React is a popular library for building web applications. However, it is only a library, not a complete framework. This is where something like NextJS becomes useful. NextJS is a complete React framework for building applications. It comes with a variety of features including routing, static site generation, and even built in API endpoints, so you can write server-side code in your application if you need it. It pairs great with the ArcGIS API for JavaScript.
You can get started with NextJS with the following command.
npx create-next-app@latest
For this application, we’re going to be looking at a layer of global power plants. For the user experience, we want to display a list of the types of power plants, and when the user clicks on a type of plant from a list, it will display a map of the power plants of the selected type.
You can find the source code for the application in this blog post on github.
API Route
To accomplish the first task of getting a list of the types of power plants, we can write an API route in a NodeJS environment. We can use the ArcGIS API for JavaScript in the route API to query the layer and extract the values from the results.
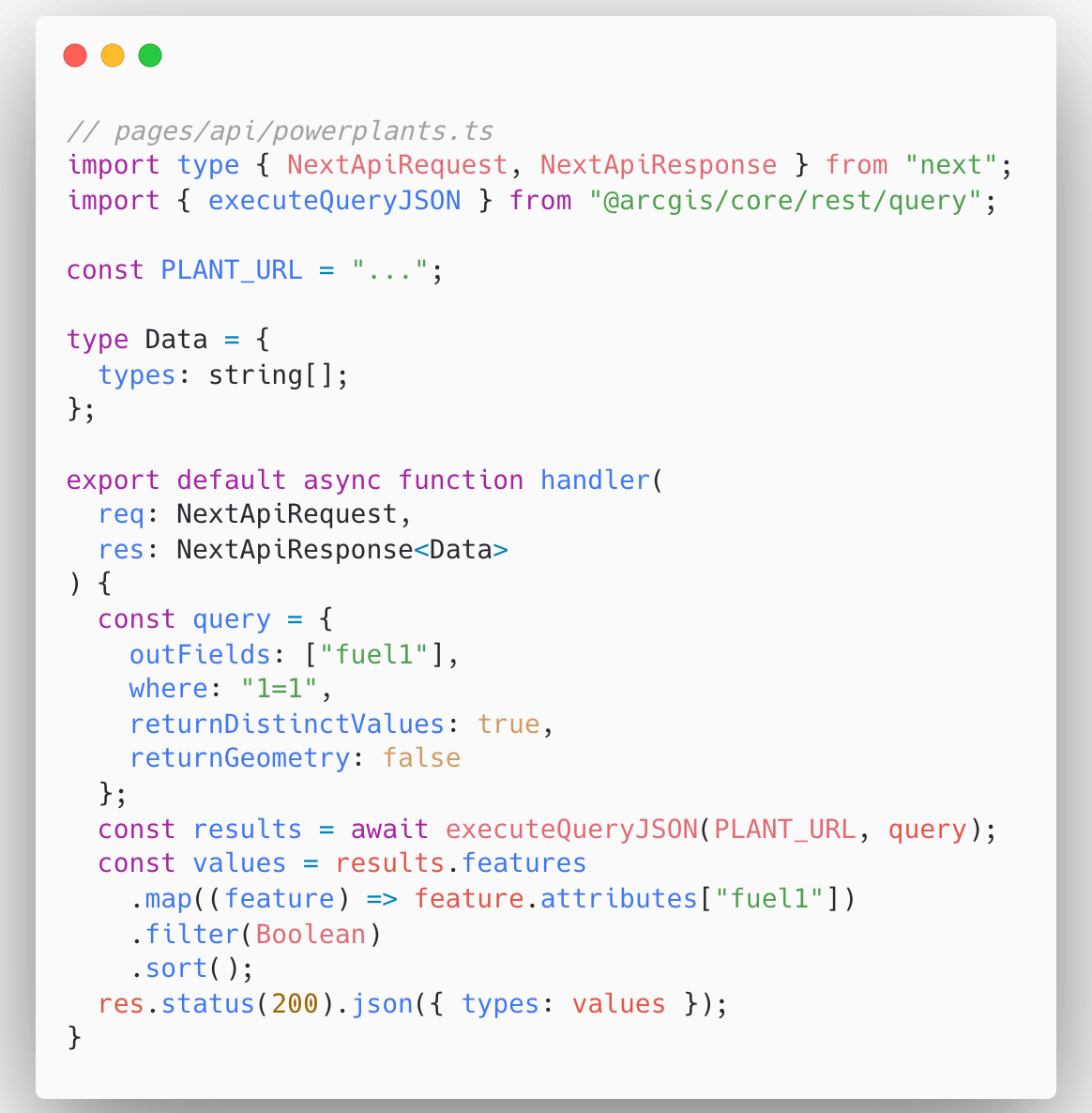
In this API route, we are going to query the feature service, limit the results only to the field for the primary type of power generated at the plant and extract that to a simple list. The best part of this is that this query is executed on the server, so there is no latency on the client to run this query.
Redux and Stores
To manage the application state, we can use Redux. If you’ve used Redux in the past, you might be thinking you need to set up a lot of boiler plate code for constants, actions, and reducers. The Redux toolkit helps to simplify this using slices with the createSlice() method. This will let you define the name of the slice, the initial state, and the reducers, or methods used to update the state. We can create one that will be used for our application.
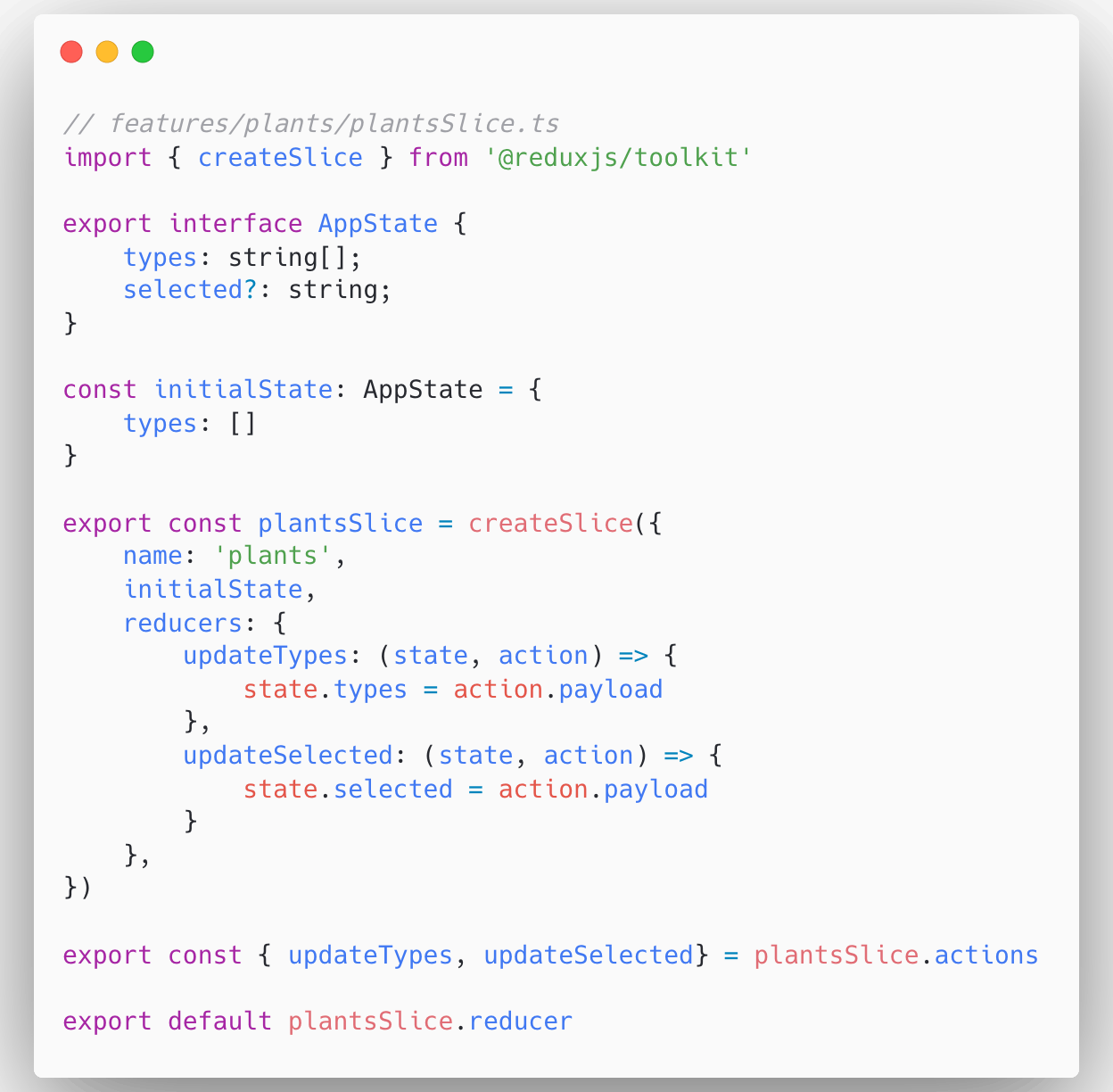
With our slice and reducers defined, we can create a React store and hook to be used in our application for the reducer.
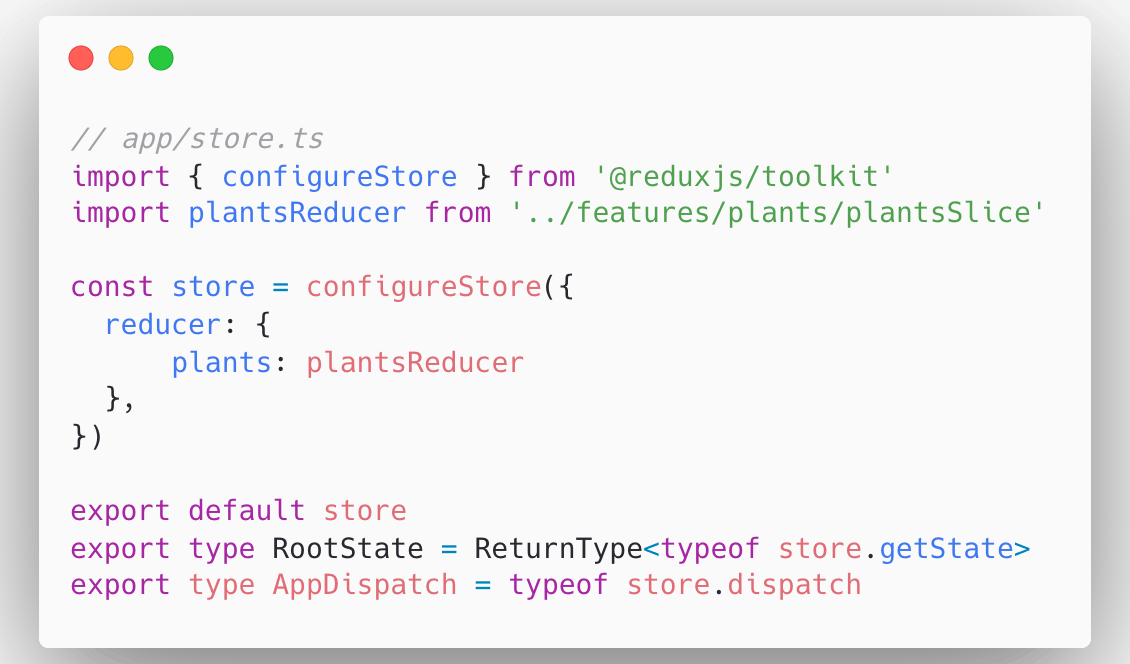
In this case, the only reason we really need the custom hooks is to have proper TypeScript typings.
Layout
At this point, we can start thinking about how the application and pages will be displayed. We can start with a layout file.
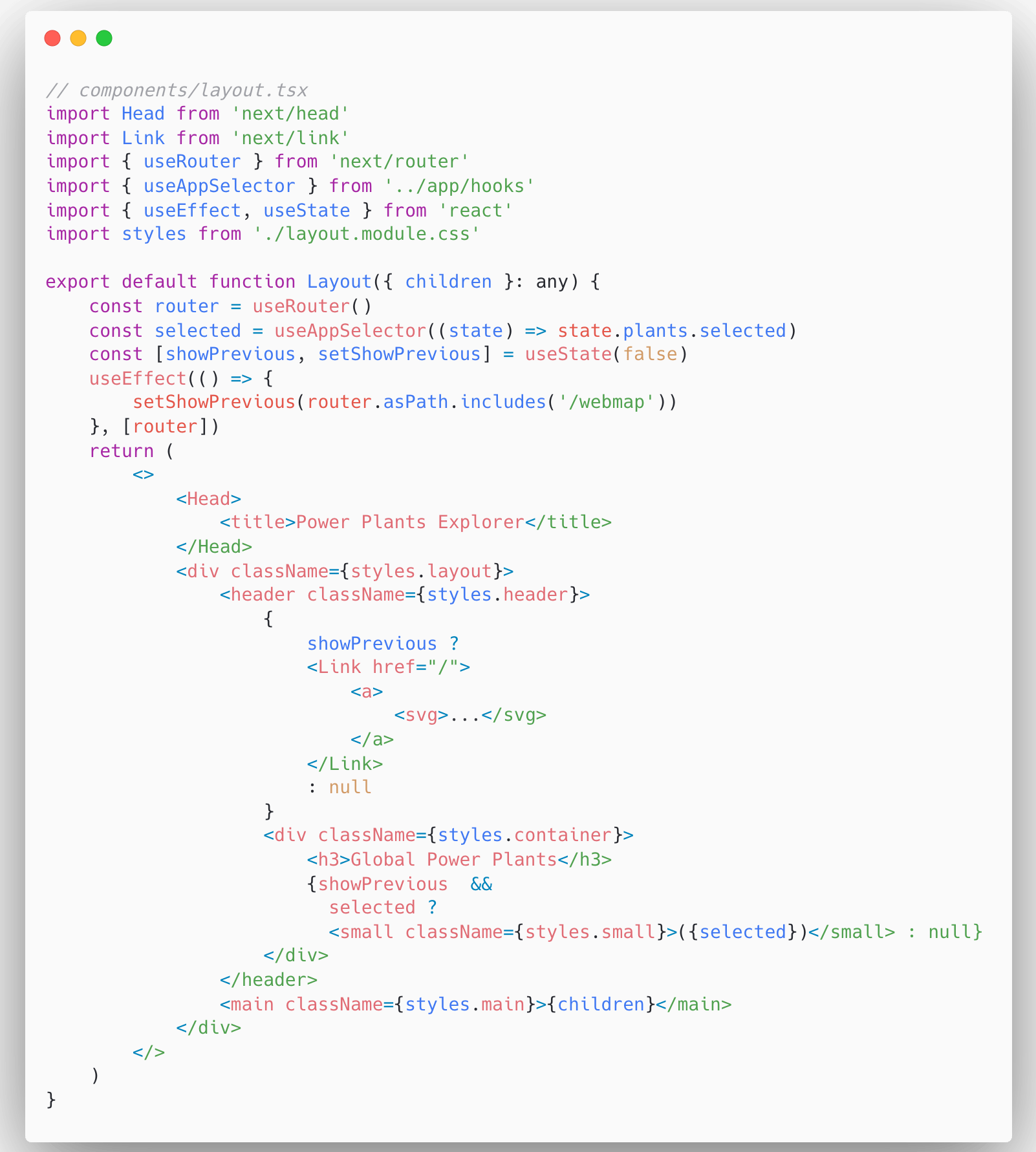
The layout is going to define how the all pages are going to look. We are going to have a header on the page with a navigation button and a title. This will be visible on all the pages of our application. Then we can define a section of the layout that will be used for the varying content.
Router
This is also where we start looking at the provided router with NextJS. When we are on the page that displays the map, we want to add a back button to return to the list of power plants. The layout page creates a header, and a main element for the content.
We can use the layout in the global App for NextJS.
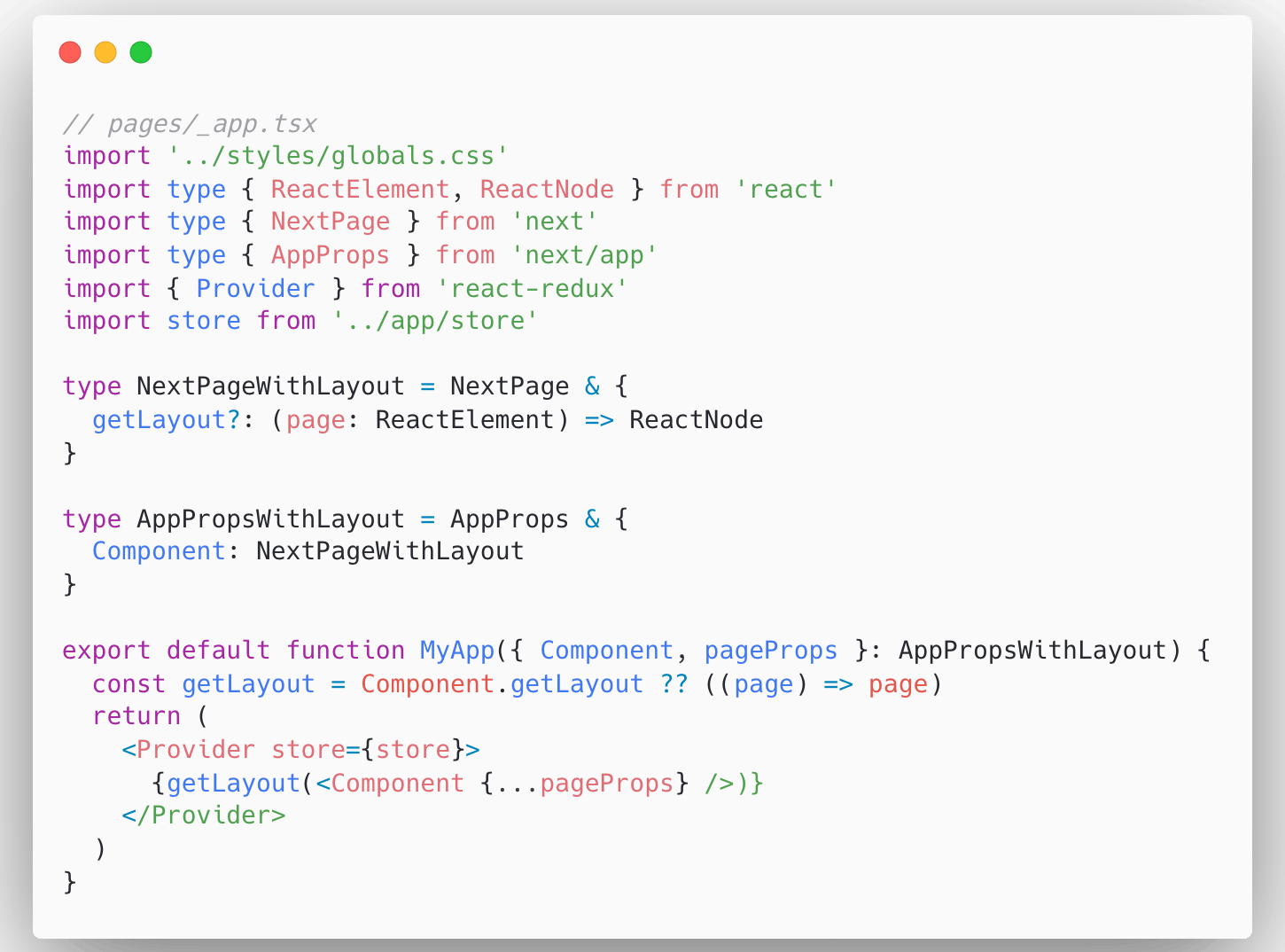
It’s in this global app file that we can add the layout and provider for our Redux store. The global app will determine if there is a layout or not and apply it.
API
To fetch data from our routing API, we can use swr, which will provide a React hook that handles fetching data for us. It’s not required, but it’s a useful tool to help wrap a number data fetching capabilities, like caching and more.
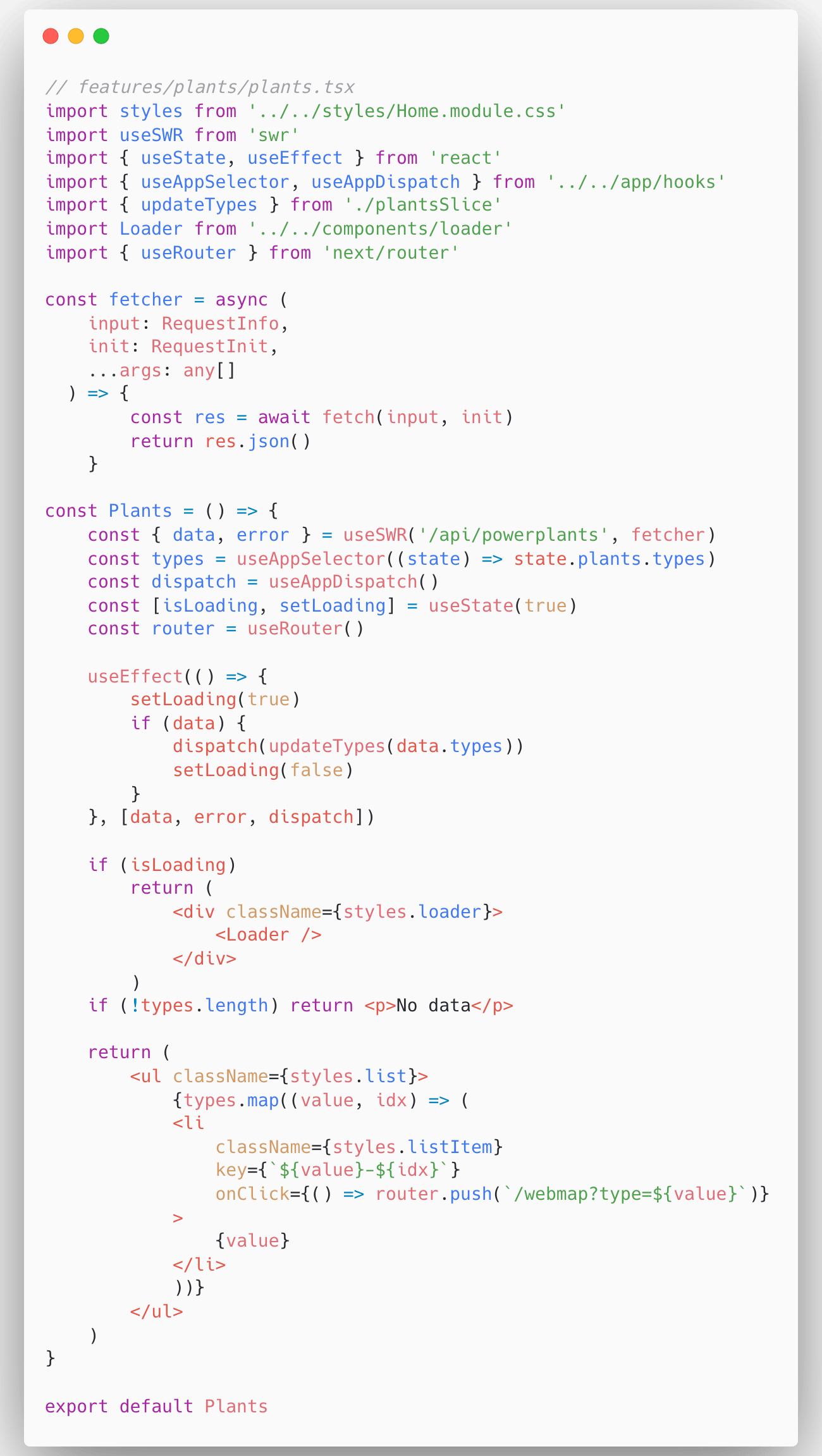
Pages
The plants component will fetch the list of power plants and display them. It will display a simple animated SVG loader while it loads the request. When a type of power plant is selected from the list, it will route to a page that displays the map and will filter the results to the selected type of power plant. Since the entry page for this application will display the list of power plants, we can use this Plants component in our index.tsx file.
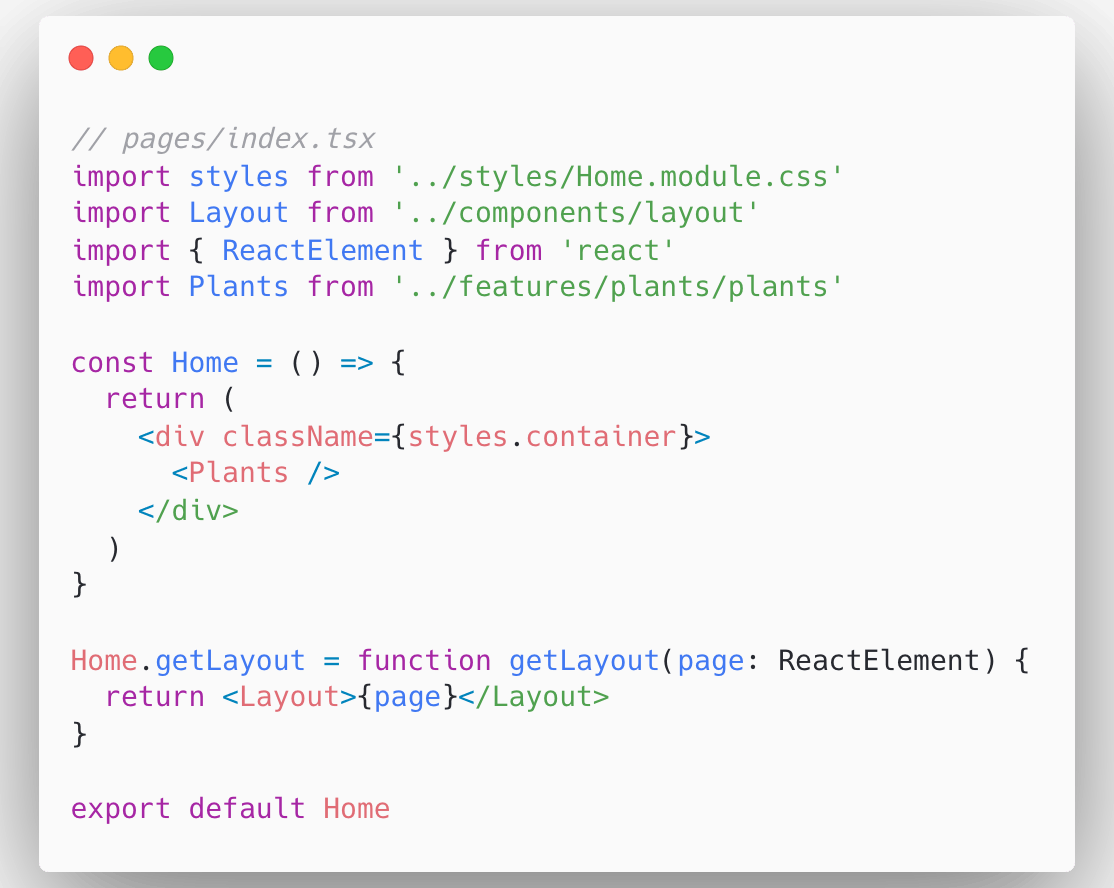
Our index.tsx file exposes a Home component that will be the home route for our application.
The next step is defining our webmap route for the application. This page will display our webmap and filter the results to only display the Power Plants type that was selected from the list in the home page. To make this more configurable, we can also add a ?type= parameter to the URL string so we can share this link with other users later on.
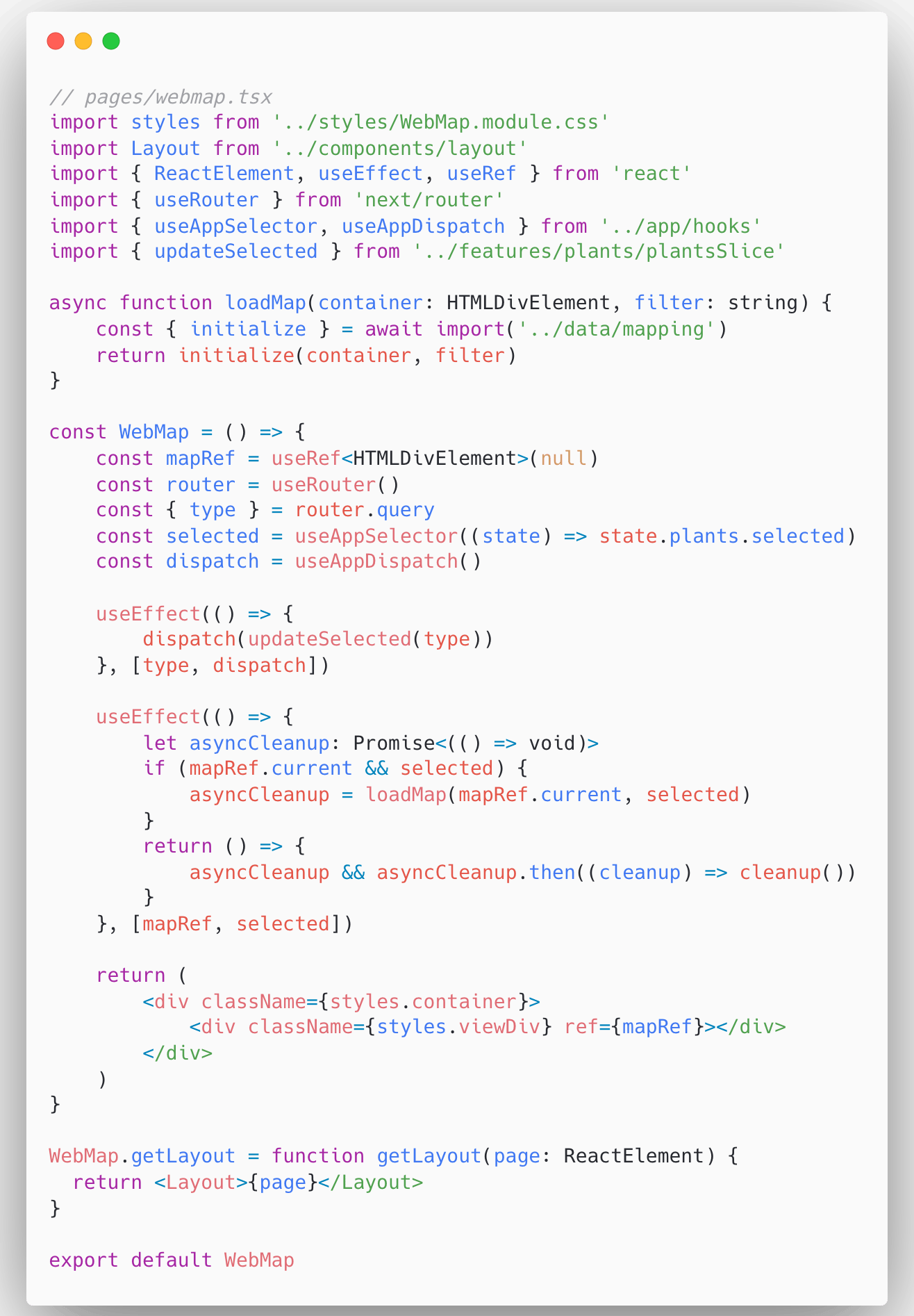
There are few things happening here. We’re using the provided routing hooks from NextJS to get the query parameters. We also manage a little bit of state to display a button to navigate back to the home page. Notice there is no reference to the ArcGIS API for JavaScript in this component. We have a loadMap() method that dynamically imports a mapping module. This mapping module is how we communicate with modules from the ArcGIS API for JavaScript.
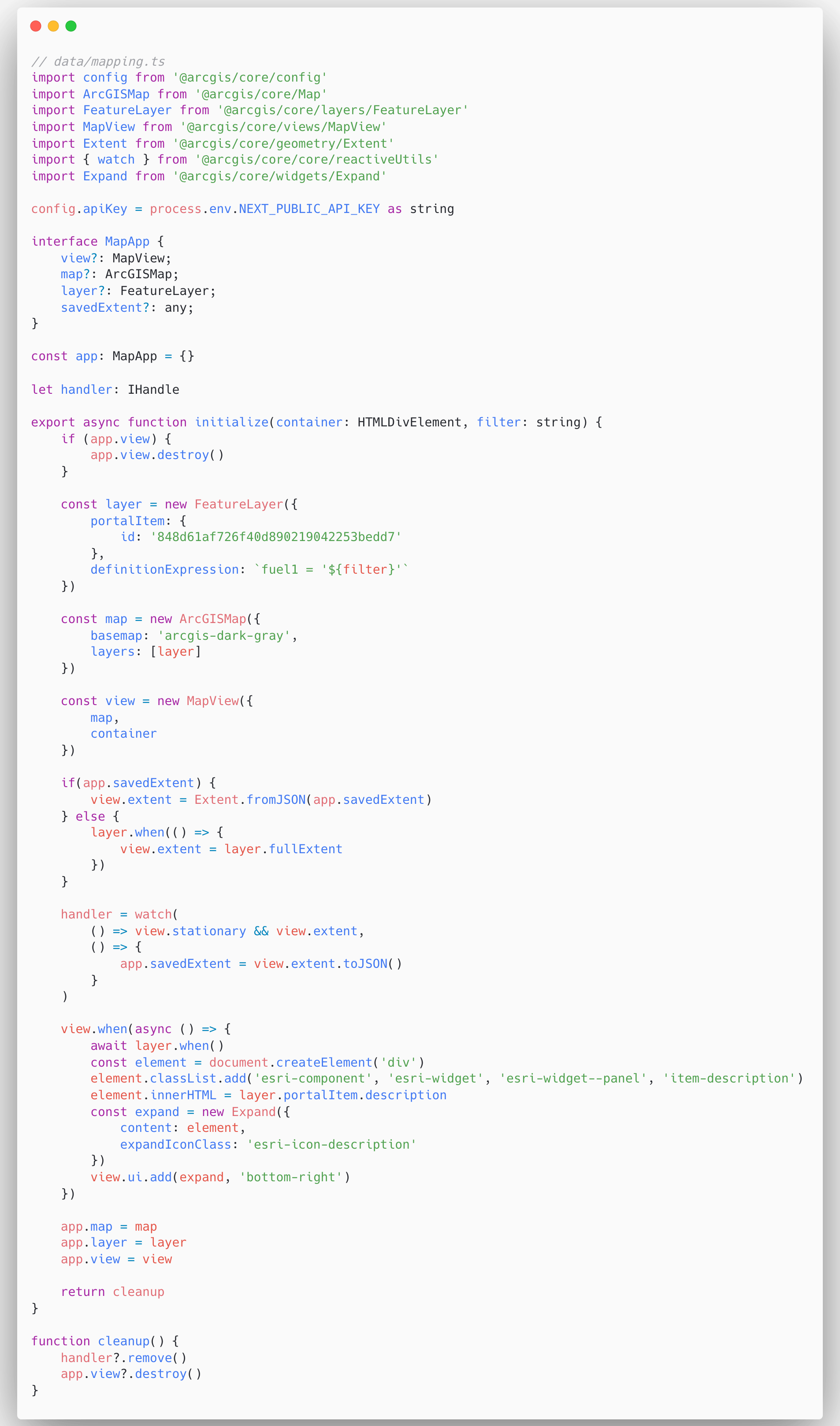
This mapping module creates a thin API layer in our application to communicate with the ArcGIS API for JavaScript and our application components. The initialize method creates the map and layer. It also saves the extent as a JSON object as the user navigates the map. So when the user navigates to the home page and comes back to the map, their last viewed location will saved and reused again. This is a useful way to provide a more seamless user experience.
This is what the finished application looks like.
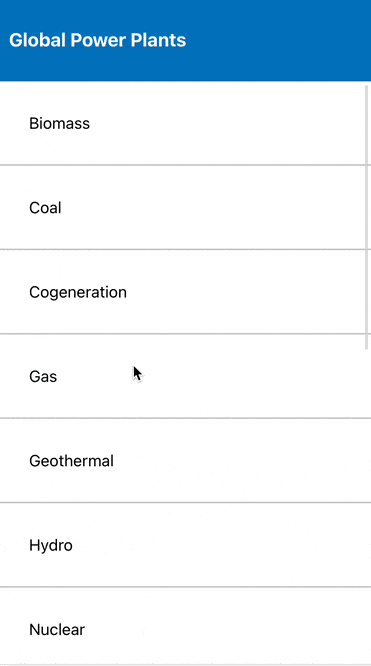
Deployment
NextJS leverages what is called serverless functions. Serverless functions are short lived methods that last only seconds, spun up for use and quickly destroyed. NextJS uses them for the API route when serving pages. You will need to keep this in mind when you deploy your application. It should be noted, NextJS is developed by Vercel, and they do offer a hosting solution that works with serverless functions. Other platforms like Heroku and Amazon do as well. It’s up to you to decide where you want to deploy your application to use these serverless functions. For demo purposes, I deployed the application to Heroku here.
Summary
NextJS is a powerful React framework you can use to build scalable production ready applications using the ArcGIS API for JavaScript. You can use tooling like Redux to help you manage your application state, and even use the ArcGIS API for JavaScript to query mapping services in serverless functions. This application also provides the benefits of fast load times by deferring loading the map until necessary.
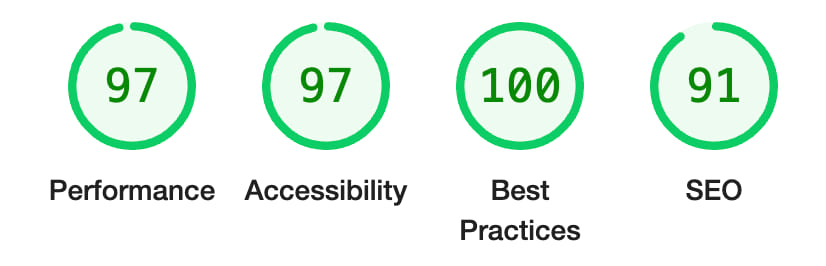
The combination of NextJS and the ArcGIS API for JavaScript provide a great developer experience, and one that I highly recommend you try for yourself. Have fun, and build some awesome applications!
Article Discussion: