Introduction
Getting Started
ArcGIS Runtime makes getting started with 3D very simple. Conceptually, there are two basic components, which are the SceneView (the visual component), and the Scene (the data component). Let’s take a deeper look at these components, how they relate to each other, and how you can use them to create a 3D application.
SceneView
To visualize your data in 3D you need to start with a globe, which is implemented via the ArcGIS Runtime SceneView. You can change many of the visual aspects of the SceneView, such as the atmospheric effects, sun position, light characteristics, and so on.
There are two different atmospheric effects, realistic and horizon. You can see usage of the realistic atmospheric effect and how to apply sun lighting in the code snippets later in the topic.
A SceneView is used to display a Scene which contains the 3D data.
Scene
Once you have the SceneView, you need to configure your data by creating a Scene with a basemap (using the basemapStyle API), add a base surface, and one or more operational layers. The basemap provides context for your data (background in other words), the BaseSurface provides the terrain (elevation), and the operational layers represent your interactive data and other relevant information needed by the application. Let’s take a quick look at some of the basic components of the Scene.
Basemap
In ArcGIS Runtime, you can construct a scene using a basemap. There are several choices for Esri provided basemaps that you can use out of the box and you can choose from a collection of raster or vector basemaps. If none of those basemaps fit your needs, you can also construct a basemap using your own data.
BaseSurface
This is where terrain, which is an integral part of a 3D application, is added to the scene. Terrain is defined by a collection of elevation sources, which can be tiled elevation sources (online or offline), raster elevation sources or a combination of both. The code snippet below adds a tiled elevation source via a URL.
OperationalLayers
Operational layers are the interactive data component of the scene. You can do various operations on them such as identify, selection, apply renderers and so on. You will learn a lot more about them in the subsequent articles in the series.
With all the three data components, the scene is ready to be added to the SceneView for visualization. The code snippet below demonstrates how to construct them.
// Create a scene using the ArcGISImagery BasemapStyle
Scene* scene = new Scene(BasemapStyle::ArcGISImagery, this);// create a new elevation source from Terrain3D rest service
ArcGISTiledElevationSource* elevationSource = new ArcGISTiledElevationSource(
QUrl("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer"), this);// add the elevation source to the scene to display elevation
scene->baseSurface()->elevationSources()->append(elevationSource);// Create a scene view, and pass in the scene
m_sceneView = new SceneGraphicsView(scene, this);// Apply an atmospheric effect to the sceneview
m_sceneView->setAtmosphereEffect(AtmosphereEffect::Realistic);// add an operational layer
ArcGISSceneLayer* sceneLayer = new ArcGISSceneLayer(QUrl(QDir::homePath() + "/ArcGIS/slpks/sanfran_v17.slpk"), this);
scene->operationalLayers()->append(sceneLayer);
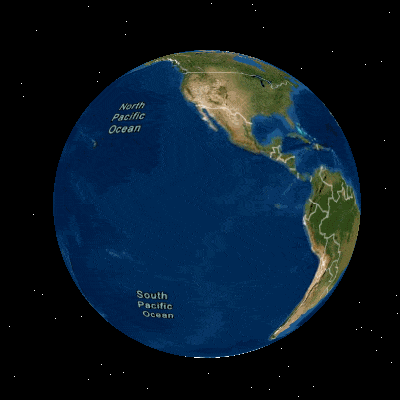
SceneView with no atmospheric effect.
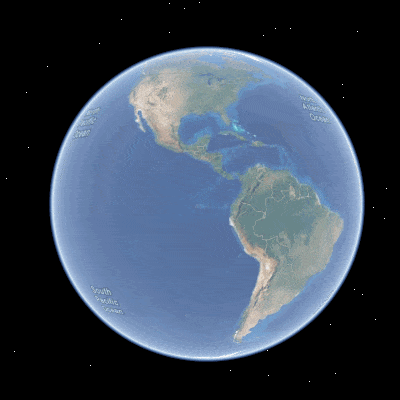
SceneView with realistic atmospheric effect.
Navigating within the SceneView
ArcGIS Runtime provides various mechanisms for navigation within the SceneView. You can use any of the CameraControllers for specific ways to navigate. Let’s take a look at the controllers and see how they work.
Navigation using Camera Controllers
Runtime provides multiple camera controllers out of the box Camera controllers can be a great way to control the navigation experience based on a specific workflow. The default camera controller is the GlobeCameraController. The globe camera controller can be used to move around freely without any constraint within the sceneview.
If you need to lock the camera to a (moving or stationary) object in the scene, and follow it if it moves, OrbitGeoElementCameraController is an excellent choice. The camera in this case will always focus on the object.
If you need to lock the camera to a certain location, then OrbitLocationCameraController is ideal. You can create beautiful animations using methods on the camera controllers. Below is an example of how an OrbitLocationCameraController can be used to spin around a location for a specified period of time.
// create a new controller using the location from the screenToLocation callback and the camera's current location
m_controller = new OrbitLocationCameraController(location, m_sceneView->currentViewpointCamera().location(), this);// set the camera controller
m_sceneView->setCameraController(m_controller);// move the camera with just a heading offset and run it for 10 secs
m_controller->moveCamera(0, 360, 0, 10);
});// call screen to location to get the center of the sceneview
m_sceneView->screenToLocation(m_sceneView->width() * 0.5, m_sceneView->height() * 0.5);
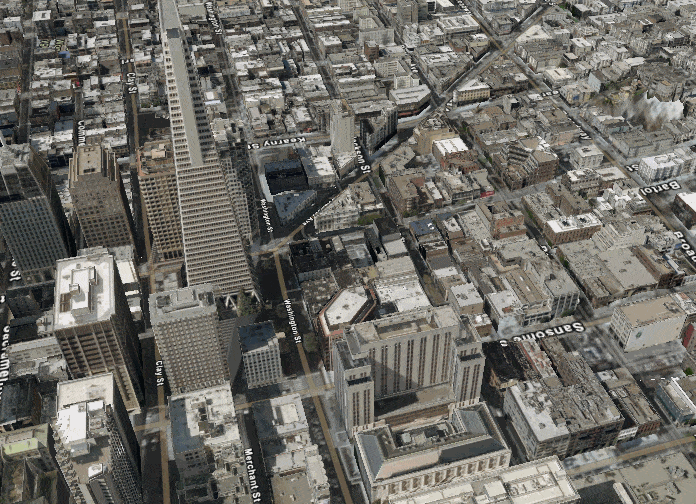
In the code above, the OrbitLocationCameraController is being created on the click on a button. The video above shows the result of that operation.
Subsurface Navigation
When working in 3D, not all data is above the ground. You may also need to view and navigate around data below ground. Imagine a city employee trying to look at its utility system that’s below the ground or a designer trying to visualize the part of a building that’s under the ground like an underground parking structure. This can be easily achieved by changing the navigationContraint property on the scene’s baseSurface. By default, the scene restricts navigation to stay above the ground. So, when there is a need to go below ground, this constraint can be removed by setting it to none. The code below demonstrates how to do this.
// change the constraint to none to enable subsurface navigation
scene->baseSurface()->setNavigationConstraint(NavigationConstraint::None);
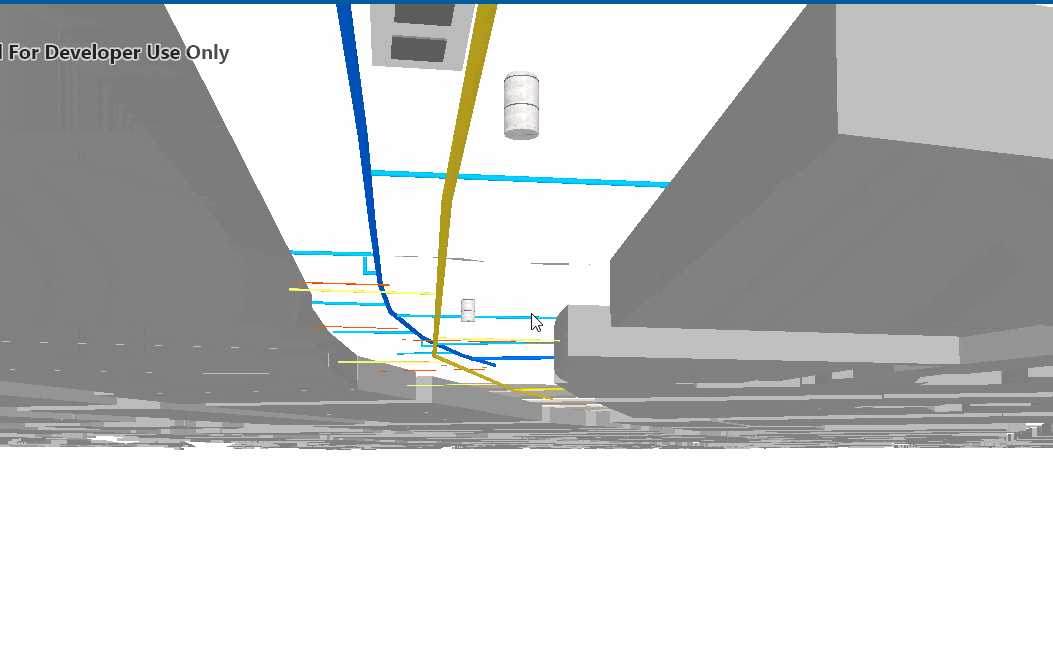
Scene with 100% opaque basesurface
In addition to the above, if you need to look at objects beneath the surface, while staying above ground, you can easily achieve that by increasing the basesurface transparency as illustrated with the following snippet.
// set a desired opacity value for the basesurface
m_scene->baseSurface()->setOpacity(0.75);
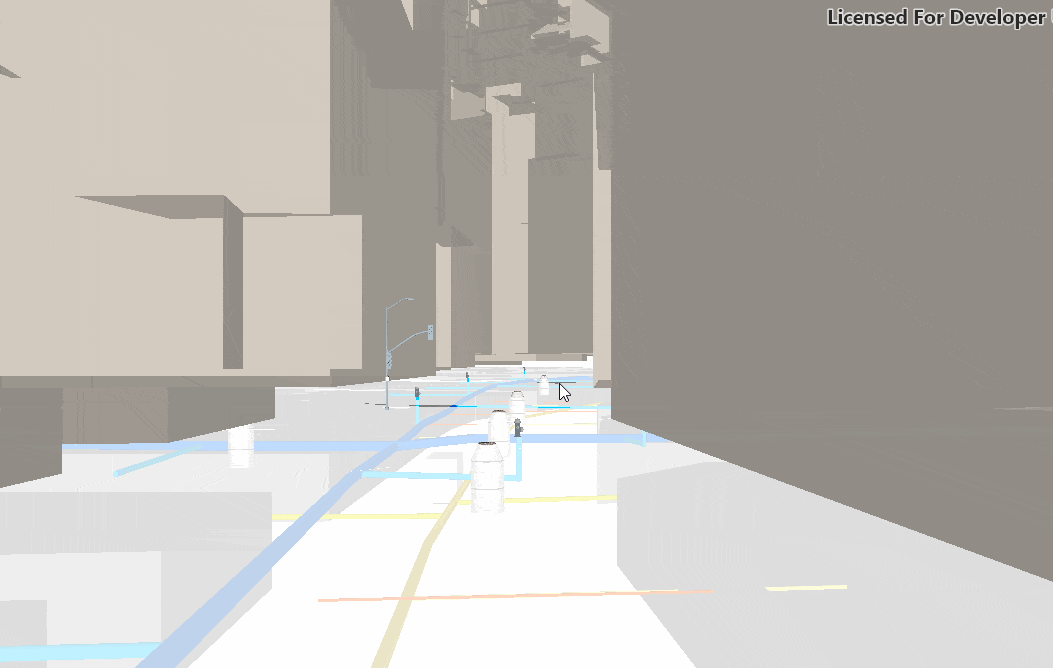
Scene with 75% opaque basesurface
Visual Effects
Earlier, you saw how to configure a scene that can be added to the SceneView. Let’s take a look now at one of the effects that can be applied to the SceneView, which is sun lighting.
Sun lighting effect
Sunlighting can be controlled in the SceneView with just a couple of lines of code. Sun lighting can be used to light up your objects in the scene using the sun as the lighting source. You can choose between three options.
- You can enable lighting only in which case objects in the scene are illuminated or darkened based on the time of the day, but don’t cast shadows
- Choose lighting and shadows where lighting is applied and the objects in the scene (such as buildings) also cast shadows. The shadows from sun lighting adds more context to your data and thus makes them visually richer.
- No lighting (which is the default) where there is no illumination or shadows.
Shadows can be visualized in real time by setting the suntime, which casts shadows based on the local time and date. The following snippet illustrates applying sun lighting and shadows to the SceneView.
// set the lighting mode to lights and shadows
m_sceneView->setSunLighting(LightingMode::LightAndShadows);// set the datetime based on your system clock for the shadows
m_sceneView->setSunTime(QDateTime::currentDateTime());
This will create sun lighting with the local date and time in the current time zone. The images below show the effects with sunlighting and shadows enabled, and sun lighting only with no shadows enabled.
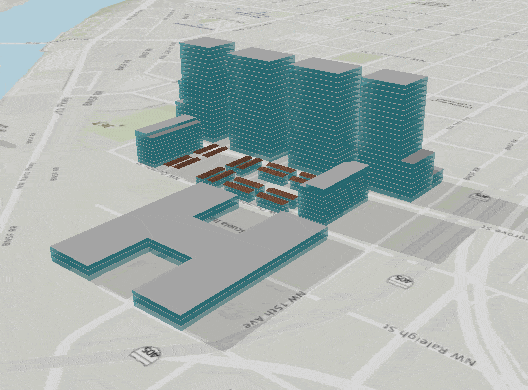
SceneView with lighting and shadows enabled.
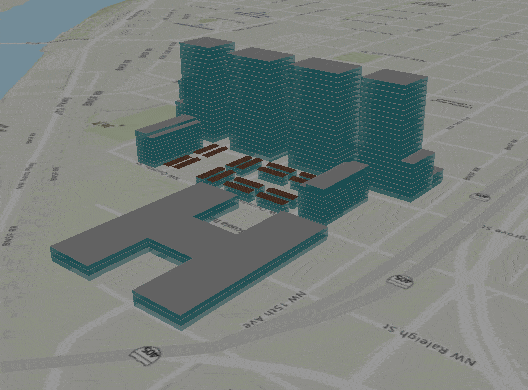
SceneView with only lighting enabled but no shadows
Summary
In this article, you’ve seen how to get started with 3D in ArcGIS Runtime. You also explored some of the components available when creating a 3D application along with some of the navigation controls and effects that pertain to 3D visualization. In the next article, you will learn various ways you can animate your scene using the camera, the available 3D data types, and how to add them as operational layers and work with them in your application.
We hope you enjoyed this article and look forward to hearing your thoughts and feedback. We can’t wait for you to start building 3D applications.
Article Discussion: