Just like we did with ArcGIS Runtime 100.6 in late 2019, we have been working ever since to bring the capabilities of the utility network to the JavaScript API. We are pleased to announce that the first cut of the utility network API is now available on ArcGIS JavaScript API 4.20. In this blog post we discuss what’s new in ArcGIS Utility Network for JavaScript, illustrate few code samples and finally discuss what’s coming in future releases.
This blog assumes you know the basics of the utility network, to learn more visit our core help page here.
The utility network JavaScript API on 4.20 require the named trace configuration capability which is available in Enterprise 10.9. You will need ArcGIS Enterprise 10.9 and a Utility Network version 5 created with ArcGIS Pro 2.7 or 2.8 to take advantage of the new API.
What’s new for utility network in ArcGIS JavaScript 4.20
This release is focused on delivering the tracing functionality through the new named trace configuration shipped in 10.9. Trace configurations can be created and persisted on a UN 5.0 with Pro 2.7 and later. The persisted trace configuration has all the properties, filters and output required to execute a particular trace. Trace configurations can be shared through webmaps and consumed by simply referencing the trace configuration id (GUID). Read more about trace configurations here.
Opening a WebMap with utility network
Given a webmap created with Pro 2.7 or later with shared trace configuration, the JavaScript API can open the webmap and list all the shared named trace configurations.
Here is an example of how you can load a webmap and any utility network objects shared within it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
async function init(){ //A webmap with utility network layer and shared trace configurations const webmapId = "aafe41463e32497ab56cbac0acf16339" const portalUrl = "https://utilitynetwork.esri.com/portal" //create a webmap object const webmap = new WebMap({ portalItem: { id: webmapId, portal: { url: portalUrl } } }); //create a webmap view and load it into div with id `viewDiv` const view = new MapView({ map: webmap, container: "viewDiv" }); //load the webmap definition await view.when(); //retrieve the first utility network object found in the webmap //this array is empty if no utility network layers are shared in the webmap const utilityNetwork = webmap.utilityNetworks.getItemAt(0) //load the utility network definition and all shared trace configurations etc.. await utilityNetwork.load(); } |
Reading UN Metadata and shared named trace configuration
Once the utility network is loaded successfully, you can query for its meta-data. Let’s consider few examples of properties that can be read from the utility network object.
Reading basic utility network meta-data
While most properties require fully loading the utility network definition via the load method to be accessed, some properties can be accessed without loading the un. Example are layerId, id and title.
1 2 3 4 5 6 7 8 |
//make sure to load the utility network //prints the id of the utility network (UUID) console.log (utilityNetwork.id) //prints the physical datasetname console.log (utilityNetwork.datasetName) //prints the layer id of the utility network console.log (utilityNetwork.layerId) |
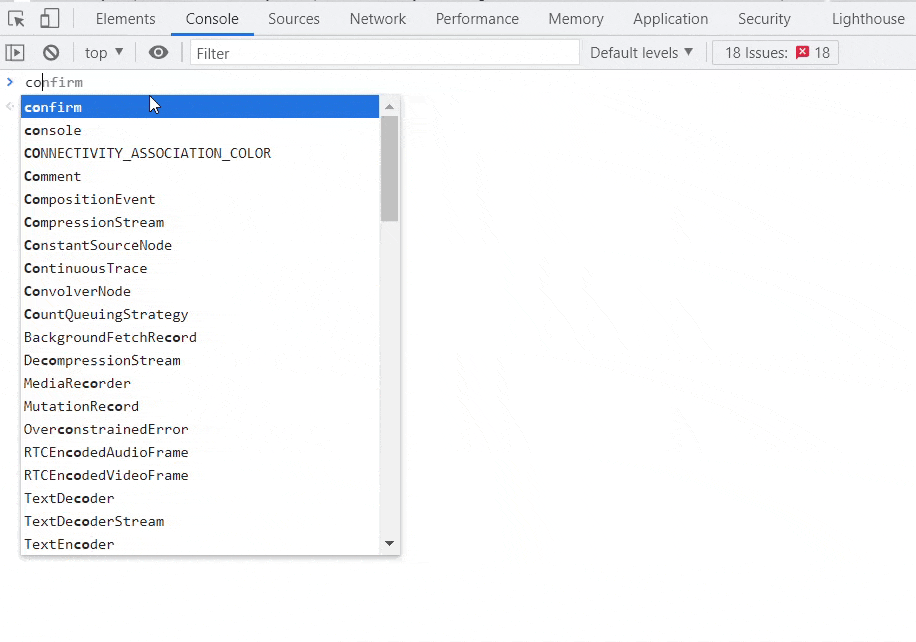
Reading the named trace configurations
During publishing, a webmap containing a utility network can be shared with a list of named trace configurations. These named trace configurations can be accessed through the utiltynetwork object.
1 2 3 4 5 |
//get a reference to the utility network object from the webmap //prints all active named trace configurations, //the title and the globalId of the shared named trace configurations can //be accessed without fully loading the utility network utilityNetwork.sharedNamedTraceConfigurations.forEach(tc=>console.log(tc.title)) |
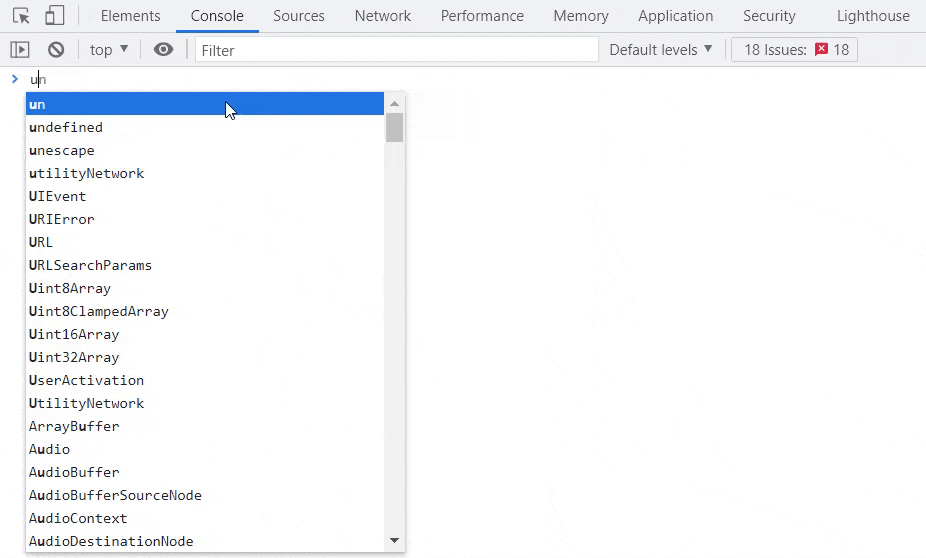
Reading the full named trace configurations
While the title and the globalId properties can be accessed without loading the utility network, access to the full trace configuration require loading the utility network. Here is how to access the result types of a particular named trace configuration.
1 2 3 4 5 |
//get a reference to the utility network object //find the named trace configuration named Connected_Elements. const connectedTraceElements = utilityNetwork.sharedNamedTraceConfigurations.find(tc=>tc.title === "Connected_Elements") //print all result types expected from this trace configuration connectedTraceElements.resultTypes.forEach(r => console.log(r.type)) |
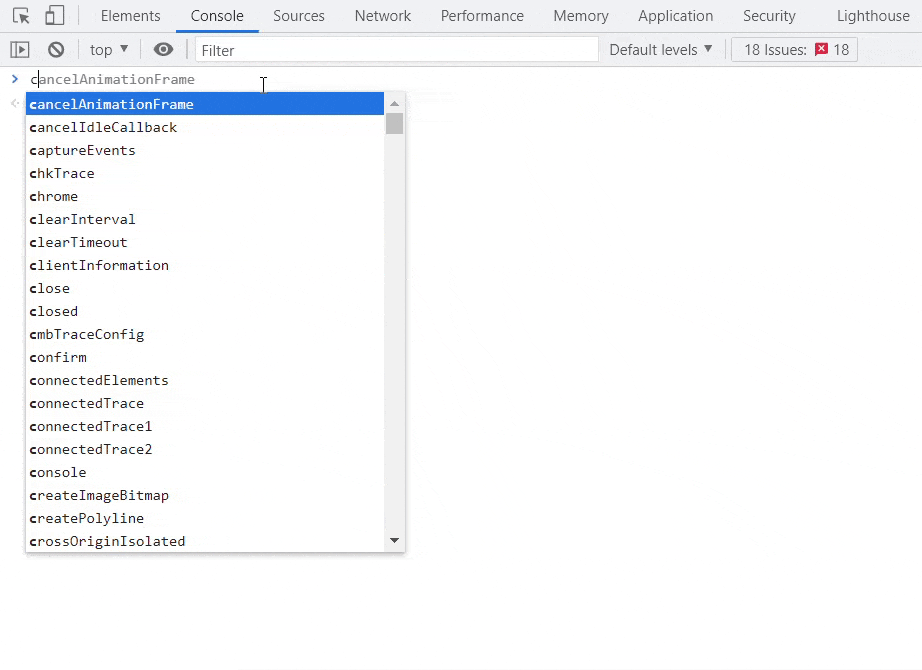
Performing a trace using a named trace configuration
You can perform a trace by providing the globalId of the trace configuration and optional starting locations. You can even provide the version and moment to trace to support tracing in a version or a pervious historical moment.
1 2 3 4 5 6 7 8 9 10 11 12 |
//get a reference to the utility network object //find the named trace configuration Subnetwork_RMT003. This trace doesn't require any starting locations const subnetworkRMT003 = utilityNetwork.sharedNamedTraceConfigurations.find(tc=>tc.title === "Subnetwork_RMT003") //create a trace parameter object and provide const traceParameters = new TraceParameters ( { "traceLocations": [], "namedTraceConfigurationGlobalId": subnetworkRMT003.globalId } ) //execute trace providing the utility network server url and trace parameters const traceResult = await Tracer.trace(utilityNetwork.networkServiceUrl, traceParameters); //print the result count console.log(traceResult.elements.length) |
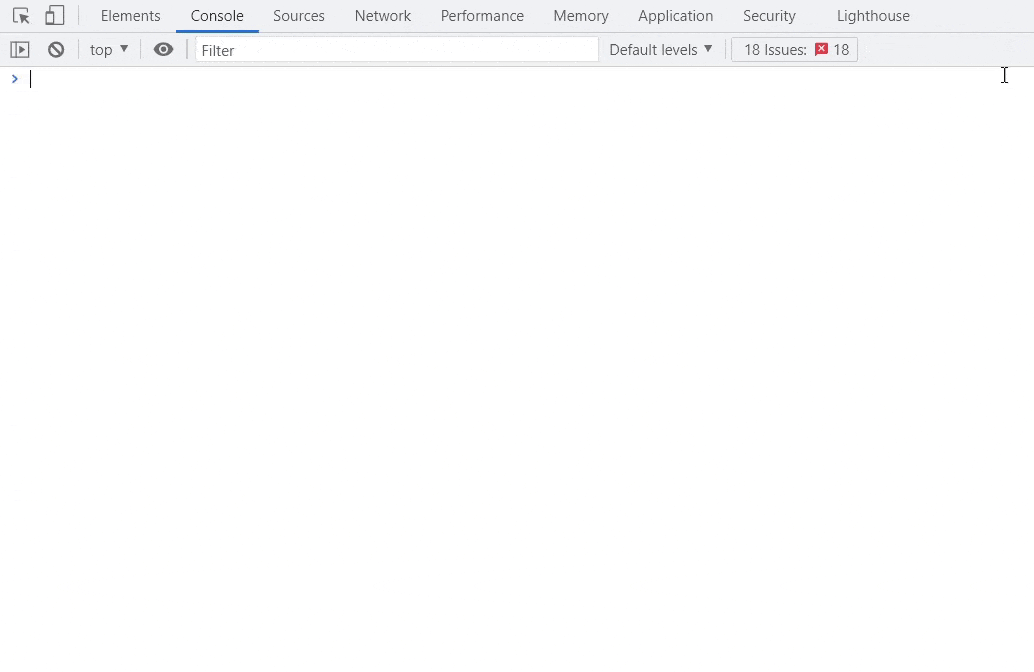
View Associations
In 4.20 we also introduced the ability to view associations located in a given extent. Control exists to filter what type of associations you would like to render and to limit the number of rows to pull.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
//get the current extent const extent = view.extent; //create the list of parameters to view associations //we want to return a max of a 100 structural attachment and connectivity associations only const params = new SynthetizeAssociationGeometriesParameters({ "extent": extent, "returnAttachmentAssociations": true, "returnConnectivityAssociations": true, "returnContainerAssociations": false, "outSR": utilityNetwork.spatialReference, "maxGeometryCount": 100 }) //call the synthesizeAssociationGeometries to synthesize assocaitions and return them const result = await SynthetizeAssociationGeometries.synthesizeAssociationGeometries (utilityNetwork.networkServiceUrl, params) //add associations geometries to the view (remove any existing) view.graphics.removeMany(view.graphics.filter(a=>a.name === "associations")) view.graphics.addMany(result.associations.filter(a => a.associationType === "connectivity").map(g=>getGraphic("line", g.geometry, CONNECTIVITY_ASSOCIATION_COLOR, "associations" ))); view.graphics.addMany(result.associations.filter(a => a.associationType === "attachment").map(g=>getGraphic("line", g.geometry, ATTACHMENT_ASSOCIATION_COLOR , "associations"))); |
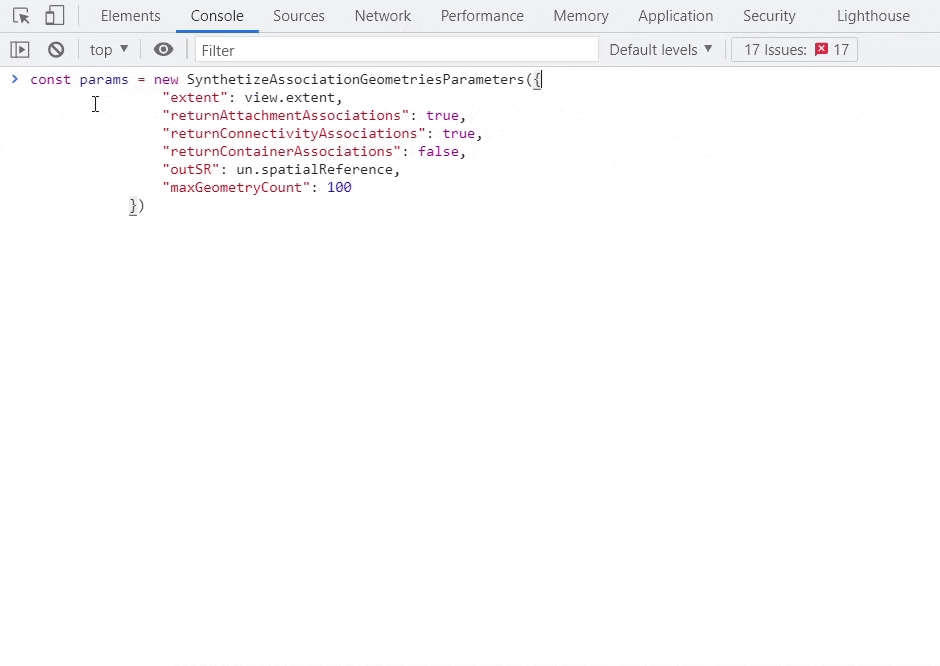
Full demo with source code
Enjoy this full demo of the utility network API for JavaScript, you can find the source code here. At any point you can head to the Esri JavaScript core doc on the API to learn more.
What’s Next?
We are very excited for this first release but of course we are just getting started. Here are few more things brewing in our incubator for the upcoming releases
Override trace configuration
In the 4.20 release, you can execute traces by referencing a persisted named trace configuration global Id. In the coming releases you will be able read and override existing named trace configurations as well as build your own to pass into the trace. This will allow for example the ability to modify a named trace configuration that only returns elements to instead return aggregated geometries without the need to create a new named trace configuration.
Query Network Topology Moments
Additional functionality to read the state of the network topology, such as when it was last validated, when the last schema change was applied and more.
Rule-based Editing, Validate, Subnetwork Management
The ability to run utility network functions such as validate network topology and update subnetwork to maintain network consistency while also exposing network rules for use to derive editing snapping.
Useful Resources
Here are few links that will help you get started.
Overview on the Utility Network

Article Discussion: