You guys asked, and we delivered.
We have been working hard on bringing the utility network capabilities to your devices via ArcGIS Runtime. I’m pleased to say that the first cut of the utility network API is now available on ArcGIS Runtime 100.6. In this blog post we will discuss what’s new in ArcGIS Runtime 100.6 API with regards to utility network. We will go through a few code samples from different programming languages that the ArcGIS Runtime supports such as .NET, IOS-Swift, Qt and Android-Java. Finally we will discuss what’s coming for future releases.
This blog assumes you know the basics of the utility network, to learn about more visit our core help page here.
What’s new for utility network in ArcGIS Runtime 100.6?
In ArcGIS Runtime 100.6 we support the ability to open a feature service that has a utility network through services only, read the metadata and perform connected tracing. We will go through both of these features in detail.
Reading utility network metadata
We have added the ability to open a feature service containing a utility network from runtime and read its metadata such as network sources, asset groups, etc. This allows developers to access the utility network properties and use this to build interesting generic mobile applications and frameworks that works with any utility network out there.
Here is a snippet of how to open a utility network using ArcGIS Runtime for .NET
//The feature server url of a feature service that has a utility network.
string url = "https://sampleserver7.arcgisonline.com/arcgis/rest/services/UtilityNetwork/NapervilleElectric/FeatureServer";
//create a utility network object and pass in the url.
var utilitynetwork = new UtilityNetwork(new Uri(url));
//This will attempt to load the utility network meta data and open the associated network sources and feature tables.
await utilitynetwork.LoadAsync();
//print the version of the utility network (2)
Console.WriteLine(utilitynetwork.Definition.SchemaVersion);
The following metadata is available for 100.6 which can be accessed through the utility network definition object. We are continuing to add additional utility network metadata to future releases of ArcGIS Runtime.
- Network sources – The backend source classes that make up the utility network. You can ask a network source for its corresponding feature table which points to a layer in the feature service. Here is a snippet showing how to get the list of network sources.
Here is a snippet of how to work with network sources using ArcGIS Runtime Swift
// Get the network definition object.
let utilityNetworkDefinition = utilityNetwork.definition
// Print the number of sources (8).
print("The utility network has \(utilityNetworkDefinition.networkSources.count) network source(s).")
// If you know the name of the class you can call networkSource(withName:).
if let structureLineClass = utilityNetworkDefinition.networkSource(withName: "Structure Line") {
print("The Structure line class name is '\(structureLineClass.name)' and has id \(structureLineClass.sourceID)")
}
// If you don't know the name of the source you can use the sourceUsageType.
// Note: you might get multiple results for certain usage types if you have more than 1 domain network.
if let structureLineClass = utilityNetworkDefinition.networkSources.first(where: { $0.sourceUsageType == .structureLine }) {
print("The Structure line class name is '\(structureLineClass.name)' and has id \(structureLineClass.sourceID)")
}
- Asset Group – This is the subtype of the network source, the asset group code and name.
Here is a snippet of how to work with asset groups using ArcGIS Runtime Qt
/* Asset Group */
//get the Aerial Support asset group
UtilityAssetGroup* aerialsupportAssetgroup = structurelineClass->assetGroup("Aerial Support");
//print the subtype code (103)
qDebug() << QString("Subtype code of %0 is %1").arg(aerialsupportAssetgroup->name(), aerialsupportAssetgroup->code());
- Asset Type – This is the discriminator for the asset group that defines an asset in the utility network. It’s at this level where the most interesting properties are set such as categories or terminal configuration.
Here is a snippet of how to work with asset types using ArcGIS Runtime Qt
/* Asset Type */
//get the first asset type that supports being a Structure.
const auto assetTypes = aerialsupportAssetgroup->assetTypes();
auto structureAssettypeIt = std::find_if(assetTypes.cbegin(), assetTypes.cend(), [](UtilityAssetType* assetType) -> bool
{
return assetType->associationRole() == UtilityAssociationRole::Structure;
});
qDebug() << QString("Asset type %0 code %1 supports being a structure").arg((*structureAssettypeIt)->name(), (*structureAssettypeIt)->code());
We also support additional properties as follows.
- TerminalConfiguration – This is the terminal configuration for a given asset group / asset type pair.
- AssociationRole – Whether this asset group/asset type supports being a container or structure or none.
- Terminal – This is the terminal object. Terminals can be used to create feature elements which can in turn be used to place starting points for tracing, set valid paths, modify associations, and much more.
- Network Attribute – This is the persisted network attribute in the network topology, used for fast access during tracing.
- Category – Categories act like tags for an asset group/tag. A category can be assigned to multiple asset group/asset type.
Connected tracing with services through starting points and barriers
For release 100.6 we have also added the ability to run connected tracing through a feature service that supports a utility network. You can place starting points, barriers and call the connected trace to have the server return the trace results as feature elements.
Here is a snippet of how to perform a connected trace on a utility network using ArcGIS Runtime for Java
/* Connected Trace */
//We want to place starting point and barrier on two well known features on naperville
// The starting point is a load side of a circuit breaker, three phase with globalId {B8493B23-B40B-4E3E-8C4B-0DD83C28D2CC}
// The barrier is on a line with globalId {95F1FD0E-CD70-4020-9CC6-FA76D21A6AC4}
//build the starting point
UtilityNetworkSource deviceClass = utilityNetworkDefinition.getNetworkSource("Electric Distribution Device");
UtilityAssetType circuitBreakerAssetType = deviceClass
.getAssetGroups().stream().filter((ag -˃ ag.getName().equals("Circuit Breaker"))).findFirst().get()
.getAssetTypes().stream().filter((at -˃ at.getName().equals("Three Phase"))).findFirst().get();
UtilityTerminal loadTerminal = circuitBreakerAssetType.getTerminalConfiguration()
.getTerminals().stream().filter((ut -˃ ut.getName().equals("Load"))).findFirst().get();
UtilityElement startingPointElement = utilityNetwork.createElement(circuitBreakerAssetType,
UUID.fromString("B8493B23-B40B-4E3E-8C4B-0DD83C28D2CC"),
loadTerminal);
//build the barrier
UtilityNetworkSource lineClass = utilityNetworkDefinition.getNetworkSource("Electric Distribution Line");
UtilityAssetType mvAssetType = lineClass
.getAssetGroups().stream().filter((ag -˃ ag.getName().equals("Medium Voltage"))).findFirst().get()
.getAssetTypes().stream().filter((at -˃ at.getName().equals("Underground Three Phase"))).findFirst().get();
UtilityElement barrierElement = utilityNetwork.createElement(mvAssetType,
UUID.fromString("95F1FD0E-CD70-4020-9CC6-FA76D21A6AC4"),
loadTerminal);
//build trace parameters
UtilityTraceParameters traceParameters = new UtilityTraceParameters(UtilityTraceType.CONNECTED, Collections.singletonList(startingPointElement));
traceParameters.getBarriers().add(barrierElement);
//run the trace and get the result
ListenableFuture<List<UtilityTraceResult˃˃ traceResultFuture = utilityNetwork.traceAsync(traceParameters);
traceResultFuture.addDoneListener(() -˃ {
try {
List<UtilityTraceResult˃ traceResults = traceResultFuture.get();
//You get a array of results since it is possible to get multiple results such as functions, elements.
UtilityElementTraceResult elementTraceResult = (UtilityElementTraceResult) traceResults.get(0);
//print the trace result (239)
System.out.println(String.format("Trace successful returned %d elements", elementTraceResult.getElements().size()));
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
});
The following series of gifs, is a demo of the connected trace authored with ArcGIS Runtime on .NET
In this animated gif, we place a starting point on a line and you can see that we detect where exactly we clicked on the line, then we place a barrier on another line and then run a connected trace.
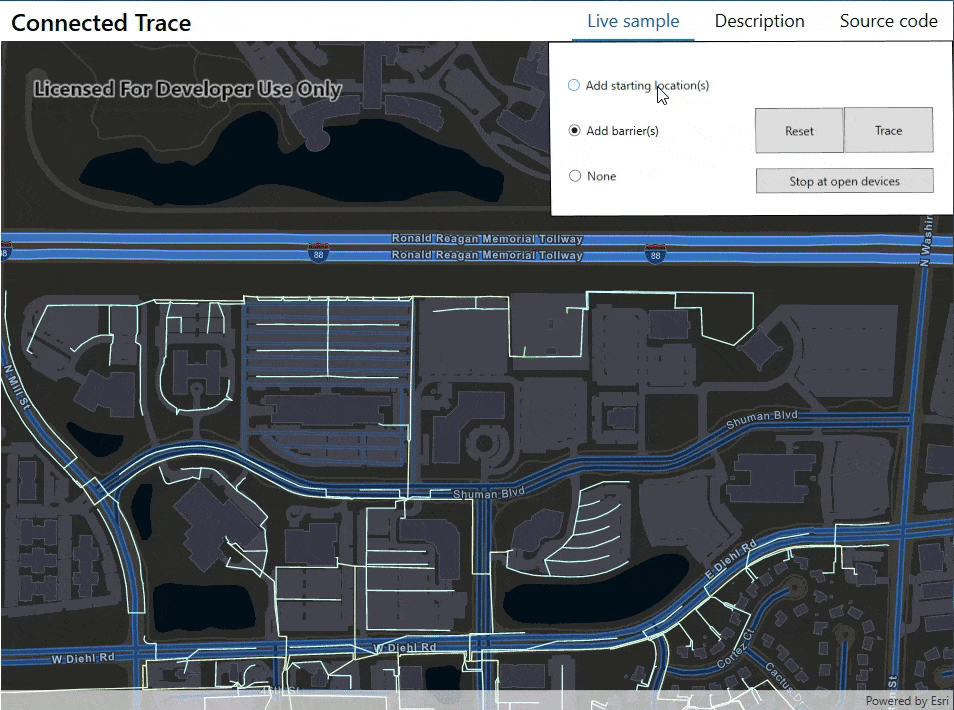
In this animated gif, we place a starting point on a device that has multiple terminals (Source and Load) and we actually place the starting point on the load side. We place a barrier on a line feature and run the trace. Notice that we are getting upstream result, this is something we will fix next.
Learn more about terminals here.
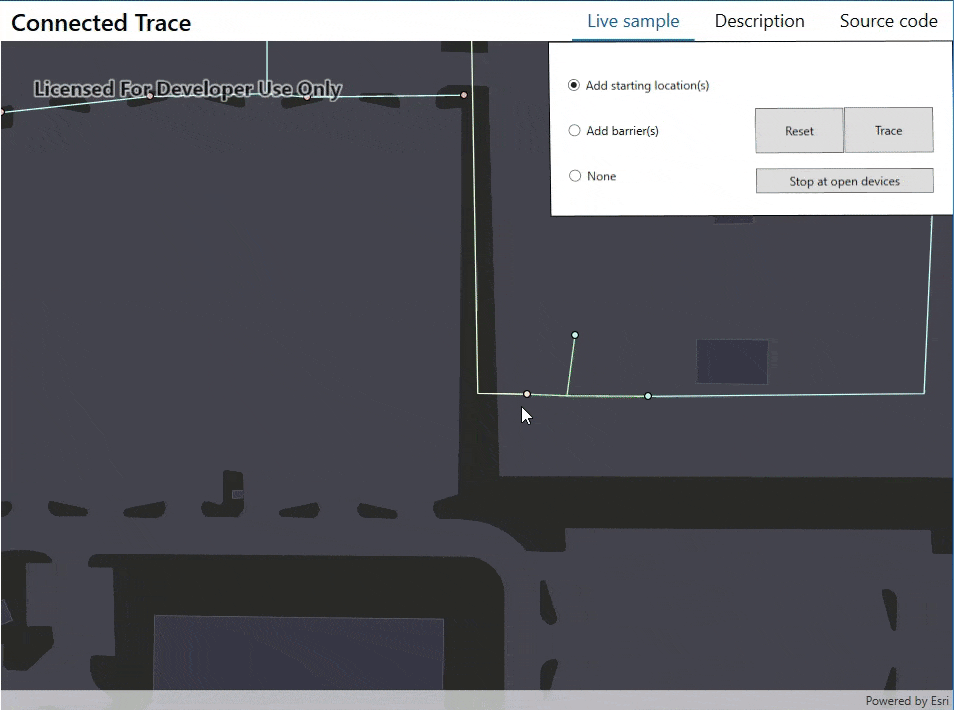
In this animated gif, we prevent upstream features from being selected by placing a barrier on the source side terminal so the trace doesn’t select the substation features.
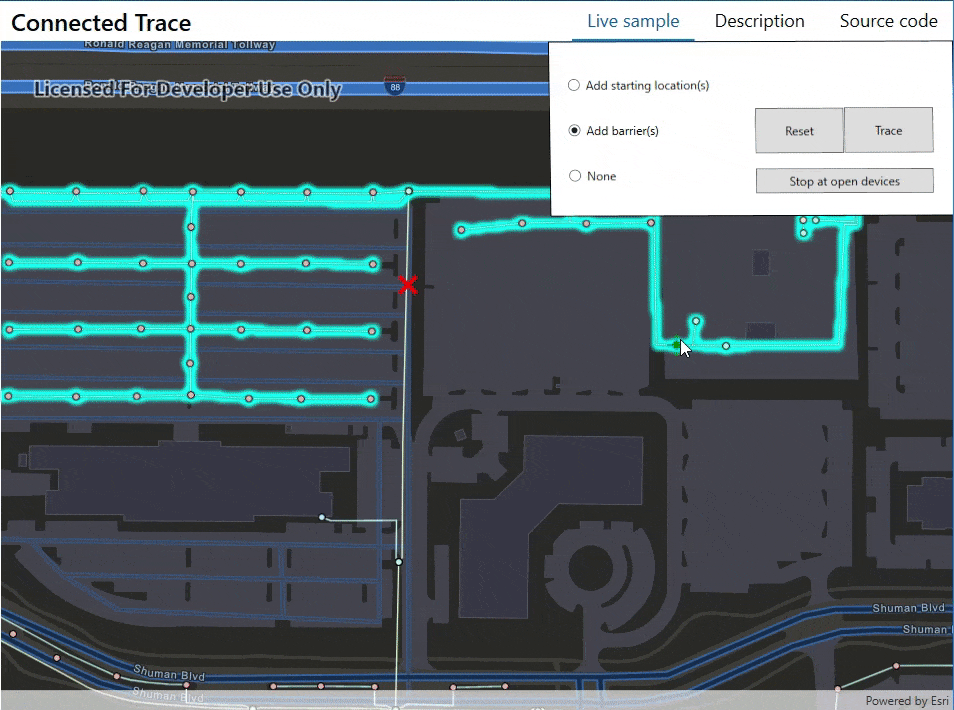
This interactive connected trace sample provides an example of usage as well.
What’s Next?
We are excited to continue bringing additional utility network functionality to ArcGIS Runtime. For upcoming near-term releases, we are planning to provide the ability to specify trace configuration such as traversability, function barriers, and propagation. We also plan to add additional metadata such as domain networks, tiers, and subnetwork management and much more.
What do you want to see next?
Article Discussion: