We are pleased to announce OGC Indexed 3D Scene Layers (I3S) Community Standard is now supported in the latest release of CesiumJS, Version 1.99. This Esri contribution allows for I3S 3D Object and IntegratedMesh Scene Layers to be directly consumed in CesiumJS. Furthermore, it also allows for displaying and symbolizing any feature or mesh attributes associated with the I3S layer.
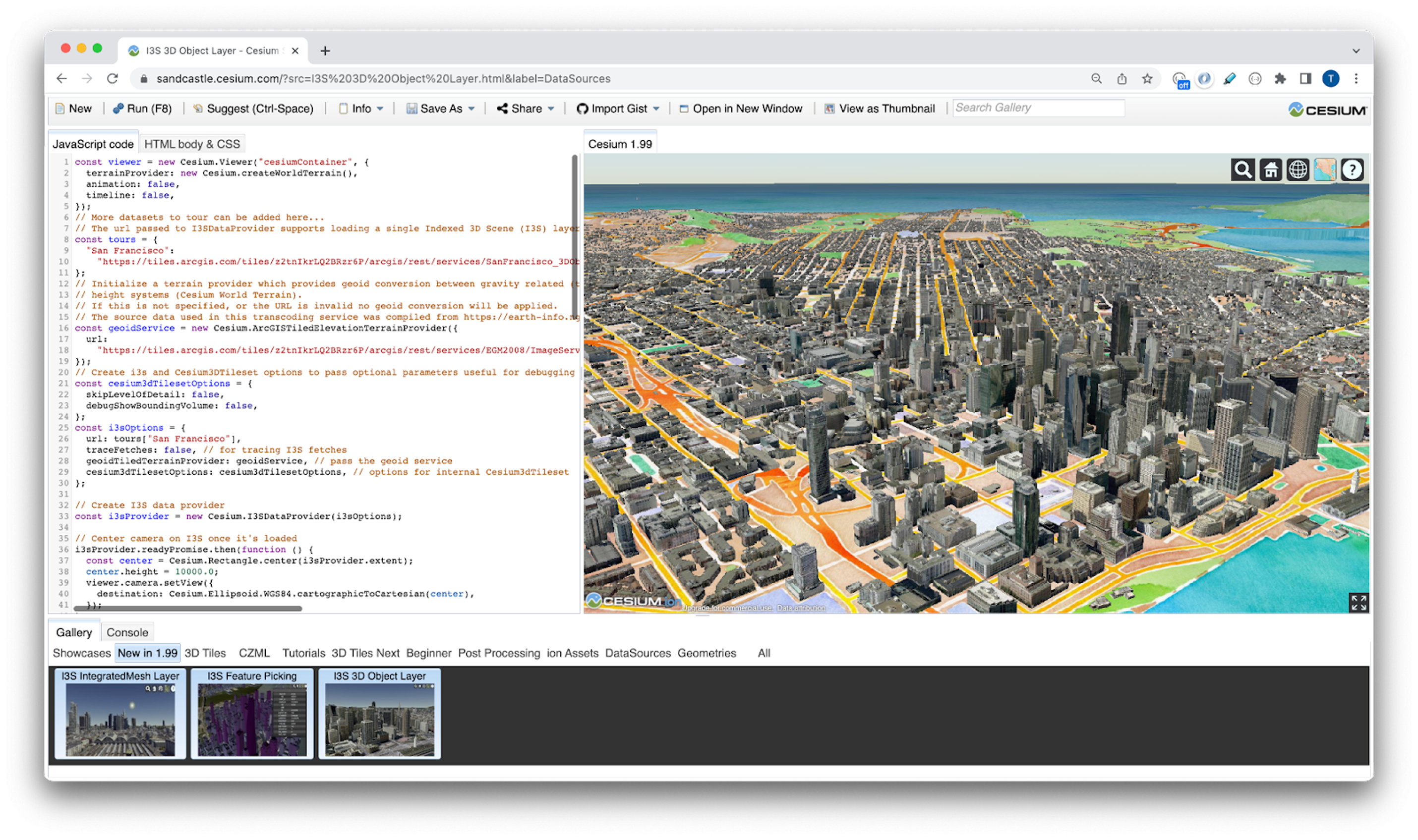
I3S adoption in Free and Open Source Community (FOSS)
I3S has been gaining much traction in the Free and Open Source Community (FOSS). Since 2019, Loaders.gl and Deck.gl frameworks added support for loading and visualizing 3D Object and IntegratedMesh I3S Scene layers. Recently, Version 3.0 of Loaders.gl added support for I3S Building Scene Layer (BSL) type. I3S BSL released in March 2019 by Esri is now going thru OGC adoption process for inclusion as part of I3S OGC 1.3 community standard.
Esri also worked with the Loaders.gl community to create an open source Tile Converter, a command line utility (CLI) for two-way batch conversion between I3S and 3D Tiles.
Continuing this trend, the current contribution adds a new scene primitive in CesiumJS allowing just in time (JIT) transcoding of I3S geometry payload, materials and texture to glTF (glb). The JIT transcoding occurs for both binary geometry data as well as I3S resources required for efficiently traversing an I3S data structure (encapsulated as a paged resource). Textures in jpeg/ktx2/basis format are directly consumed without any transcoding.
Architecture of I3S support in CesiumJS
I3S 3D Object and IntegratedMesh layer support is achieved by processing the scene layer resource of an I3S dataset to create a Cesium 3D Tileset. Once a Cesium 3D Tileset is created, we process the root node of the I3S layer to create a root Cesium 3D Tile, parented to the Cesium 3D Tileset. The CesiumJS system then loads all children of the root tile. For each child, we create a placeholder 3D Tile so that the Cesium LOD display can use the tile’s selection criteria, maximumScreenSpaceError, derived from an I3S nodes’ maxScreenThreshold property.
Leveraging CesiumJS’ refinement and LOD replacement architecture, if the newly created Cesium 3D tile is visible, CesiumJS invokes requestContent on it. At that moment, we intercept the call to requestContent and re-implement Cesium3DTile.prototype.requestContent enabling us to load the I3S geometry data transcoded on the fly to glTF format. Once the geometry is loaded, we then load all children of this node as placeholders so that the CesiumJS LOD system can know about them too.
Transcoding Architecture
We use web workers to transcode I3S geometries into glTF. The steps involved include decoding geometry attributes (positions, normals, etc..) either from draco or Binary format. If requested, when creating an I3SDataProvider the user has the option to specify a tiled elevation terrain provider (geoidTiledTerrainProvider). The geoidTiledTerrainProvider enables conversion of heights for all vertices and bounding boxes of an I3S layer from (typically) gravity related (Orthometric) heights to Ellipsoidal. This step is essential when fusing data with varying height sources. For example, fusing a gravity related I3S dataset with cesium world terrain (ellipsoidal).
The final phase in the transcoding process is the transformation of vertex coordinates from longitude, latitude and height based system to Cartesian in local space. This phase takes into account appropriately scaling of the positional values as well as cropping of UVs (if present) – when scale and UV regions are defined in the attribute metadata, respectively. The transcoding process concludes by creating a glTF document in memory that will be ingested as part of a glb payload.
Sandcastle example walkthrough
The main public class for I3S support is I3SDataProvider. I3SDataProvider accepts the url of an I3S dataset allowing the creation of a data object that can be directly inserted into a scene’s list of primitives and should behave transparently. I3SFeature, I3SNode and I3SLayer classes implement the Object Model for I3S entities, with public interfaces.
const i3sData = new I3SDataProvider ({
url:
'https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/Frankfurt2017_vi3s_18/
SceneServer/layers/0' //add ?token=<token> for secure services
});
viewer.scene.primitives.add(i3Data);
Orthometric height systems vs ellipsoidal
To seamlessly fuse datasets created on disparate vertical coordinate systems (VCS), one needs to perform harmonization of height systems with which datasets are recorded in. This is typically achieved by leveraging a pre-computed/compiled lookup table – Interpolation Grid codes – at various resolutions, such as the one available from Earth Gravitational Model 2008 (EGM2008) Data.
As part of this implementation, we provide a service-based architecture avoiding bloated look up files to convert gravity related (a.k.a orthometric height) datasets to ellipsoidal heights. In other words, if an I3S Datasets is based on orthometric height values (above sea level), we transcode it to be meters above an ellipsoid (typically WGS84). This is done so that I3S datasets with gravity related height systems match when fused with ellipsoidal based terrain datasets, such as the Cesium World Terrain.
How Does it Work ?
When I3S layers with orthometric height systems are used in conjunction with ellipsoidal based terrain data, executing the code below achieves height system harmonization. This is achieved by performing geoid conversion of the geometric primitives and bounding volumes of the I3S layer in such height systems to ellipsoidal based height system. The required conversion is happening, before the I3S geometry payloads are converted to glTF.
const geoidService = new Cesium.ArcGISTiledElevationTerrainProvider({
url: 'https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/EGM2008/ImageServer'});
let i3sData = new I3SDataProvider ({
url:
'https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/Frankfurt2017_vi3s_18/
SceneServer/layers/0', //add ?token=<token> for secure services
geoidTiledTerrainProvider: geoidService});
viewer.scene.primitives.add(i3Data);
The conversion of orthometric heights to ellipsoidal uses an ArcGIS Image Service, that provides the Interpolation Grid, Earth Gravitational Model 2008 (EGM2008) Data, as an Elevation service. A prior collaboration between Esri and Cesium, added support for consuming Esri World Elevation Service in CesiumJS via ArcGISTiledElevationTerrainProvider. ArcGISTiledElevationTerrainProvider is a TerrainProvider that produces terrain geometry by tessellating height maps retrieved from Elevation Tiles of an an ArcGIS Image Service.
As a result, an I3SDataProvider accepts, as an optional parameter, what we call, geoidTiledTerrainProvider.
When a geoidTiledTerrainProvider is provided, we sample the geoid height at each I3S positional vertex data and shift by the difference between that value and the height at the center of the tile. To make this conversion performant, this is done in a web assembly module asynchronously. I3SNode.prototype._createContentUR is responsible for loading the geometry data as well as for creating and scheduling a TaskProcessor, that converts orthometric heights to ellipsoidal, by sampling the geoid. Similarly, an I3S bounding volume also goes through similar transformation before being persisted to a Cesium3DTileset object.
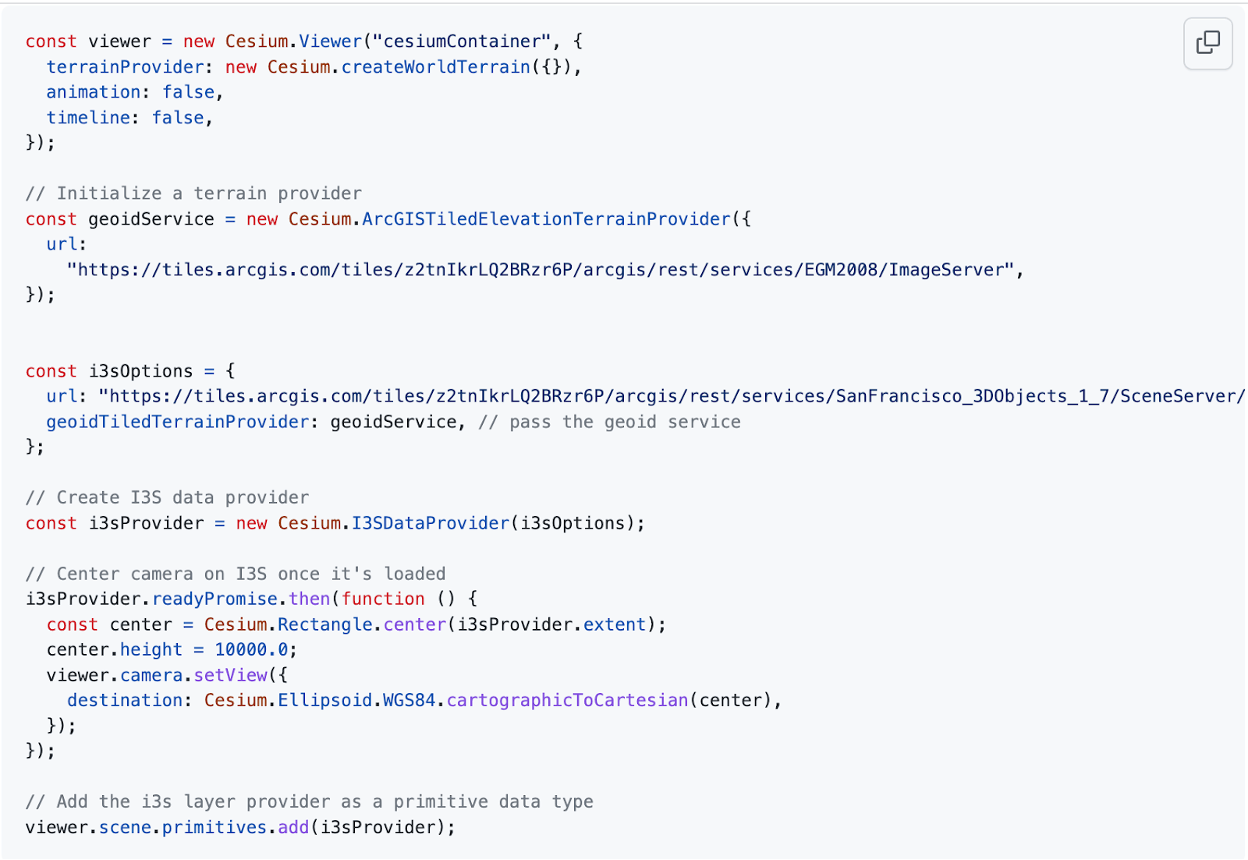
Evolution of a Standard
I3S as an OGC Community Standard for streaming and storing massive amounts of geospatial content has been rapidly evolving to capture new use cases and techniques to advance geospatial visualization and analysis. I3S enables efficient encoding and transmission of various 3D geospatial data types including 3D objects, integrated surface meshes, point cloud as well as highly detailed BIM (Building Information Model) content. Thus, I3S enables an interactive visualization experience on web browsers, mobile, and desktop apps for both offline and online access.
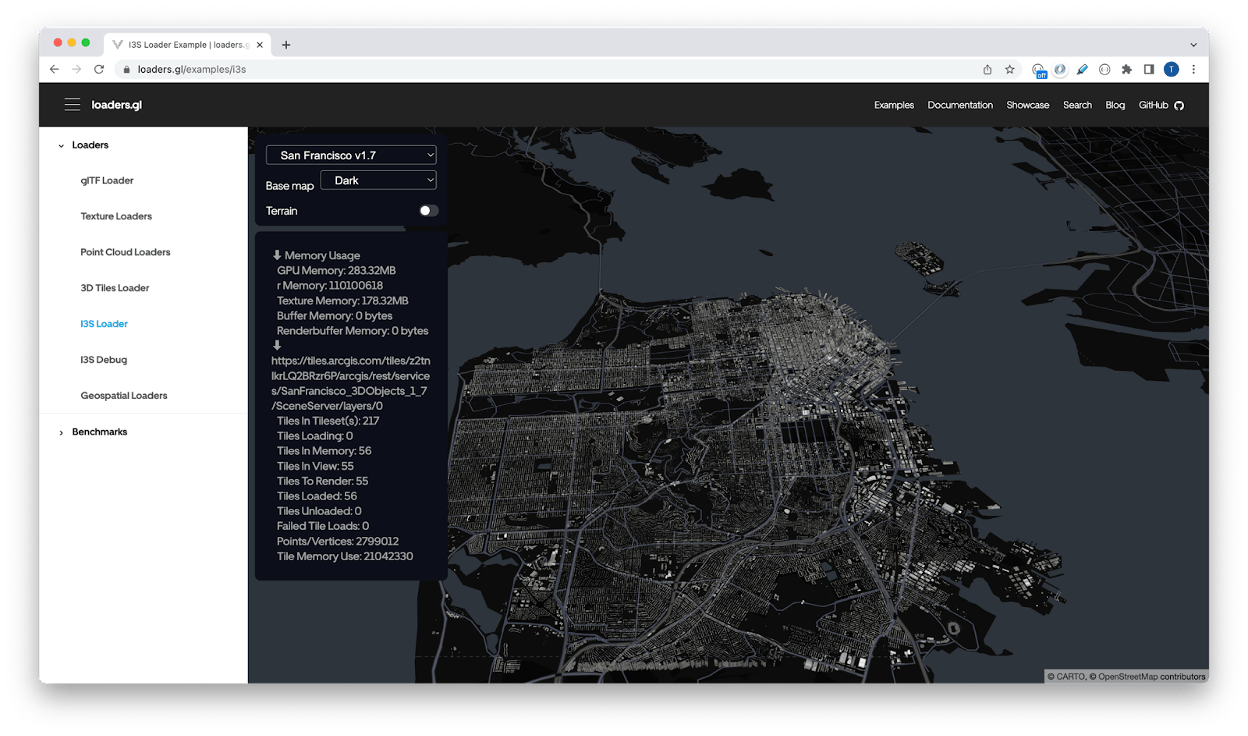
Loaders.gl & Deck.gl allow loading and visualization of 3D Object, IntegratedMesh and Building Scene Layers (OGC I3S Version 1.2 & OGC I3S Version 1.3 (candidate)).
One aspect of I3S standard that has been evolving is its support for cross platform compatibility for texture assets. Performant 3D systems routinely utilize compressed textures, as an essential optimization technique to support the loading and display of massive amounts of textures in 3D applications. With GPU-decodable Supercompressed Textures (GSTs) becoming an efficient delivery mechanism, I3S has adopted the Basis Universal Texture format as its choice of GST. OGC I3S Version 1.2 was the first 3d geospatial streaming format to standardize on Basis Universal SuperCompressed Texture format in khronos KTX™2.0 containers.
Basis Universal has excellent decoding speed, great compression rates (while keeping the visual quality) and cross platform compatibility. An area that needed improvement was its encoding rate. As detailed in this blog, Esri collaborated with Binomial to improve the texture codec speed of Basis Universal by over 3X in early 2021. Further continuing the engagement this year, we were able to achieve an additional 10x improvement in the encoding speed, when using GPU in parallel. Both these improvements are part of the open source Basis Universal encoder available at github.
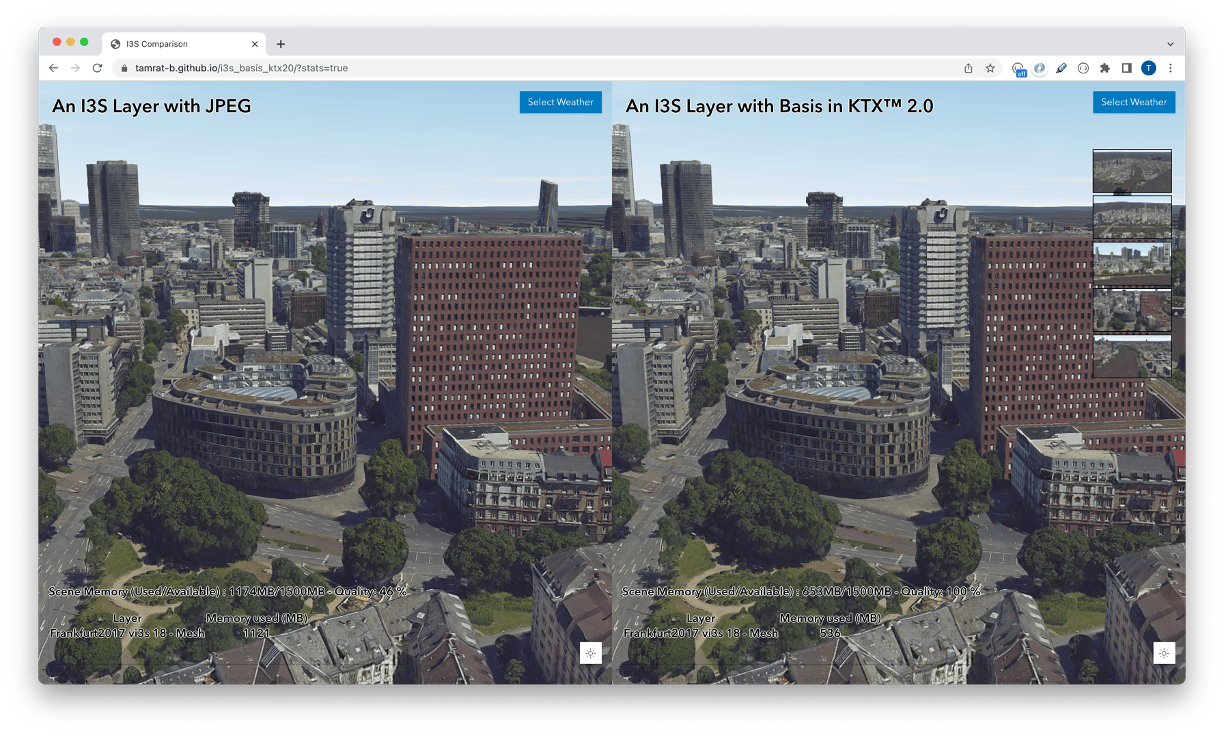
I3S OGC 1.3
As a living breathing specification and standard, I3S has been evolving ever since it was released as the first OGC 3D streaming standard in the fall of 2017. The latest Version, I3S OGC 1.3, currently under voting, adds support for Building Scene Layers (BSL). A Building Scene Layer is a 3D representation of a building model. A building model may be derived from 3D construction content, such as BIM data, or from a relational database model that contains 3D spatial information. The I3S BSL capability is designed to model the organization of construction data by grouping content into standard engineering disciplines. Content in a BSL may represent a partial building, an individual building, or multiple buildings on a campus.
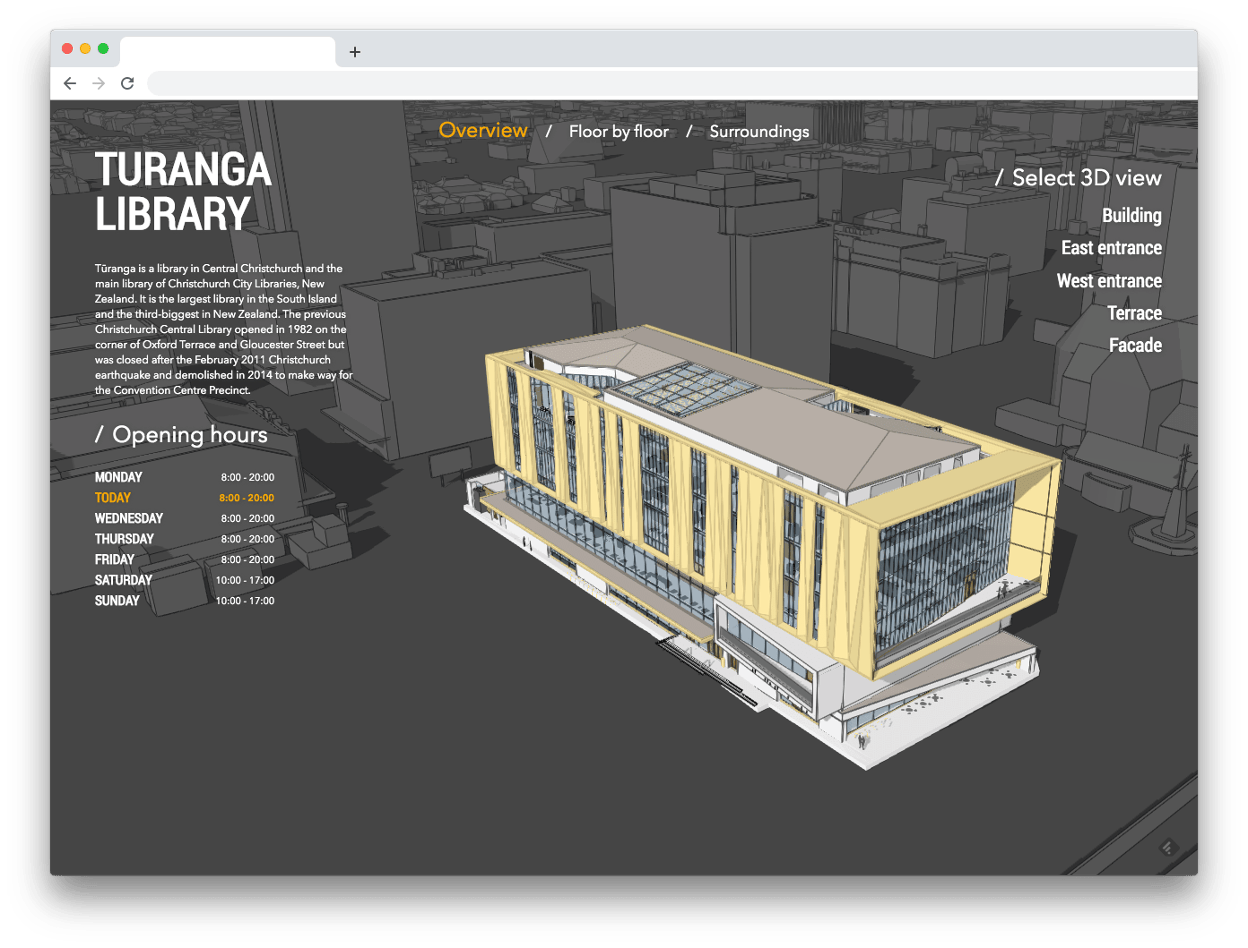
An example of an interactive Building Viewer 3D website based on ArcGIS API for JavaScript for Turanga Library building. The live application code and sample walk thru blog explaining how to use an OGC I3S 1.3 (candidate) Building scene layer content in an intuitive website allowing you to explore different floors, navigate and visualize the rooms and spaces.
Summary
As stated above, I3S adoption in the Free and Open Source Community (FOSS) has been increasing. Since 2019, loaders.gl and deck.gl – a collection of open source loaders and writers for file formats focused on visualization of big data, including tabular, geospatial, and 3D formats, has added support for loading and visualizing 3D Object and IntegratedMesh I3S Scene layers. Recently, Version 3.0 of Loaders.gl added support for I3S Building Scene Layer type. I3S Building Scene Layer was released in March 2019 by Esri and is now undergoing adoption vote for inclusion as part of I3S OGC 1.3 community standard.
This contribution is an attempt to further increase I3S adoption in the FOSS community. Coupled with the open source Tile Converter that allows for a a two-way batch conversion between I3S and 3D Tilest, we believe this work further advances interoperability goals in the geospatial community in general.
So go on, give the Sandcastle a try and let’s know your feedbacks.
Article Discussion: