This is the third post in a four-part series covering the basic topics related to migrating your app built with the Google Maps JavaScript API to the ArcGIS API for JavaScript (JavaScript API). The series covers the following topics:
- Getting Started: Displaying a marker with a popup
- Getting directions and displaying a route
- Searching and Geocoding
- Adding a shape
In this post, we’ll do an overview on searching and geocoding.
Getting started
To get started with ArcGIS, sign up for the ArcGIS Developer Program at no cost. This account will get you access to the JavaScript API, 1,000,000 basemaps and geosearch (e.g. interactive find and zoom) transactions per month, access to a wide selection of rich content and services hosted in ArcGIS Online, unlimited non-revenue generating apps deployed, and quite a bit more for free. More information can be found in the first blog of this series.
Create a basic mapping app
Check out the Getting Started blog for an overview on how to load the JavaScript API and create a map.
Searching for places
There are multiple ways in which you can locate a place of interest on a map. One way of doing that is searching for a list of places nearby a particular location, based on a keyword or type.
Say you’d like to search for restaurant place names around a given location. With Google, you would define the search center, and then use the PlacesService to do a nearbySearch. In the callback you would parse and display the search results.
const searchCenter = {lat: 40.0150, lng: -105.2705};
const map = new google.maps.Map(document.getElementById("map"), {
zoom: 7,
center: searchCenter
});
const service = new google.maps.places.PlacesService(map);
service.nearbySearch({
location: searchCenter,
radius: 500,
type: ["restaurant"]
}, callback);
To do a similar search with the ArcGIS API for JavaScript, you define the search center point, and then use a Locator to search around that location using addressToLocations. The locator will use the ArcGIS World Geocoding Service and search for locations that are in the “food” category.
require([
"esri/Map",
"esri/views/MapView",
"esri/tasks/Locator",
"esri/geometry/Point"
], function(
Map, MapView, Locator, Point) {
const searchCenter = new Point({
type: "point",
latitude: 40.0150,
longitude: -105.2705
});
const map = new Map({
basemap: "streets-vector"
});
const view = new MapView({
container: "viewDiv",
map: map,
center: searchCenter,
zoom: 7
});
const locator = new Locator({
url: "https://geocode.arcgis.com/arcgis/rest/services/World/GeocodeServer"
});
locator.addressToLocations({
location: searchCenter,
distance: 500,
categories: ["Food"]
})
.then(function(results) {
// Parse results and draw graphics
})
});
If you’d like to try out a step-by-step tutorial on finding places, check out this DevLab.
With ArcGIS API for JavaScript you can also integrate search using the out of box Search widget. With just a few lines of code the widget provides an experience for searching and geocoding. In addition to doing a place search or geocode, you can configure it to also search within your own data on the map.
const searchWidget = new Search({
view: view
});
Here’s an example for adding the Search Widget in the top-right corner of an application:
view.ui.add(searchWidget, {
position: "top-right"
});
When a result is available the widget zooms the map to the associated location and automatically creates a popup. Here’s a sample for using the Search widget.
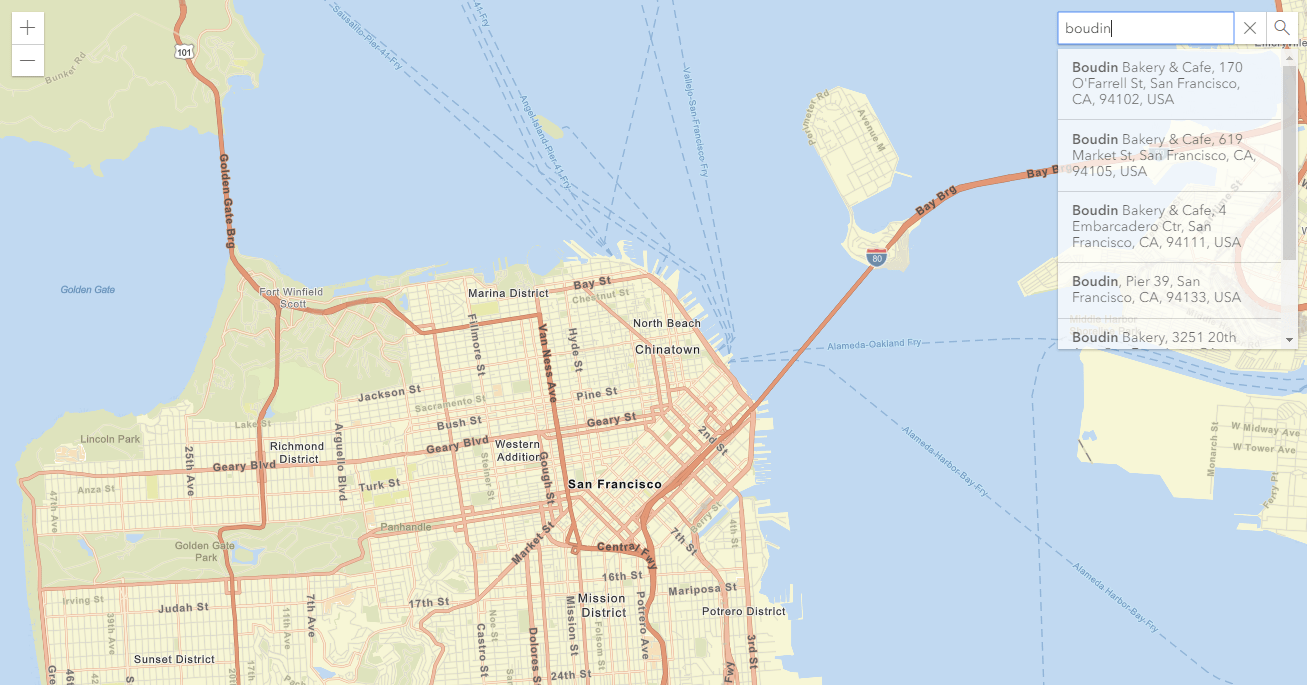
The Locator can be used in several ways in addition to searching for place names, for example geocoding and reverse geocoding. To search for an address by a specific point on the map use locationToAddress. This is done by listening for a click event that gives you the point where you clicked on the map. You can use this point with locationToAddress to return the best address for this location.
view.on("click", function(event){
geocoder.locationToAddress(event.mapPoint)
.then(function(response) {
// Parse results and draw graphics
});
});
If you’d like to try out a step-by-step tutorial on searching for an address using reverse geolocation, check out this DevLab.
Next Steps & more resources
Stay tuned for the next tutorial that will cover adding a shape to the map.
There are a variety of resources for learning about the ArcGIS API for JavaScript and maximizing your productivity when building your web apps:
- Learn more about what you can do with Locator, for example processing multiple addresses in a request, convert a location to an address, and the suggest API which provides character by character autocomplete suggestions.
- Explore hundreds of samples in a live sandbox for playing around with the code, a guide focused on key topics about developing with the API, and the API reference.
- Try out some ArcGIS API for JavaScript DevLabs, which are step by step tutorials for learning how to develop with the API.
Commenting is not enabled for this article.