The 4.7 and 3.24 versions of the ArcGIS API for JavaScript introduce the client-side projection engine. The big advantage of using this engine is its ability to convert geometries from one spatial reference to another on the client-side, so that no network requests are required. This means that using the client-side projection engine instead of GeometryService projection can significantly improve the performance of web applications, particularly if they make frequent requests to GeometryService or project a large number of geometries.
There are several things to remember when using the client-side projection engine:
- The client-side projection engine will only load in browsers that support WebAssembly.
- At this time, the client-side projection engine supports equation-based geographic transformations only. You can specify a specific equation-based geographic transformation for the project operation, or accept the default transformation if one is needed.
- When projecting array of geometries, the project method will use the input spatial reference of the first geometry in the array as the input spatial reference. So make sure that all geometries are in the same spatial reference.
Versions 4.7 and 3.24 already take advantage of the client-side projection engine in several places. For example, the CSVLayer in version 4.7 can be re-projected on the client-side if its spatialReference does not match the view’s spatialReference.
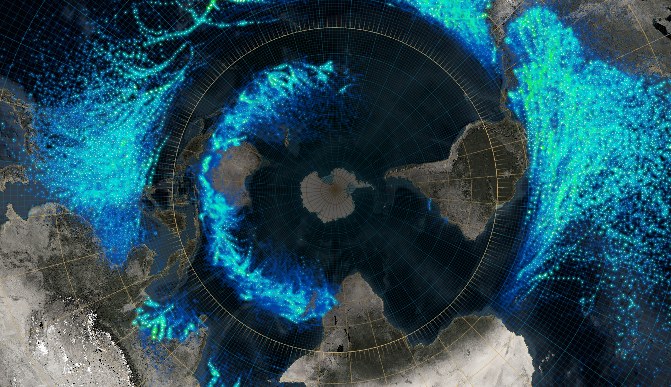
The coordinateFormatter module is also new at 4.7 and 3.24. It converts between points and specified formatted coordinate notation strings such as decimal degrees and U.S. National Grid (USNG). This module takes advantage of the client-side projection engine. This 3.24 sample highlights the use of client-side projection engine. One of the things it does is display the re-projected coordinates of the cursor in real-time in the bottom left corner of the application. This is accomplished by re-projecting the point from Winkel III projection to WGS84 projection using the project method, then passing the projected point to coordinateFormatter.toLatitudeLongitude method. Projecting a point using the GeometryService would not be ideal and as responsive in this case.
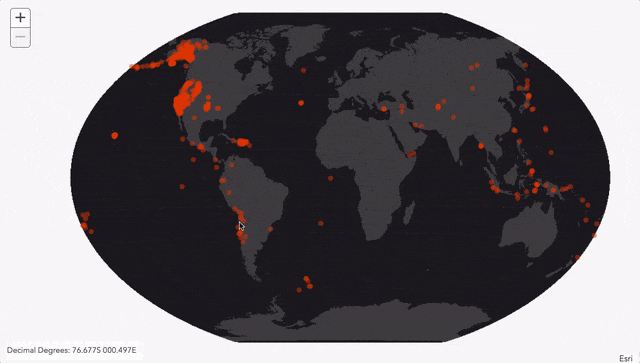
Using the client-side projection engine
To use client-side projection, the application must reference the projection module and should check if the projection engine is supported by the browser. If it is supported then load the projection engine. Once the projection engine is loaded successfully, the geometries can be converted from one spatial reference to another by calling its project() method. Example code to convert geometries from one spatial reference to another could as simple as the following:
require([
"esri/geometry/projection",
"dojo/domReady!"
], function (
projection
) {
if (!projection.isSupported()) {
alert("client-side projection is not supported");
return;
}
// load the projection module
projection.load().then(function () {
// project an array of geometries to the specified output spatial reference
projectedGeometries = projection.project(geometries, outSpatialReference);
});
});
Performance compared to GeometryService projection
If the GeometryService was used to convert geometries from one spatial reference to another, each individual projection operation would require separate requests to the server every time the project() method is called. The sheer number of requests made to the server (with each mouse-move for example) would slow down the application. However, the client-side projection removes the need for requests made to the server, which makes the application more reactive.
To see the differences in performance between the two modules, check out this sample that compares the time it takes to project large polygons with the client-side projection engine versus the GeometryService. The sample converts polygons representing world continents and oceans from WGS84 to a specified projection using the client-side engine projection and GeomeryService projection operation. As you load the application and change the projection, you should notice that the client-projection is faster compared to GeometryService projection. The time it takes the client-side projection and GeometryService to produce results will vary depending on number and complexity of geometries, network speed, and browser; in most cases, however, the client-side projection engine will perform faster. You can get the source code for this sample from here.
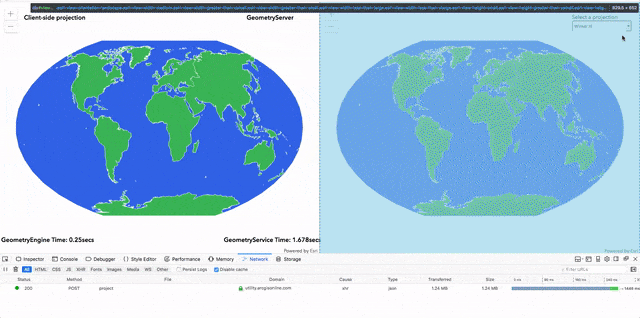
Keep in mind that the client-side projection is not necessarily the correct solution for every projection issue. The client-side projection engine only supports equation-based geographic transformations, while the GeometryService project operation can convert geometries with equation-based geographic transformations, grid based geographic transformations, and vertical transformations.
We hope client-side projection engine will make you more productive in your application development. We are very excited to see how you will use client-side projection in your applications.
Commenting is not enabled for this article.