You can search for your assets in ArcGIS Online and ArcGIS Enterprise, and those searches are honored in Collector, Explorer, and other apps. To configure these searches, use ArcGIS Online or ArcGIS Enterprise and configure the settings for your map. But what if you want the same search to be used by a set of maps with the same layer, or want to make other search changes across multiple maps without having to make the update for each map individually?
Use Python to automate configuring search. You can configure various components, including the search hint text, as well as what layers and fields are searched. Let’s look at how.
Search a feature layer
Supporting feature search allows your users to find the assets they need to work with. For example, they might want to search hydrants by their asset IDs.
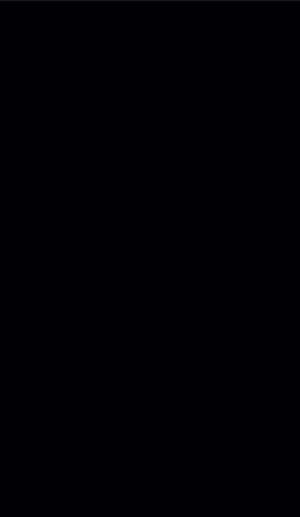
For an individual map, this can be configured in the web map settings.
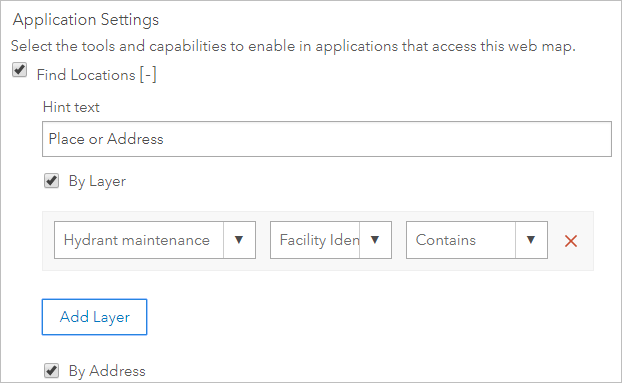
You can also add feature search using Python. You might be adding feature search to the map, adding it to an additional layer, or even adding it to an additional field in a layer that’s already searchable. No matter which scenario applies to you, you can accomplish it using Python.
In the function below, you pass in a WebMap
, the URL for the layer you want to search, the name of the field to search, if it should be an exact match, and the type of field.
add_layer_search(webmap_item, layer_url, 'Facility Identifier', True, 'esriFieldTypeInteger')
This function updates the map so that the layer is searchable.
Expand to see `add_layer_search` function code
def add_layer_search(webmap_item, layer_url, field_name, exact_match, esri_field_type): json_data = webmap_item.get_data() if 'applicationProperties' not in json_data: print("Bad JSON -- OOPS!") return app_properties_viewing = json_data['applicationProperties']['viewing'] # Get the ID of the layer to search, based on the URL you know it has webmap = WebMap(webmap_item) layers = webmap.layers layer_id = None for layer in layers: if layer.url == layer_url: layer_id = layer.id if layer_id is None: print('Layer URL not found: ' + layer_url, end='\n\n') return # if there isn't feature search defined, add the property with the defaults so that the next section can add the layer info if 'search' not in app_properties_viewing: search_property = {'enabled': True, 'disablePlaceFinder': False, 'hintText': 'Place or Address', 'layers': [{'id': layer_id, 'field': {'name': field_name, 'exactMatch': exact_match, 'type': esri_field_type}}]} app_properties_viewing['search'] = search_property webmap_item.update(data = json.dumps(json_data)) # else if there is already a feature search defined, add a layer to that search elif 'search' in app_properties_viewing: search_layers_property = app_properties_viewing['search']['layers'] new_layer = {'id': layer_id, 'field': {'name': field_name, 'exactMatch': exact_match, 'type': esri_field_type}} search_layers_property.append(new_layer) webmap_item.update(data = json.dumps(json_data))
Provide hint text
Use the hint text for your search to let users know what they can search for to find your assets. For example, if you added the hydrant ID, include that in the search hint.
For an individual map, this can be configured in the web map settings.
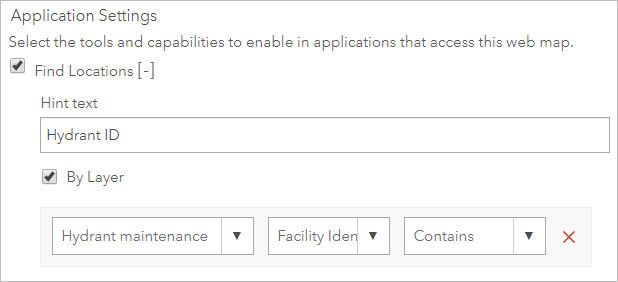
If you want to customize the hint text for multiple maps, you can configure it using Python. In this code, you provide the new hint text and the map is updated with it.
Expand to see the code to check if a layer has search configured, and if so, provide hint text
webmap_item = webmap_item_with_search json_data = webmap_item.get_data() app_properties_viewing = json_data['applicationProperties']['viewing'] if ('search' in app_properties_viewing): layer_search_property = app_properties_viewing['search'] hint_text = {'hintText': 'My search-specific hint text'} layer_search_property.update(hint_text) webmap_item.update(data = json.dumps(json_data))
Disable place search
What if you want your mobile workers to always find assets by the IDs for which you’ve enabled searching, and you don’t want them searching for generic places or addresses? You can disable place search in the map’s settings:
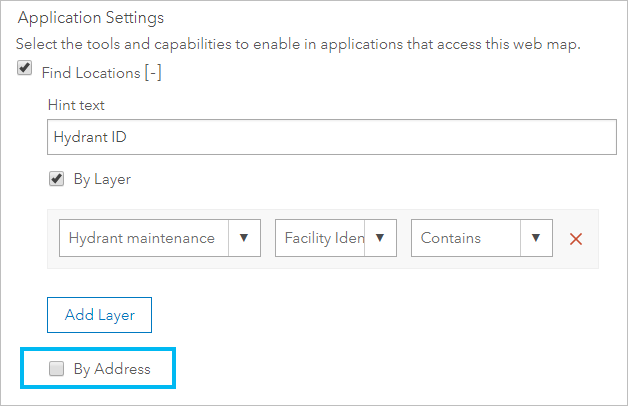
As with the other search configurations above, you can also do so using Python. Here, the disablePlaceFinder
property is set to True
:
Expand to see the code to disable place search
webmap_item = webmap_item_with_search json_data = webmap_item.get_data() app_properties_viewing = json_data['applicationProperties']['viewing'] if ('search' in app_properties_viewing): layer_search_property = app_properties_viewing['search'] disable_place_finder = {'disablePlaceFinder': True} layer_search_property.update(disable_place_finder) webmap_item.update(data = json.dumps(json_data))
Next steps
Now that you can automate the search settings of your maps, think about the maps you have and where searching could be useful. If there are multiple maps that need similar updates, you can update them all at once instead of having to configure each map’s search.
Commenting is not enabled for this article.