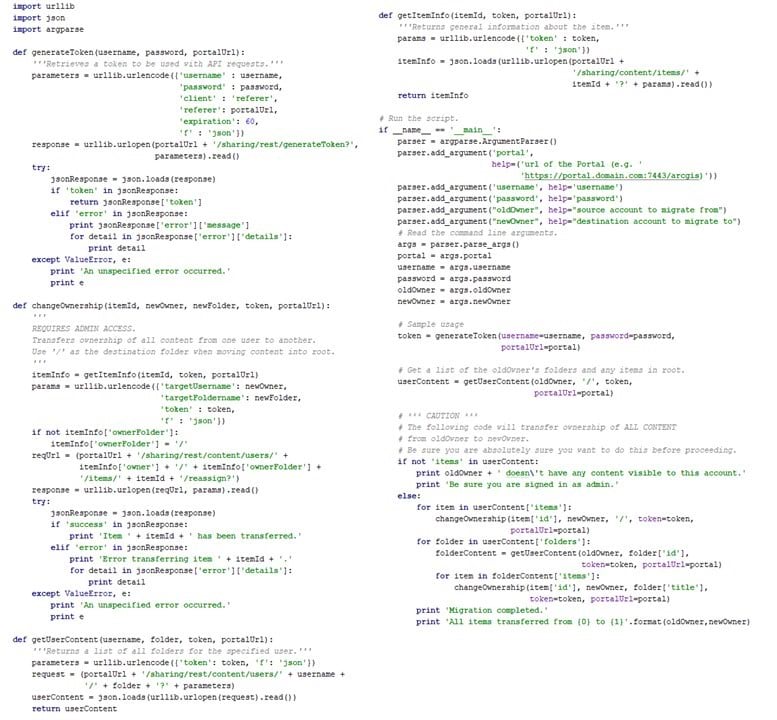
In contrast, to perform the same workflow using the ArcGIS API for Python, all you need is:
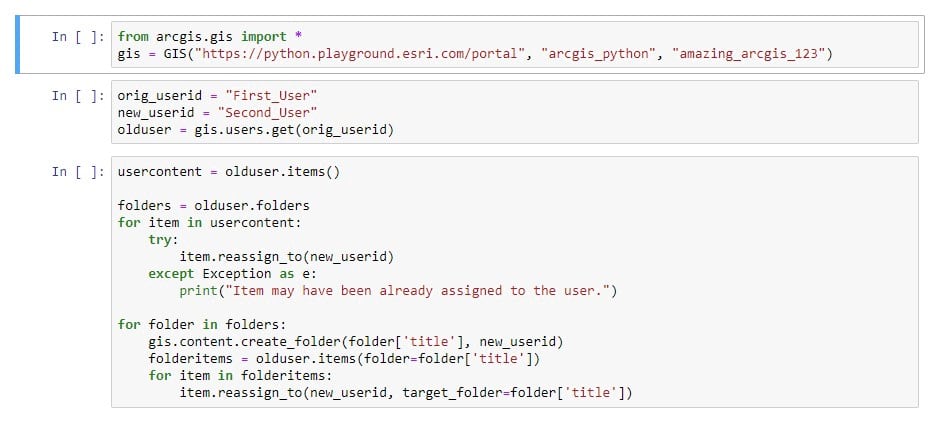
As the ArcGIS API for Python constructs URL requests, you can write or share a much simpler script than you would without it. In the above example, using the ArcGIS API for Python reduced the number code lines from 117 to 19 to complete the same task! Before you start using the ArcGIS API for Python, it is nice to have a good grasp on what the ArcGIS API for Python is and some best practices. This two-part blog breaks down all the components and concepts of using Python API, how they relate to each other, and best practices.
What is the ArcGIS API for Python?
The ArcGIS API for Python can be defined as: “A Python API developed by Esri to manage Web GIS via the ArcGIS REST API.”
But that definition assumes that you know what all the following are:
- Python
- API
- ArcGIS REST API
- Web GIS
If you are reading this blog, I assume you already understand Web GIS. Just to get everyone on the same page, though, Web GIS is a concept that contains any GIS data management system using the web to share, edit, or manage the data. At Esri, we use Web GIS to refer to both ArcGIS Online and ArcGIS Enterprise (particularly Portal for ArcGIS). We will now dive in to the other concepts and how they connect to allow you to manage your Web GIS.
Understanding Python Installation
Python is an open-source programming language that Esri, along with many other software platforms, uses to create processing tools. A Python installation comes with both a core code, for example a python.exe and associated files, plus a package of additional tools and functions. The user can add other packages to expand the functionality.
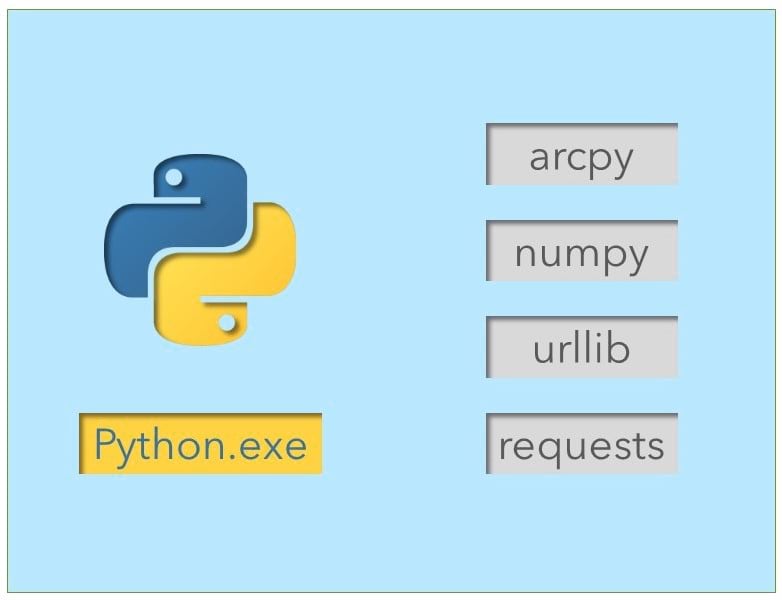
For example, the Python installation that comes with ArcGIS Desktop has the arcpy package, which contains all ArcGIS geoprocessing tools. Other common packages include numpy, which has mathematical functions, and urllib and requests, which allow you to construct and send URL requests through your Python script. Numpy is usually installed by default with Python, but the user must install urllib and requests.
ArcGIS Desktop comes with a Python install, but not all ArcGIS Python installs are the same. Between the development of ArcMap and ArcGIS Pro, Python came out with several new versions.
Because of this, an ArcMap installation will come with Python 2.7 and the arcpy package in ArcMap works with Python 2.7.
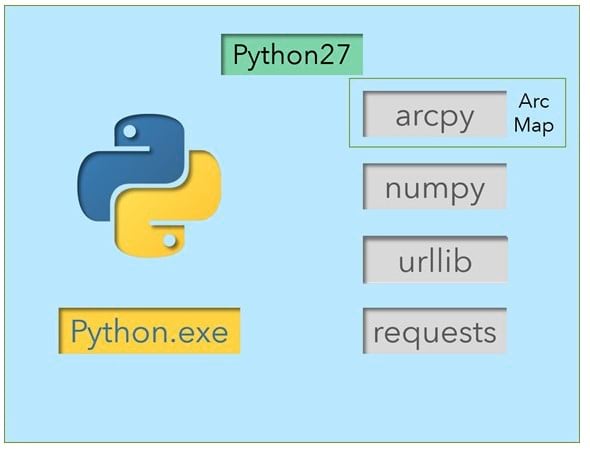
ArcGIS Pro, on the other hand, was developed using Python 3.5, and the ArcGIS Pro arcpy package requires a Python 3.5 install. Similarly, the ArcGIS API for Python can only be installed on a client using Python 3.5. The Python package of the ArcGIS API for Python is called arcgis.
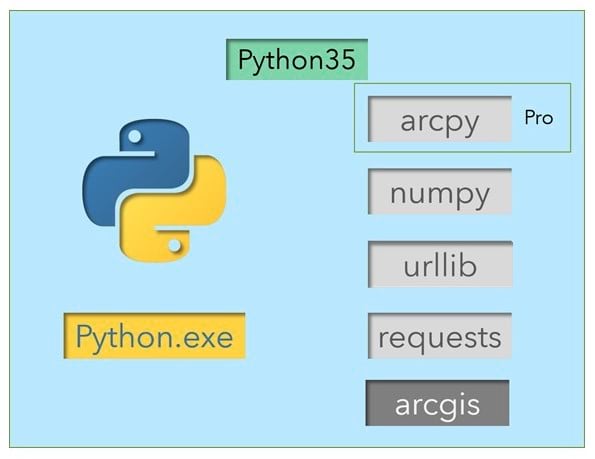
“But wait,” you may be thinking, “I thought the ArcGIS API for Python was an API. Why are you referring to it as a Python package?”
Good question!
The ArcGIS API for Python is both a Python package and an API.
Python packages are packages of tools that can be installed in a Python environment, and that is exactly what the ArcGIS API for Python is.
However, the ArcGIS API for Python is also an API. Which leads us to the next question: what is an API?
What is an API?
API stands for “Application Programming Interface” and is a library of tools that allows two applications to interact with each other. Examples include the Javascript API, Silverlight API, and ArcGIS REST API.
Imagine you have a colleague who wants a drink from a vending machine. In other words, we have an application (a thirsty colleague) that would like to make a request (buy a soda) from a server (the vending machine). To do this, there needs to be an interface that both the application and the server can understand and interact with. In this case, the keypad acts as the interface between the application and the server.
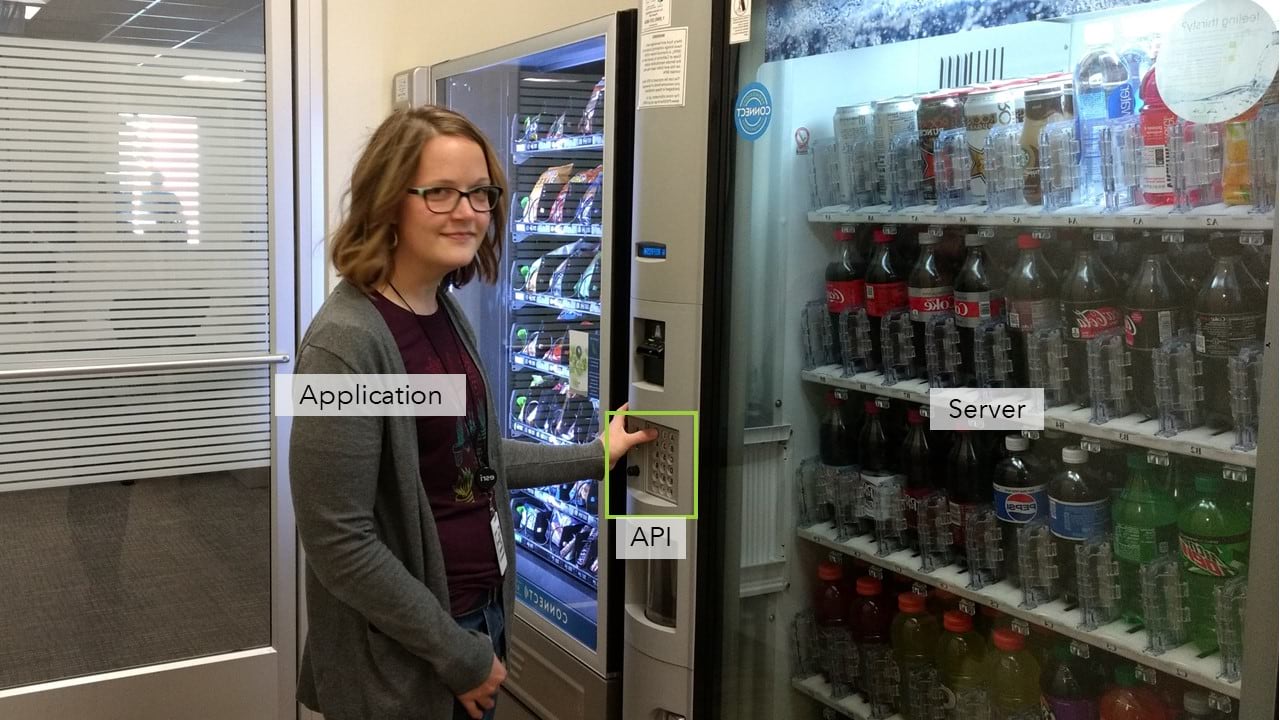
A real-world example of an API is the ArcGIS REST API, which is a set of tools that allows applications to make requests of ArcGIS Server sites. As the name implies, the ArcGIS REST API contains the tools to allow applications to make REST requests of the RESTful ArcGIS Server sites. Since the ArcGIS API for Python interacts with the ArcGIS REST API, let’s dig into what it means to say a server site is RESTful.
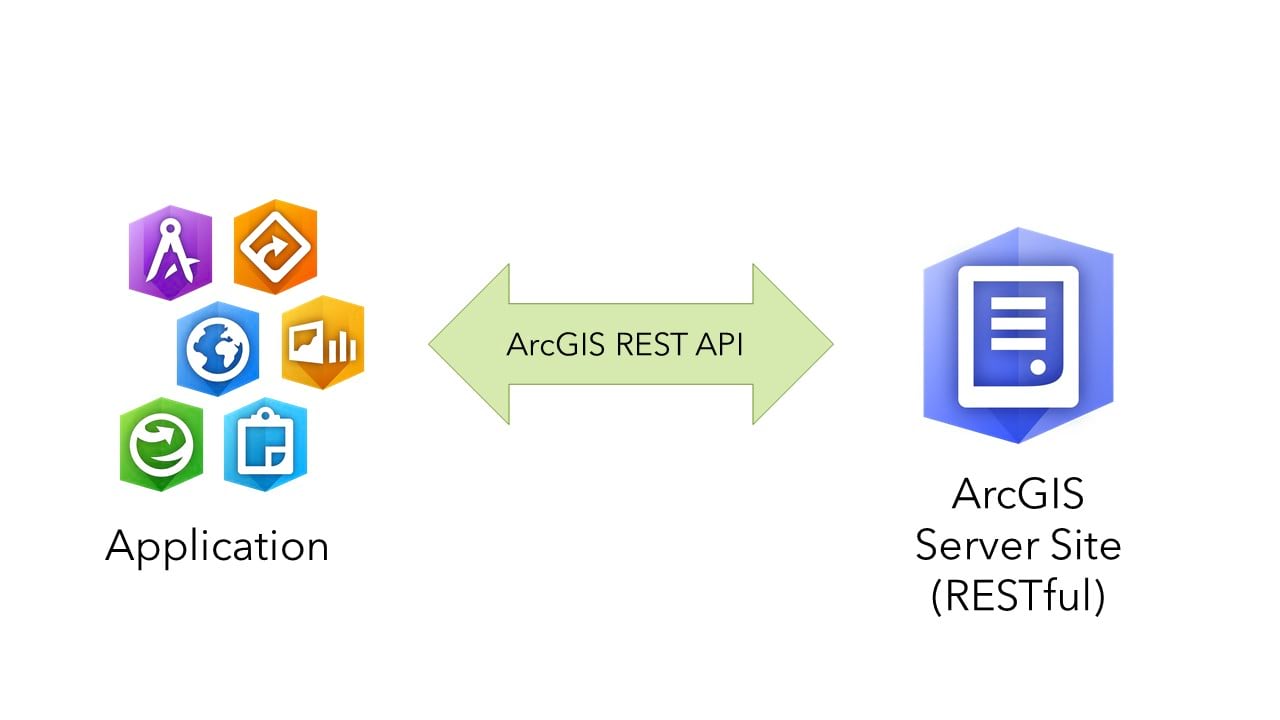
What is REST?
Representational State Transfer, or REST, is an architectural style that, among other functions, organizes a site in a way that allows people to read URLs. ArcGIS Server sites use the REST architectural style to create sites that can be navigated the same way you navigate through computers folders. Here we are in the services directory on the REST site of the SampleServer6 server: https://sampleserver6.arcgisonline.com/arcgis/rest/services
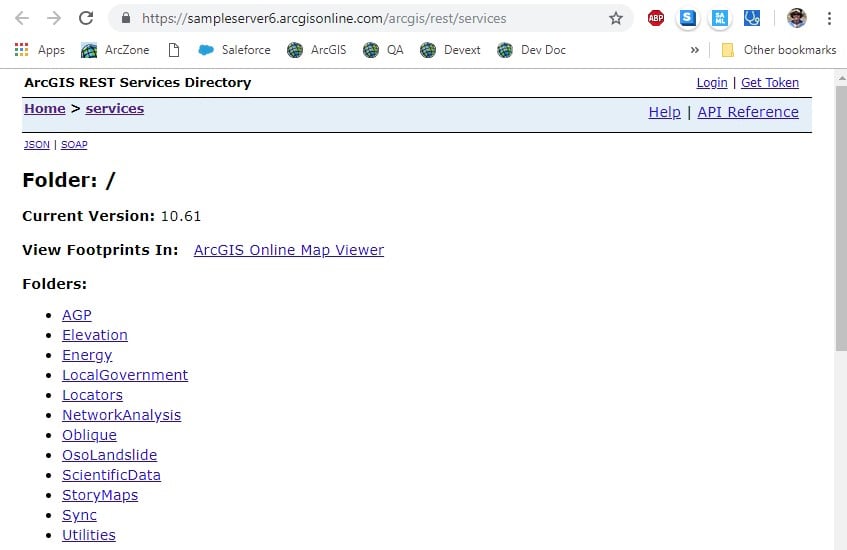
If I open the Energy folder on the Service Directory, “/energy” gets added to the URL just as it would in Windows Explorer (https://sampleserver6.arcgisonline.com/arcgis/rest/services/Energy). From there, I can open the Geology Feature service, which will get added to the URL as “Geology/FeatureServer.”
If I want to work with a specific service layer (for example, the Fault layer) the number of the layer (0) will be added: https://sampleserver6.arcgisonline.com/arcgis/rest/services/Energy/Geology/FeatureServer/0
Once the URL is pointing to a specific service or service layer, operations you want to perform on that service or layer can be appended to the end of the URL. For example, if you want to query a specific feature by its ObjectID from the Fault (0) layer of the Geology service, you could add the “query?” operation from the ArcGIS REST API and any parameters for the operation (for example, “objectIds=41568”). When you send the URL request, SampleServer6 responds to the request with information on the feature 41568 in the first layer of the Geology feature service. The URL request now looks like this:
The ArcGIS REST API, then, is a list of all the operations you (or an application) can perform on services in ArcGIS Server sites. These operations are then applied to the services using the REST architecture in the URL request.
The ArcGIS API for Python vs the ArcGIS REST API
If an API is the interface between two applications, and the ArcGIS REST API is the interface between an application and ArcGIS Server sites, what is the ArcGIS API for Python an interface between?
There are two ways to think about this:
You can think of the ArcGIS API for Python as the interface between a Python script and the ArcGIS REST API. The ArcGIS REST API then interfaces between the ArcGIS API for Python and the ArcGIS Server site.
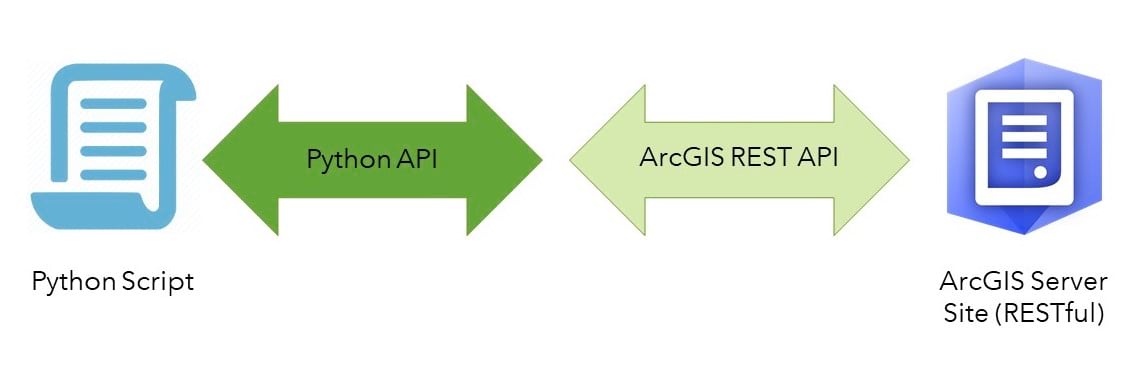
Alternatively, you can think of the ArcGIS API for Python as a pythonic wrapper around the ArcGIS Rest API, and both work together as the interface between the script and the ArcGIS Server site.
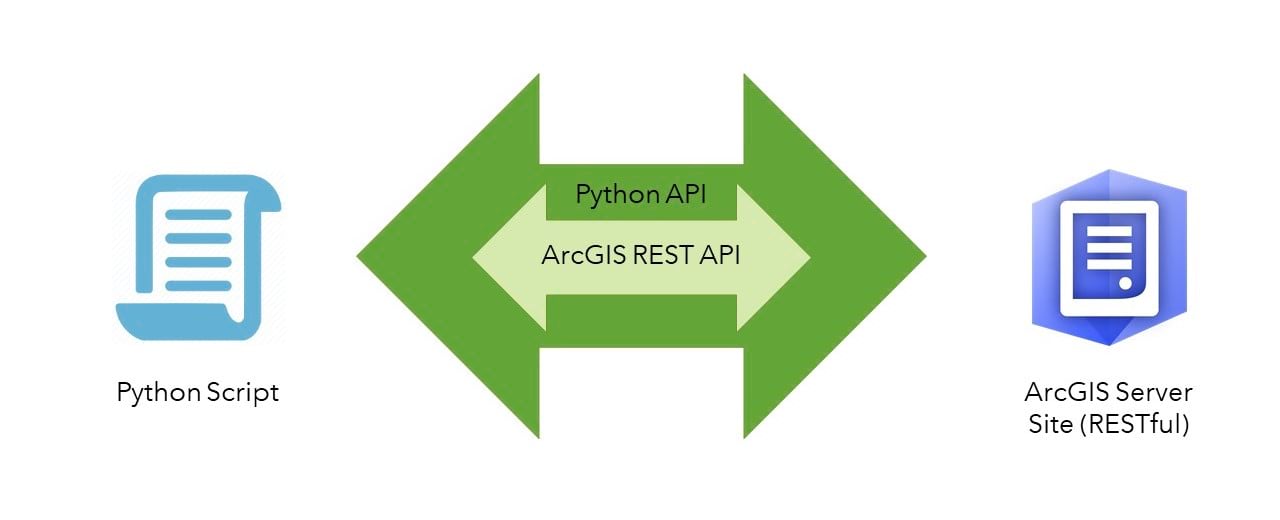
The ArcGIS API for Python wraps the construction of ArcGIS REST API URLs in pythonic functions, so instead of having to construct a URL manually in a script, you can call on pre-built functions that will construct the URLs in the backend.
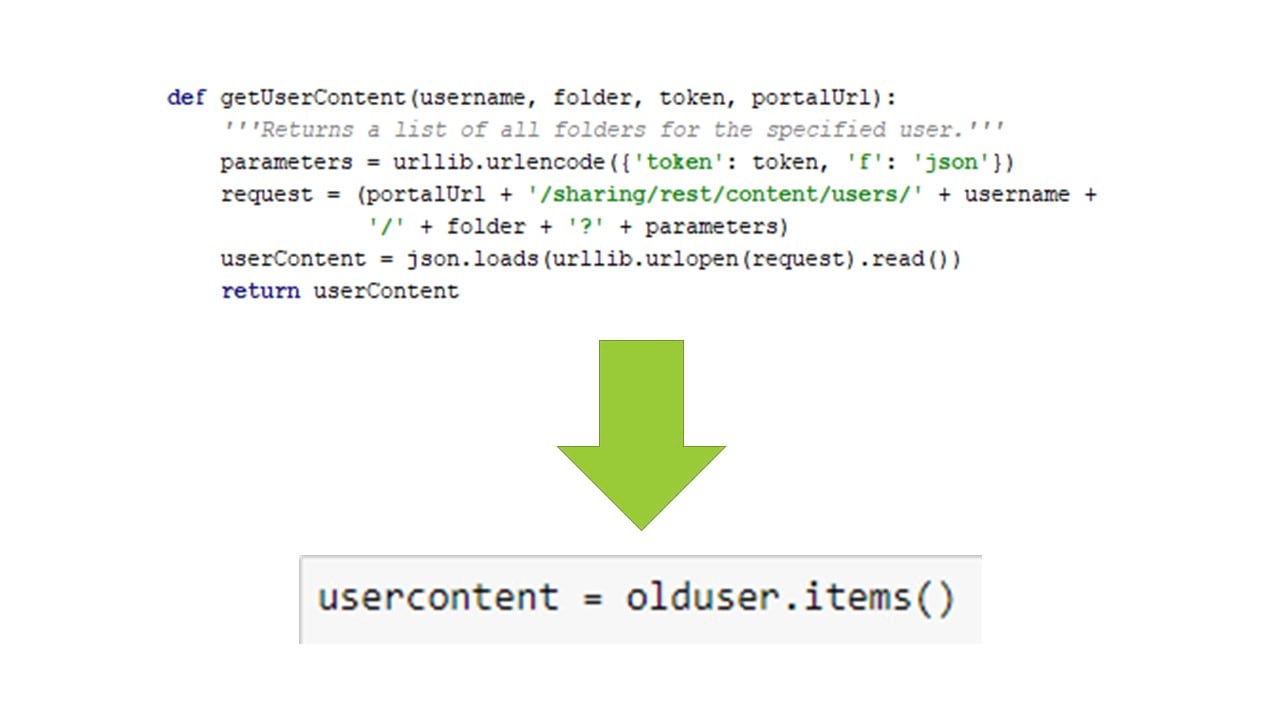
What is the ArcGIS API for Python?
Now that we have dissected Python, Python packages, APIs, and the ArcGIS REST API, we can look back at the original definition and dissect it a bit more to come up with a final definition:
The ArcGIS API for Python is both an API and a Python package. It includes tools that help a Python script use the ArcGIS REST API, which in turn includes the tools to make requests of ArcGIS Server services. Therefore, the ArcGIS API for Python helps you write Python scripts to manage your content and users in your Web GIS.
Want to learn more? Check out the second part of this blog.
Article Discussion: