If you heard about ES modules and want to learn more, then this post is for you! ES modules, or ESM, work natively in the latest generation of browsers, and they make it significantly easier to build applications with third-party frameworks and local build tools. This post discusses some of the differences between AMD and ESM, there’s a hands-on tutorial to help you build your first app, then there is bonus content at the end.
Comparing AMD and ESM
If you have previously created applications using the ArcGIS API for JavaScript, then you are familiar with AMD modules and the well-known pattern of using the require() method as a wrapper for your API code. The AMD modules and ES modules provide the same API functionality, however the way they are implemented in JavaScript and used by browsers is very different.
Scaling up your applications. When building a vanilla JavaScript application using AMD, you write the code and run it immediately from a web server, there is often no additional tooling or processing involved. This is the easiest way to deploy mapping applications with the ArcGIS API for JavaScript!
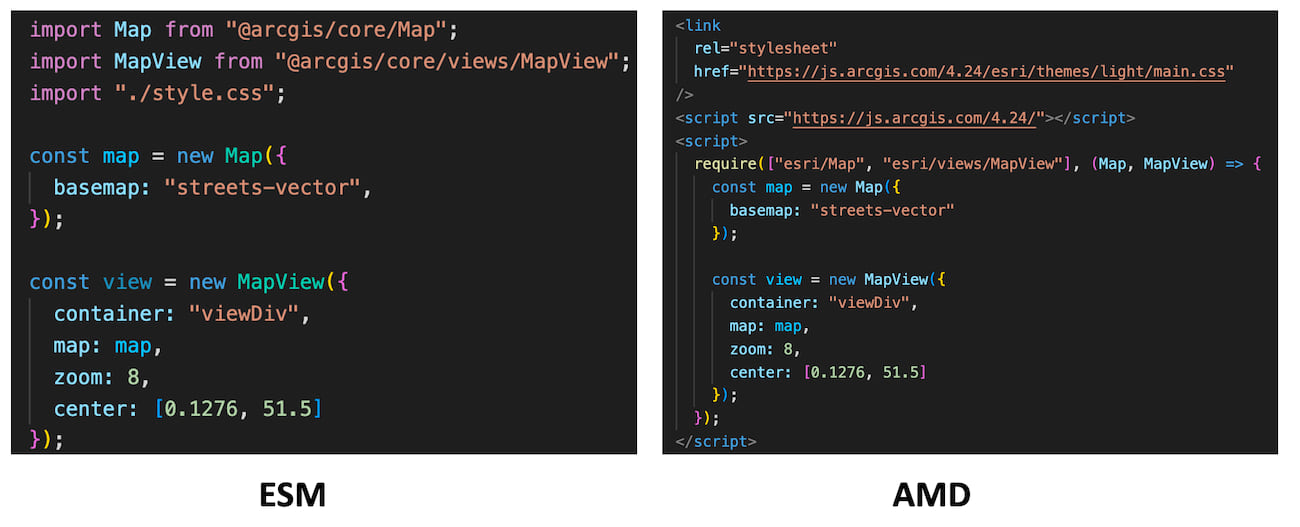
As applications grow over time and become more complex, developers can benefit from certain coding tools and automation. ES modules work directly with most modern client-side tooling to provide capabilities such as compiling, minifying, bundling, tree-shaking, linting and unit testing. This requires the use of Node.js, which lets you create modules and tools using server-side JavaScript and run them outside of the browser environment.
Node.js also comes with NPM, which is a package manager for Node.js. It includes a large online repository of code packages as well as a popular command-line interface (CLI). The most common usages of NPM include using its CLI for installing and managing project dependencies, running scripts for starting development servers, unit testing, and creating production builds. You can see NPM in action in the tutorial below.
Using packages. Packages are essentially wrappers for JavaScript libraries, and they can contain a single file or an entire directory of files that perform specific tasks. When you install a package it can retrieve and install other dependencies from the online repository, your own private repository or even from a local directory. The package for ArcGIS API for JavaScript’s ES modules is @arcgis/core, which is often simply called “ArcGIS core”.
Roll up your sleeves, it’s time for hands-on learning!
What comes next is the exciting part. Follow these steps to create your first ESM project. Go here if you want to try out a full working version of this sample.
Step 1 – Install Node.js and NPM by following the instructions here: https://docs.npmjs.com/downloading-and-installing-node-js-and-npm.
Step 2 – Create a new directory and use the NPM CLI to install Vite. It is a lightweight JavaScript framework that is easy-to-use. You can learn more about it here: https://vitejs.dev/guide/.
npm create vite@latest
Follow all the prompts. For example, assign your project a name, then choose “vanilla” framework and “vanilla” variant. Proceed to the next step once you are done with each prompt.
Step 3 – Replace the entire index.html file with the code below, or simply replace the <div> as described in the code comments.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Vite App</title>
</head>
<body>
<!-- Replace the <div> below -->
<div id="viewDiv"></div>
<script type="module" src="/main.js"></script>
</body>
</html>
Step 4 – Overwrite the entire contents of styles.css:
@import 'https://js.arcgis.com/4.24/@arcgis/core/assets/esri/themes/light/main.css';
html,
body,
#viewDiv {
padding: 0;
margin: 0;
height: 100%;
width: 100%;
}
Step 5 – Install the @arcgis/core modules:
npm i @arcgis/core@latest
Step 6 – Overwrite the entire contents of main.js. This .js file is an ES module:
import Map from "@arcgis/core/Map";
import MapView from "@arcgis/core/views/MapView";
import "./style.css";
const map = new Map({
basemap: "streets-vector",
});
const view = new MapView({
container: "viewDiv",
map: map,
zoom: 8,
center: [0.1276, 51.5],
});
Step 7 – Run the application in “developer” mode.
npm run dev
Congratulations, you just built your first ES module application! There is still so much more to learn, so keep reading for some insight into the history of ES modules.
TL;DR – Bonus Content
How do I deploy an ESM app?
Client-side tooling provides capabilities for creating production builds. For example, with the tutorial above you can run this command to output a production build to the dist
directory:
npm run build
This is an example where manual tasks are replaced by automation. Running the build command starts a series of precise and easily repeatable workflows to tree-shake, bundle and optimize your application code.
What is an ES module?
ES modules were officially announced in 2015 as part of the JavaScript language specification called ECMAScript 2015. That specification is sometimes referred to as the 6th edition of the JavaScript standard, “ES2015” or simply “ES6”.
ES6 was the beginning of a fundamental change in JavaScript functionality by introducing new syntax for organizing JavaScript logic into modules and class declarations that would be natively supported by web browsers. The standard was, in fact, adopted by all major browsers, and it has gone through multiple updates since then. For example, ES2021 was published in June 2021 and is the 12th edition of the language specification.
Why do ES modules matter?
The latest generation of browsers, popular frameworks and build tools expect applications to use ES modules, they work directly in browser JavaScript engines without needing a separate loader library or additional pre-processing.
Before the release of ES6, browsers did not have a standardized JavaScript module system. Various individuals and groups who wanted to use modular programming patterns in JavaScript ended up eventually creating their own systems; for example, Dojo Toolkit released their first AMD version in 2005! Another example is CommonJS which was developed at Mozilla in 2009 and then adopted for use by Node.js.
The challenge for these original module systems is they are based on an API specification rather than an ECMA standard, and they haven’t been adopted as native JavaScript functionality by the major browsers. For that reason, running AMD in a web application requires a separate module loader library, such as Dojo or RequireJS, to help browsers understand the module wrapper syntax used by require() and define() statements.
Do I need to use ES modules with a framework?
No. Since ESM is natively supported in JavaScript by all major browsers, you can use them to build custom applications without a framework such as React, Angular or VueJS.
However, when integrating libraries such as @arcgis/core you will need a module bundler (e.g. webpack or rollup) and possibly a transformer (e.g. babel) to provide capabilities like bundling, tree-shaking, minifying, backwards compatibility and more.
Can I use the API’s ES modules in a browser, without needing to build locally?
There is an ArcGIS ESM CDN for the ArcGIS API for JavaScript, however it is designed for prototyping-only and should not be used in a production application. The build is not optimized for performance and will potentially generate hundreds of additional HTTP requests when loading dependencies.
What’s next?
We encourage you to keep learning and try out the variety of ready-to-install ESM samples here: https://github.com/Esri/jsapi-resources/tree/master/esm-samples. These samples are a great way to explore the basic about using the API with different frameworks and build tools. To gain more insights on how ES modules work, there is an excellent MDN article that goes deep into the key aspects of integrating modules into your applications.
Article Discussion: