Introduction
The August update of Native Maps SDKs brings some exciting new capabilities for 3D visualization and application development. Read on to learn about those capabilities.
Support for 3D objects in relative surface placement mode
3D object scene layers have been supported by the Native Maps SDKs and Runtime SDKs since version 100.0. They are part of the I3S specification and enable you to add GIS data, such as 3D features in your scene. The 200.2 release of the Native Maps SDKs adds support for relative placement mode in addition to absolute placement mode, which allows features in a 3D Object Scene layer to be placed relative to a scene’s surface.
Relative placement is key to supporting the new global OSM buildings layer (published by Esri in the Living Atlas) and you can now include it in your 3D scenes to provide a rich context for your data, anywhere in the world.
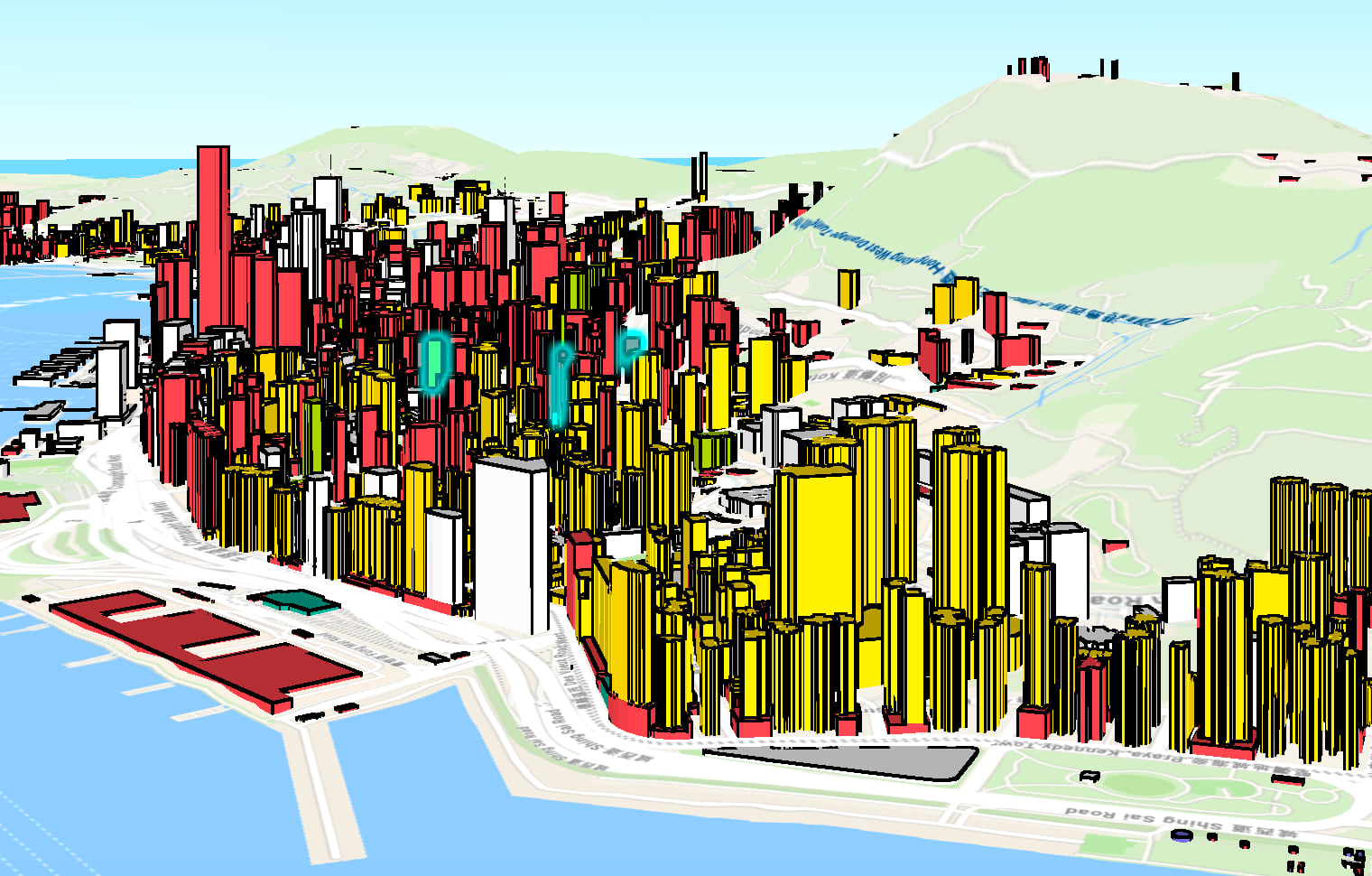
OSM buildings layer showing buildings in relative surface placement mode.
The OSM buildings layer can be added to a basemap to provide more realistic context to data in your 3D application. This sample code shows how to create a basemap that combines the OSM buildings layer and a suitable vector tile base layer using the ArcGIS Maps SDK for Qt, but all the concepts also apply to Java, Kotlin, .NET and Swift Maps SDKs:
⊕ Show code …
// create a vector tiled layer using the portal item used in the OSM 3D basemap ArcGISVectorTiledLayer* vtl = new ArcGISVectorTiledLayer(new PortalItem("1e7d1784d1ef4b79ba6764d0bd6c3150", this), this); // create a scene layer using the portal item for the buildings layer in the OSM 3B basemap ArcGISSceneLayer* osm_blds_lyr = new ArcGISSceneLayer(new PortalItem("ca0470dbbddb4db28bad74ed39949e25", this), this); // create a new basemap object Basemap* basemap = new Basemap(this); // add both the layers to the basemap's baselayers basemap->baseLayers()->append(vtl); basemap->baseLayers()->append(osm_blds_lyr); // create a new scene with the basemap m_scene = new Scene(basemap, this);
Note: Esri 3D basemap services are currently in beta and are expected to be released for production use later this year. The Native Maps SDKs currently support a subset of the layers of these basemaps, and the above code builds a Basemap object programmatically using these supported layers. When publishing a web scene containing the OSM buildings layer, the Native Maps SDKs cannot currently read that layer if it is part of the basemap and it should be added to the web scene's operational layers. Full support for 3D basemaps, including trees and labels, is on the roadmap for future Native Maps SDK releases.
The OSM buildings layer can of course also be added as an operational layer in Native Maps SDKs, like any other ArcGISSceneLayer.
Since the OSM buildings layer delivers detailed buildings at a global scale, the Native Maps SDKs team has worked hard to ensure the layer performs well, whether as part of a scene’s basemap or its operational layers.
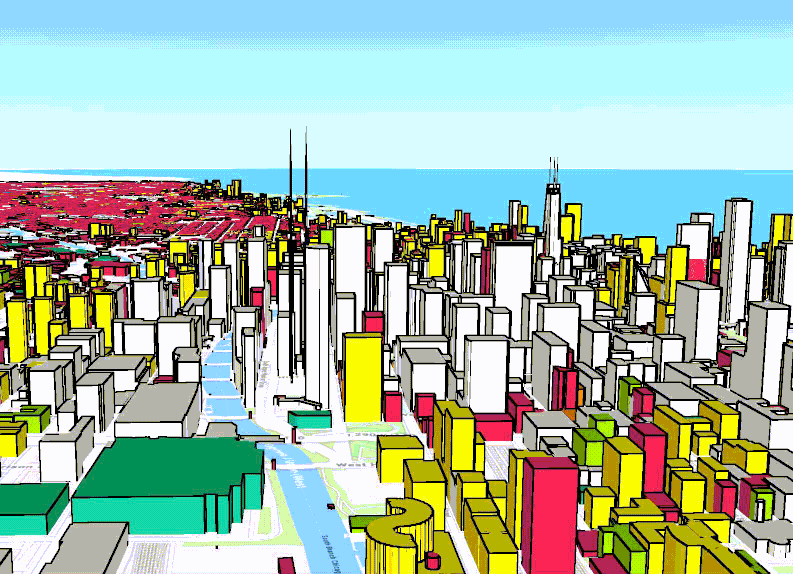
OSM buildings layer in the ArcGIS Maps SDKs for Native Apps
Support for filtering features in an ArcGISSceneLayer
The new OSM buildings layer provides great context for your data, but to see your data, you’ll often need to remove certain buildings from the OSM buildings layer, for example to view a proposed development. To let you make way for your data, we’ve added feature filtering. OSM building layer features can be filtered using a geometry, or by specifying features to show or hide.
The video below shows adding the OSM buildings layer as a basemap. The red line shows the boundary of a custom dataset of San Francisco building models. A filter is applied to hide the OSM buildings within the extent of that custom dataset which is then added and displayed without conflicting with the OSM buildings.

Filtering of features and replacing with custom dataset.
Filtering can support different workflows across various industries ranging from city planning to transportation. A typical planning workflow could entail choosing to use the OSM buildings layer as the basemap layer and then add a custom 3D model to replace the relevant features in the OSM buildings layer as in the above example. This would create a much more realistic context for data visualization. Similarly, in the transportation analysis industry, visualization and analysis of replacing a feature representing an older structure with a custom, higher-quality dataset can be easily performed.
Filtering isn’t just limited to the OSM buildings layer. You can apply it to any 3D object layer. In fact, you can also use filtering on point scene layers as well since the API is part of ArcGISSceneLayer.
Those are some basic examples of the possibilities feature filtering in ArcGISSceneLayers. Let’s take a look at the APIs that bring it to life.
There are three separate ways to perform a filtering operation, which are described below.
Per-feature filtering
Using this method, features can be identified and then a filter applied to those features using the new methods added to ArcGISSceneLayer. A single feature can be hidden using ArcGISSceneLayer::setFeatureVisible(), and multiple features can be hidden at once using ArcGISSceneLayer::setFeaturesVisible(). Hidden features can be made visible again by calling ArcGISSceneLayer::resetFeaturesVisible.
This is useful when there is a small set of features that need to be hidden. For example, a city planner decides to present the design of a new mall to the stakeholders. There is a dataset available for the proposed design. In that case, the planner could selectively hide the current structure and replace it with the newly available dataset by simply clicking or tapping on the older building and then adding the new feature as an operational layer. So, how would that look in code?
⊕ Show code …
// wire up the mouse click event to perform an identify operation connect(m_sceneView, &SceneQuickView::mouseClicked, this, [this](QMouseEvent& mouseEvent) { m_sceneView->identifyLayer(m_sceneLayer, mouseEvent.position().x(), mouseEvent.position().y(), 1.0, false); }); // wire up the identifyLayer completed event connect(m_sceneView, &SceneQuickView::identifyLayerCompleted, this, [this](const QUuid&, IdentifyLayerResult* identifyResult) { for (const auto element : identifyResult->geoElements()) { if (dynamic_cast<Feature*>(element)) { // select the feature to be filtered m_sceneLayer->selectFeature(static_cast<Feature*>(element)); // cache the feature to a list for filtering later m_features.append(static_cast<Feature*>(element)); } } }); // filter using the cached features by setting the visibility to false m_sceneLayer->setFeaturesVisible(m_features, false);
A note on why the select call was added. This was made so that upon clearing the filter, the selection set shows which features were filtered. The code below shows how to clear the filter based on selected features.
⊕ Show code …
// wire up selected features completed event connect(m_sceneLayer, &ArcGISSceneLayer::selectedFeaturesCompleted, this, [this](const QUuid& taskId, FeatureQueryResult* featureQueryResult) { FeatureIterator it = featureQueryResult->iterator(); m_features = it.features(this); // make features visible by setting the bool to true m_sceneLayer->setFeaturesVisible(it.features(this), true); }); // call selected features async m_sceneLayer->selectedFeatures();
The code above demonstrates how to filter multiple features at once. To hide a single feature call m_sceneLayer->setFeatureVisible(feature, false)
.
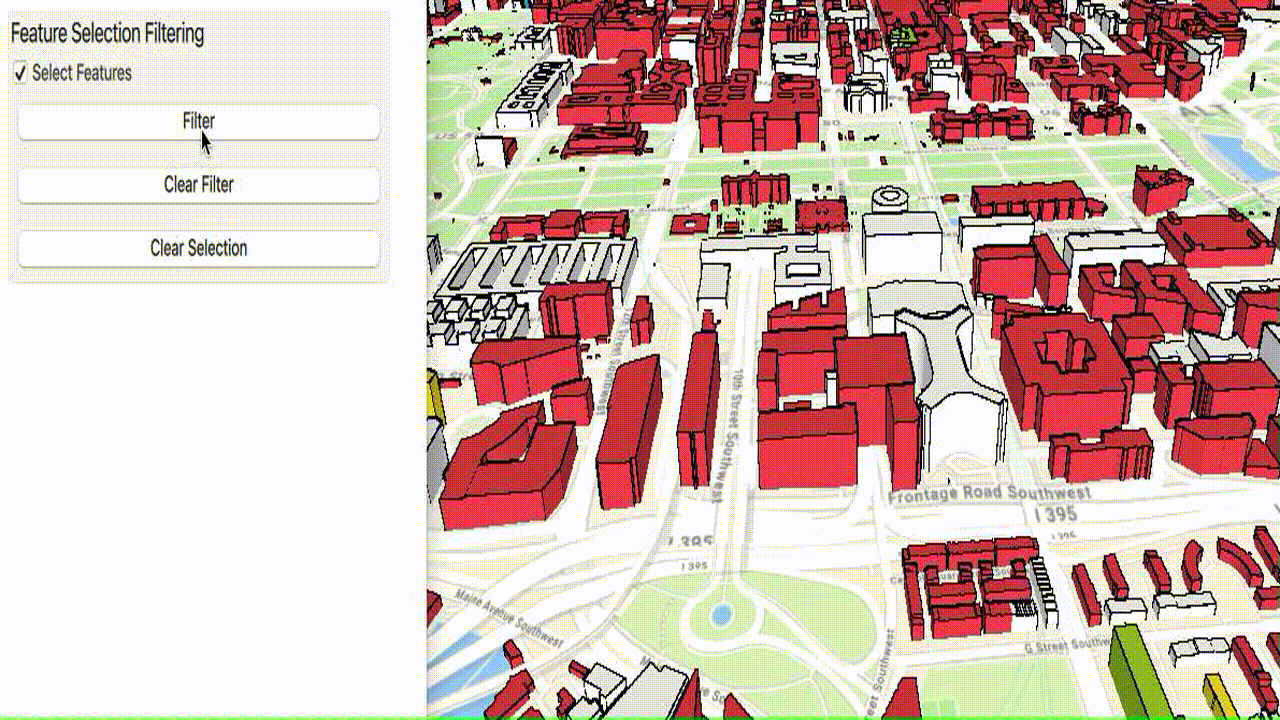
Filtering individual features in a 3D object scene layer
Spatial Filtering
Using spatial filtering, features can be filtered spatially using one or more polygons as input. A new class called SceneLayerPolygonFilter has been added to define the filter. The filter is defined by a set of polygons and a SpatialRelationship. The spatial relationship allows you to choose whether you want to filter features within (contains) or outside (disjoint) of the provided polygons. In the case of contains, everything outside of the polygons would be filtered out and vice versa for disjoint. Once the filter is created, it is applied to the ArcGISSceneLayer via a newly added property called polygonFilter to set it. Spatial filtering allows filtering using multiple polygons, and multipart polygons including polygons with holes.
Spatial Filtering is useful when all features within an area need to be filtered. Consider filtering the extent of a dataset when adding that to an application that has 3D content as part of the basemap.
⊕ Show code …
// create a new polygon filter based on a list of polygons and a spatial relationship m_polygonFilter = new SceneLayerPolygonFilter(m_polygons, SceneLayerPolygonFilterSpatialRelationship::Disjoint, this); // set the polygon filter on the scenelayer m_sceneLayer->setPolygonFilter(m_polygonFilter);
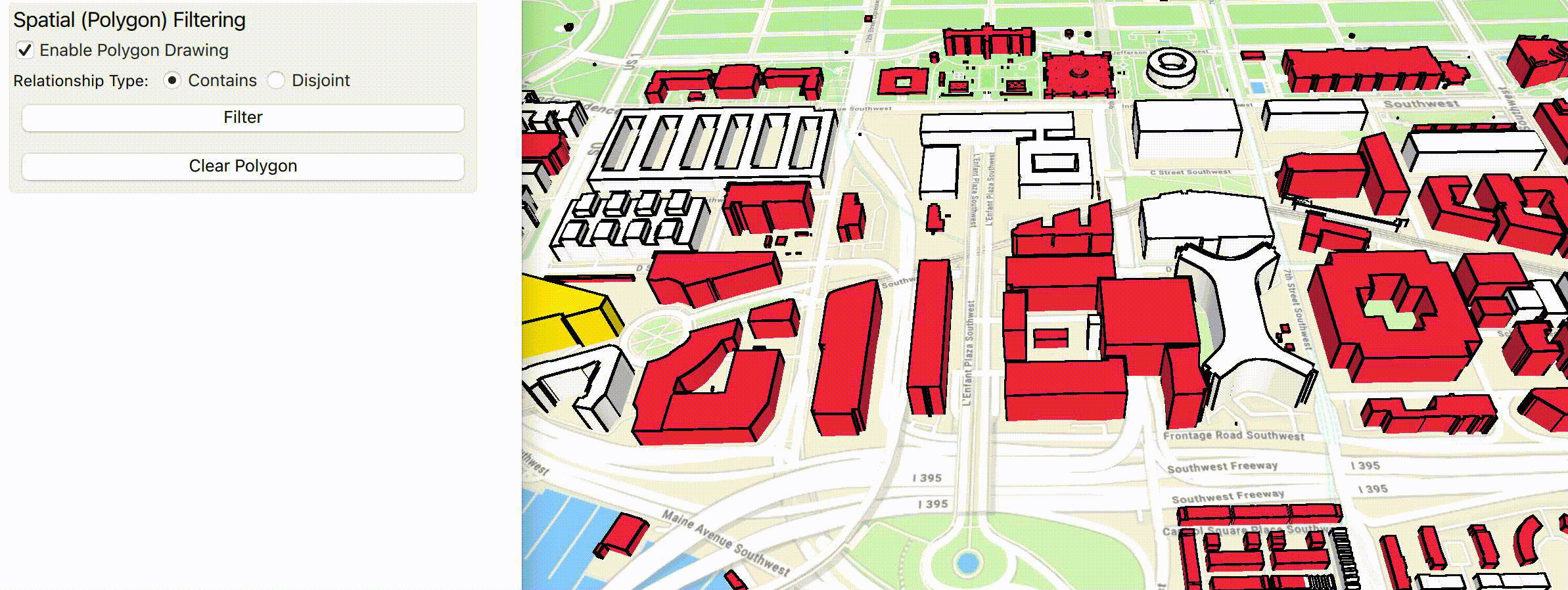
Webscenes published with filters
Webscenes can be published with filters (both per feature filter and spatial filter) applied to an operational layer or layers. The filtering information can be read and honored by the Native Maps SDKs. A new model representing the data that was filtered can then be added as another operational layer for visualization.
Summary
In this article you learned about various advancements we have made to 3D visualization for ArcGIS Maps SDKs for Native Apps. You learned about the support for Relative surface placement for 3D object scene layer. Additionally, you also learned about the filtering capabilities and the different approaches to apply filtering on an ArcGISSceneLayer. To learn more about these new capabilities, please see our documentation and samples that demonstrate these functionalities.
Please feel free to reach out to us with questions or enhancements by posting in the ArcGIS Maps SDKs for Native Apps Esri Community. Happy filtering!!
If you’re new to developing with the ArcGIS Maps SDKs for Native Apps, each SDK (.NET, Qt, Java, Kotlin and Swift) has rich guide documentation, API reference, tutorials, and samples. You can create a free ArcGIS Developer account and API key to get started building your applications.
Article Discussion: