We are always working hard to improve the developer experience with the ArcGIS API for JavaScript. We released the API via npm and Bower so that developers could create local builds of their applications with the Dojo build tools.
Now, with the recent release of version 4.7, we are happy to announce a new webpack plugin for the ArcGIS API for JavaScript!
If you are unfamiliar, webpack is a modern web development tool for building applications. It has numerous advantages, including: module bundling, dependency graphs, and built-in code optimizations. It also has many available plugins, so chances are, if you need it, there’s a plugin for it! It’s not uncommon for JavaScript libraries or frameworks to provide their own webpack plugins, so that’s what we did.
Getting Started
You can start using the @arcgis/webpack-plugin in your project pretty easily.
npm install --save-dev @arcgis/webpack-plugin
Once the plugin is installed, you can start writing your application like you normally would. For example, maybe you want to use React to build components for your application.
import "./config";
import FeatureLayer = require("esri/layers/FeatureLayer");
import SceneView = require("esri/views/SceneView");
import WebMap = require("esri/WebMap");
import * as React from "react";
import * as ReactDOM from "react-dom";
import "./css/main.scss";
const onComponentLoad = (view: SceneView) => {
featureLayer.when(() => {
view.goTo({ target: featureLayer.fullExtent });
});
};
const featureLayer = new FeatureLayer({
id: "states",
portalItem: {
id: "b234a118ab6b4c91908a1cf677941702"
},
outFields: ["NAME", "STATE_NAME", "VACANT", "HSE_UNITS"],
title: "U.S. counties"
});
const webmap = new WebMap({
portalItem: {
id: "3ff64504498c4e9581a7a754412b6a9e"
},
layers: [featureLayer]
});
/**
* React portion of application
*/
interface ComponentProps {
webmap: WebMap;
onload: (view: SceneView) => void;
}
class WebMapComponent extends React.Component<ComponentProps, {}> {
mapDiv: any;
componentDidMount() {
const view = new SceneView({
map: this.props.webmap,
container: this.mapDiv
});
this.props.onload(view);
}
render() {
return (
<div className="webmap"
ref={
element => this.mapDiv = element
}>
</div>
);
}
}
ReactDOM.render(
<div className="main">
<WebMapComponent
webmap={webmap}
onload={onComponentLoad} />
</div>,
document.getElementById("app")
);
That’s a very simple application built with React and the ArcGIS API for JavaScript.
Now you can update your webpack configuration to use the @arcgis/webpack-plugin.
// webpack.config.js
const ArcGISPlugin = require("@arcgis/webpack-plugin");
// add it to config
module.exports = {
...
plugins: [new ArcGISPlugin()]
...
}
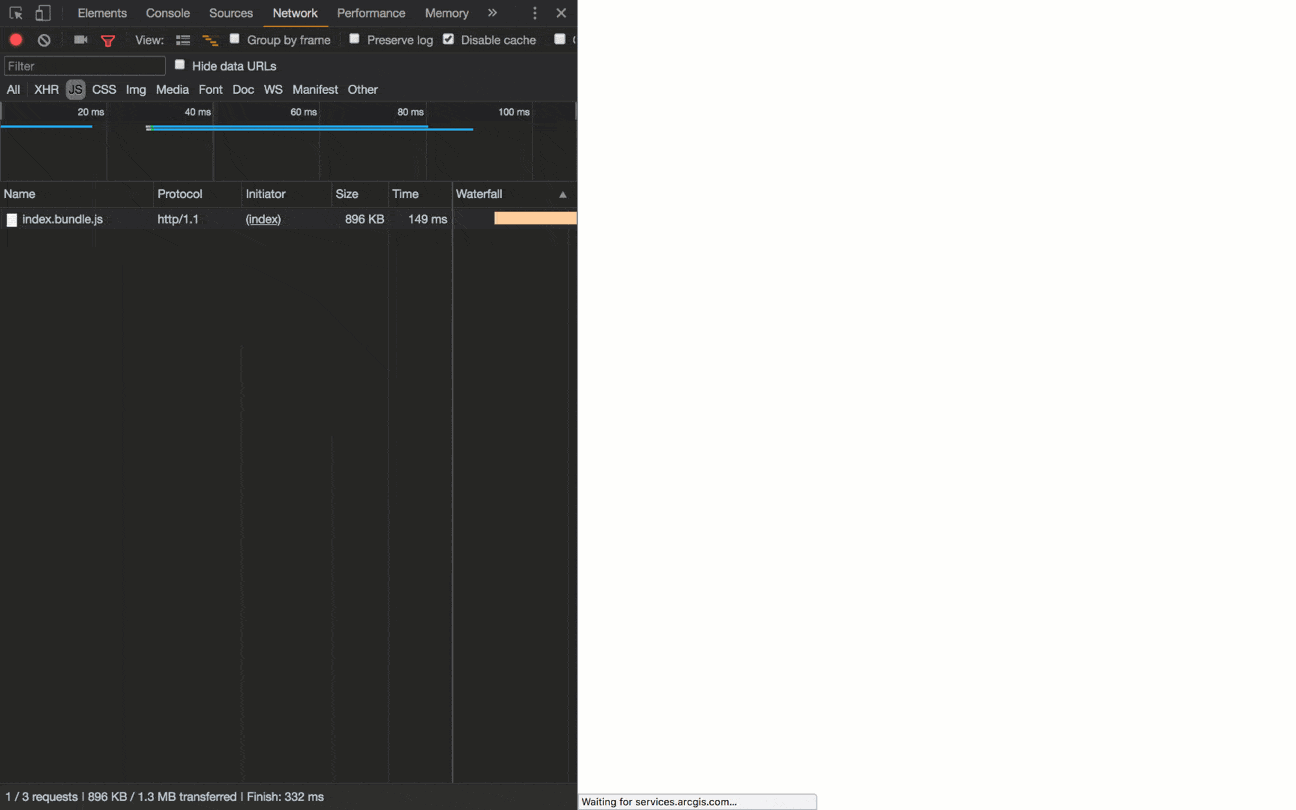
You can find the full source code for this application on GitHub. All the code splitting and bundle generation is managed by webpack. It will generate one bundle per layer, because web maps can contain any layer type, and they are loaded automatically. Your main bundle will only load the bundled files required for your application. Other bundles will be loaded as needed at runtime.
This frees you up to focus on writing your application instead of the details of building your application!
We look forward to hearing about your experience with the @arcgis/webpack-plugin, and if you have any problems, please feel free to submit an issue in the repo!
Commenting is not enabled for this article.