The ArcGIS API for JavaScript is a powerful library that you can use to build applications that leverage the power of the ArcGIS Platform. While you can use the ArcGIS API for JavaScript on its own to build compelling web mapping applications, some developers choose to integrate it with other JavaScript libraries and frameworks.
Svelte is a popular open source JavaScript library that is used to build user interfaces. Svelte makes it easy to reactively bind (connect) variables and properties between your JavaScript code and the UI. Behind the scenes, Svelte uses a compile step to convert the Svelte JavaScript code into highly optimized vanilla JavaScript code for your application, which yields great UI performance.
As a developer, Svelte is enjoyable to use. The reactivity, stores, and other features of Svelte allow you to quickly and elegantly build simple or advanced web apps. In the past few years Svelte has been ranked very highly for developer satisfaction in the Stack Overflow and State of JS surveys.
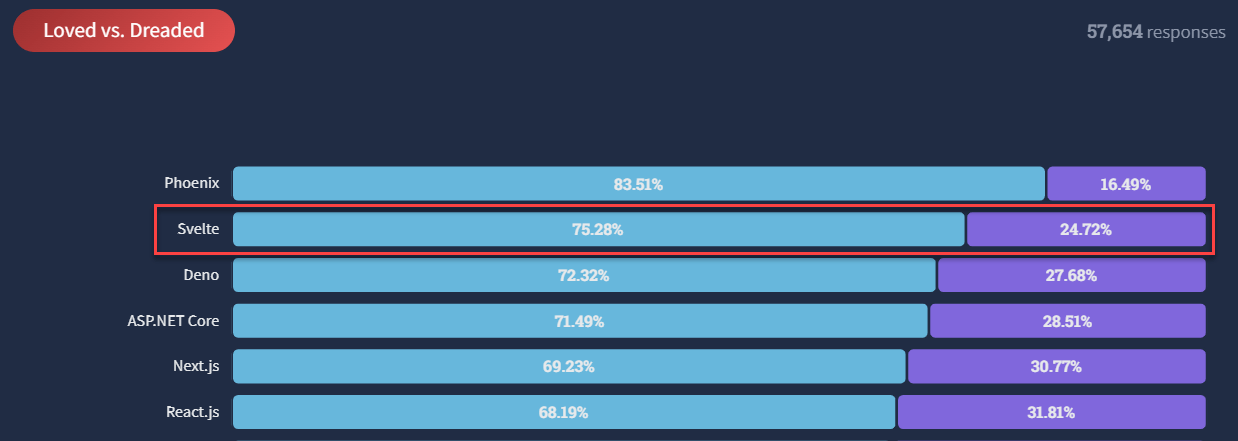
This blog post is a brief overview on how you can get started using Svelte with the ArcGIS API for JavaScript.
Getting Started
You can view the source code for the application we’re building on GitHub.
First, we’ll set up a Svelte application. We’ll use Vite, a popular JavaScript bundler, to get going quickly. Ensure you’ve installed the latest LTS version of Node.js, then use NPM (which comes with Node.js) to create a project. In the terminal (command line), browse to an empty folder and run:
npm init vite@latest
This process will ask you a few questions:
- Do you want to install the vite package? Yes.
- Project name: use any name you’d like
- Select a framework: choose “svelte”
- Select a variant: choose “svelte”
Then, in your terminal, browse to the new folder (named after the project name you choose). Then run:
npm install
npm run dev
This will run a local web server. You can navigate your browser to the URL provided in your terminal (probably http://localhost:3000). Open the folder in your code editor of choice. You can view the code that was generated by the template. Open the src/App.svelte
file and make a change to some of the text. The browser automatically refreshes, and you can see the change reflected on the page.
Adding a map
Now that the Svelte app is running, we’ll add a map to the web application. In the terminal, stop the development web server temporarily (Ctrl+c). Then install the ArcGIS API for JavaScript:
npm install @arcgis/core --save-dev
Then start the web server again (npm run dev
).
In App.svelte
, remove all the JavaScript code, HTML markup, and style rules.
Then in the <script>
tag section, import the MapView module from the ArcGIS API for JavaScript and import the necessary CSS.
<script>
// ADD these 2 lines:
import MapView from "@arcgis/core/views/MapView";
import "@arcgis/core/assets/esri/themes/light/main.css";
</script>
<main></main>
<style></style>
Then add a single <div>
, into which the map will be placed. Within that <div>
tag, add a Svelte use directive that will call a function when the DOM node is ready:
<main>
<!-- ADD this line: -->
<div class="view" use:createMap></div>
</main>
Then create a function with that name (createMap
in this case) in the JavaScript section of the code that will create the map with the DOM Node that Svelte provides:
<script>
import MapView from "@arcgis/core/views/MapView";
import "@arcgis/core/assets/esri/themes/light/main.css";
// ADD this function:
const createMap = (domNode) => {
const view = new MapView({
container: domNode,
map: {
basemap: "streets-vector",
},
zoom: 15,
center: [-90.1928, 38.6226], // longitude, latitude
});
};
</script>
Finally, add some styles to define the size of the map:
<style>
/* ADD this section: */
.view {
height: 400px;
width: 800px;
}
</style>
We now have an interactive map using the ArcGIS API for JavaScript!
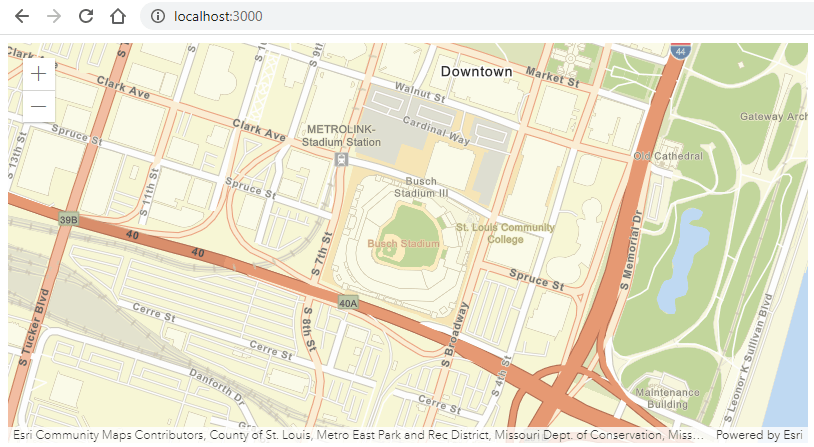
Using Svelte reactivity
Outside of the map, our application will contain some text in the page that shows the current latitude and longitude for the center of the map. The text should update as the map changes. To keep that text updated, we will use Svelte binding.
First, create a local JavaScript variable to hold the text we want to display:
<script>
import MapView from "@arcgis/core/views/MapView";
import "@arcgis/core/assets/esri/themes/light/main.css";
// ADD this line:
let centerText;
Then in the createMap
function, add some code to watch the center
property of the map and update that variable when it changes:

Finally, use a Svelte if block and the basic Svelte display markup (variable within curly brackets) to display that variable:
<main>
<div class="view" use:createMap></div>
<!-- ADD these 3 lines: -->
{#if centerText}
<p>{centerText}</p>
{/if}
</main>
Summary
In this post, we’ve built a basic interactive map that uses Svelte reactivity to dynamically display the center latitude/longitude of the map. That reactivity is one of the core features of Svelte. Svelte includes even more functionality, like Stores, component events, motion helpers, and transition helpers. You can learn more about these features in the Svelte tutorial. Using these powerful features in Svelte makes web app development even more fun and efficient. Try Svelte out today!
Article Discussion: