Want to know a Swift Maps SDK secret? The Swift Maps SDK supports showing Apple’s SF Symbols® on maps! Read on to learn the benefits of incorporating SF Symbols into apps built with the Swift Maps SDK by looking at what SF Symbols are, how to improve your iOS development experience, and how you can build visually beautiful iOS apps.
Maps and symbols go together like mac and cheese – they’re a perfect combination and enhance each other’s properties. Symbology plays an important role in GIS as symbols can express meanings through images, color, and size. Most, if not all, maps will incorporate symbology in some way.
The most basic symbols you may see are dots to represent cities and stars to emphasize capitals. Consider how your local weather presenter might present the forecast. They stand in front of a map with images of clouds or suns, and you instantly know what kind of weather to expect for the day. Symbols convey messages that can bypass any language barriers.
With the Swift Maps SDK, it’s easy to display maps containing symbol-rich cartography authored using e.g. ArcGIS Pro desktop app or an ArcGIS Map Viewer web app. But sometimes, you want to programmatically add additional custom symbols to the map on your app or change the original symbology of a data layer on the map. You can do this easily with the Swift Maps SDK, let’s look at how using Apple’s SF Symbols as an example.
What are SF Symbols?
The Swift Maps SDK offers a number of predefined simple 2D and 3D symbols (e.g. circles, diamonds, cubes, and more), as well as support for advanced symbols using the multilayer symbols API or style files (.stylx) created from ArcGIS Pro. To take your customization even further, you can import your own images as e.g. JPEG, PNG format, and create symbols from them using the picture marker symbol API. There are endless possibilities, but the hardest part about symbology is curating symbols that look good and fit your needs. An easy approach when developing iOS applications is to use Apple’s SF Symbols which are compatible with the Swift Maps SDK.
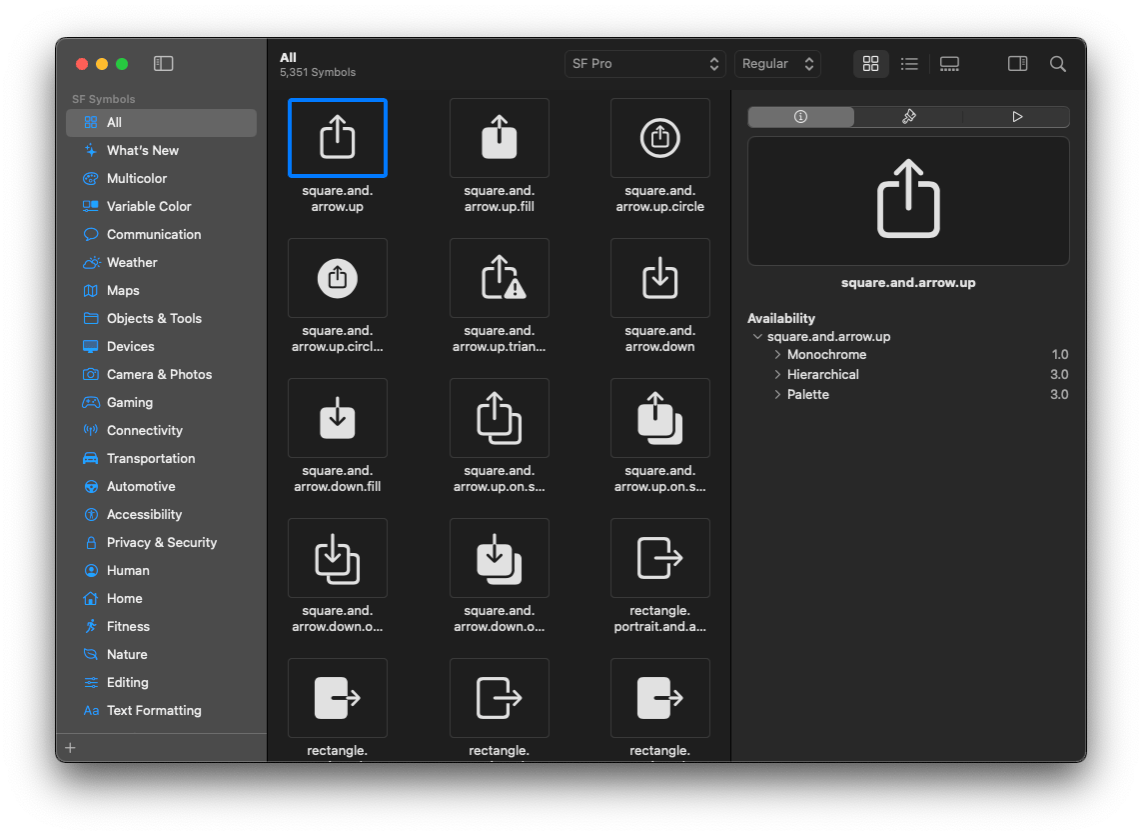
SF Symbols are vector-based images created for iOS apps, provided to developers by Apple. The “SF” in SF Symbols refers to the San Francisco system font used by Apple platforms. In Apple’s words, SF Symbols are “a library of iconography designed to integrate seamlessly with San Francisco.” The symbols are customizable in color, size, and weight. Developers are encouraged to use these in their iOS apps. You can download the SF Symbols app to browse the thousands of symbols that are offered.
Note: the library does contain copyrighted symbols and there are limitations on their use – check the SF Symbols documentation for more information.
How do SF Symbols benefit developers?


Symbols are used to indicate an action or to convey a message. You can find good examples of SF Symbols in any iOS app built by Apple. The Apple News app is a good example of using SF Symbols in a toolbar alongside text to indicate the active tab in an app. In some cases, text isn’t even necessary. For example, users can infer what action a trash can symbol represents. Additionally, SF Symbols vary in color and size. Adjusting the colors is easy using the system colors. And because SF Symbols are vector-based, the images can scale without losing quality.
You can also use symbols to represent a large data set. Thinking again of our local weather forecast, clouds and suns symbolize the weather data. Because we may use a variety of symbols in one app, it is important that the symbols have a consistent theme or font. Utilizing SF Symbols can make your apps look more cohesive.
Speaking of cohesion, iOS developers know how important it is to incorporate a consistent, clean Apple “look” to their apps. Apple is known for upholding a certain “look” to all their products and encourages their developers do the same. Apps that adhere to the same standards exude a sense of professionalism and blend seamlessly with the iOS environment. Incorporating SF Symbols in your apps can help you achieve that Apple look with minimal effort.
How to add SF Symbols to your Swift Maps SDK app
We’re ready to symbolize! Let’s create a Swift Maps SDK app that shows the start of a hiking trail in Malibu, California, using the Display a map tutorial app. This app simply displays a map with an existing basemap style and using this project as a starting point, all we need to do is add a single symbol on top of the basemap.
Step 1: Add a GraphicsOverlay
Symbols are only attributes of graphics or renderers. To display symbols, the first step is to add a graphics overlay. A graphics overlay allows you to add graphics to a layer which is then overlaid on top of the map.
// `model` is an ObservableObject that contains a GraphicsOverlay.
MapView(map: map, graphicsOverlays: [model.graphicsOverlay])
Step 2: Create a UIImage from an SFSymbol
Choose an SF Symbol from the library that is suitable for your needs; in this case, we’ve selected the “figure.walk.circle.fill” symbol. Once you’ve selected an SF Symbol, create a UIImage using its system name. The name must match exactly as it appears in the SF Symbol app, so the compiler knows which symbol to use.
let image = UIImage(systemName: "figure.walk.circle.fill")!
Step 3: Create a PictureMarkerSymbol
Next, create a PictureMarkerSymbol using the UIImage. The PictureMarkerSymbol class uses an image to symbolize graphics and features that have point or multipoint geometry. Note: PictureMarkerSymbols do not support GIFs nor can they detect theme changes; therefore, SF Symbols cannot be animated and changing the app’s light and dark appearance will have no effect.
let symbol = PictureMarkerSymbol(image: image)
Step 4: Create a point
Create a point to use as the location for the symbol.
let point = Point(x: -118.806546, y: 34.020933, spatialReference: .wgs84)
Step 5: Create a graphic
Create a graphic using the point and PictureMarkerSymbol.
let graphic = Graphic(geometry: point, symbol: symbol)
Step 6: Add the graphic
Lastly, add the Graphic to the graphics overlay. Now we know where to go for a fun hike in Malibu. How easy was that?
graphicsOverlay.addGraphic(graphic)
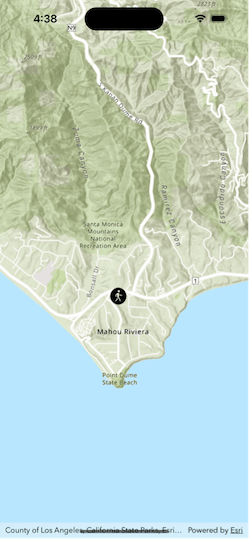
Adding SF Symbols to a dataset
There is of course more than one hiking trail in Malibu. Let’s try adding multiple symbols at once to multiple points, using a data layer. A feature layer contains a dataset that can be visualized by a renderer which contains a set of symbols. This feature layer contains data of multiple trailheads in Malibu, California. Plain circles on a map would do the job but using the “figure.walk.circle.fill” SF Symbol helps convey the message that these points are trailheads. The Add a feature layer tutorial app displays the Malibu trailhead feature layer on top of a map. Using this app as a starting point, all we need to do is add a renderer.
Step 1: Create a UIImage
To create a feature layer renderer, make a UIImage of the SF Symbol you want to display. We’re still using the “figure.walk.circle.fill” SF Symbol.
let image = UIImage(systemName: "figure.walk.circle.fill")!
Step 2: Create a PictureMarkerSymbol
Using that image, create a PictureMarkerSymbol.
let symbol = PictureMarkerSymbol(image: image)
Step 3: Create a SimpleRenderer
Lastly, create a SimpleRenderer with that symbol and set it to the feature layer’s renderer property. That’s it! Creating multiple SF Symbols is just as easy as making just one symbol! Notice below the difference between using a default circle and an SF Symbol to indicate the trailheads.
featureLayer.renderer = SimpleRenderer(symbol: symbol)
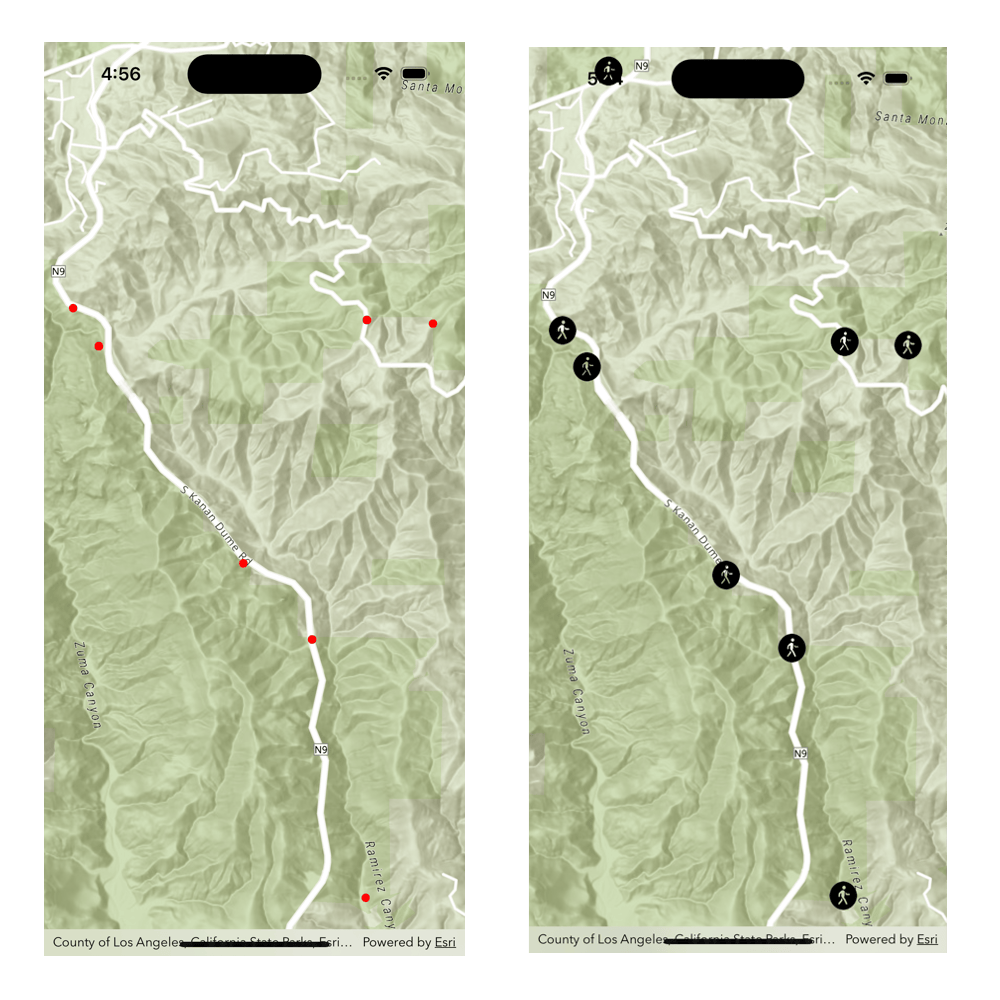
Customizing SF Symbols
What happens if you don’t like the default style of SF Symbols? Just as you can customize the default Swift Maps SDK symbols, you can easily customize SF Symbols to your preference!
Step 1: Preview
Open the SF Symbols app and ensure the Rendering Inspector panel is visible. Select your symbol and use the Rendering Inspector to preview different colors and rendering modes. We’ve changed the palette rendering mode and used purple and cyan.
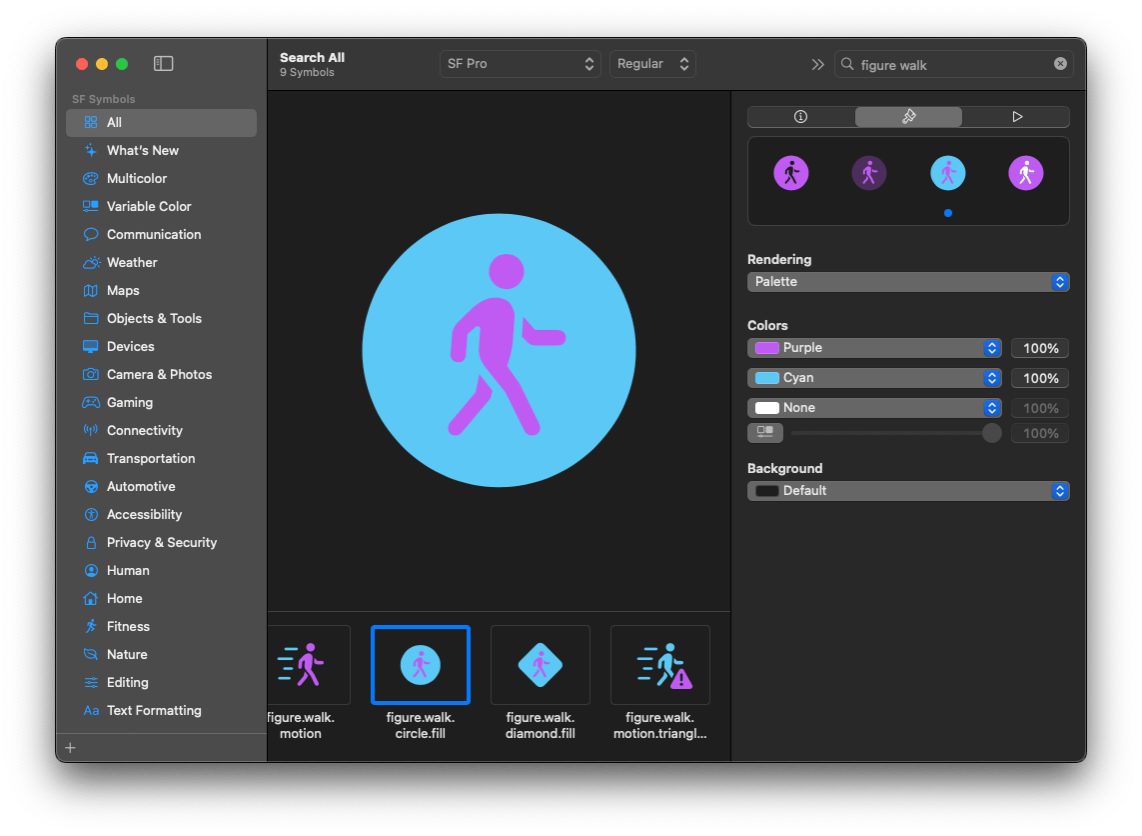
Step 2: Make a configuration
Once you’ve customized the symbol to your liking, take it to the code. Symbol configuration is an object that contains the styling information that will be applied to the UIImage. Create a symbol configuration based off the settings in preview.
var config = UIImage.SymbolConfiguration(paletteColors: [.systemPurple, .systemCyan])
config = config.applying(UIImage.SymbolConfiguration(font: .systemFont(ofSize: 30.0)))
Step 3: Apply the configuration
Create an image with the configuration or apply the configuration to an existing image. There you have it, a quick and simple way to stylize SF Symbols which you can then display in your Swift Maps SDK app!
// Creates the image with the configuration.
let imageWithConfig = UIImage(systemName: "figure.walk.circle.fill", withConfiguration: config)!
// Applies the symbol to the configuration after.
let appliedConfigImage = image.applyingSymbolConfiguration(config)!
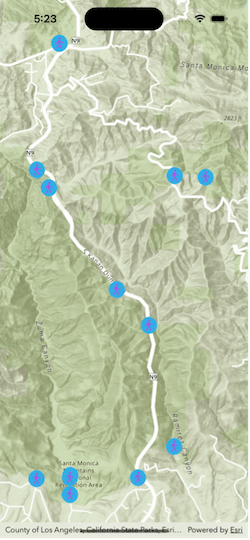
Summary
The use of symbols in your Swift Maps SDK apps provide meaningful context and messaging to your users. By including SF Symbols (tools provided by Apple) in your app, you can use them to indicate actions or convey messages alongside text or just by themselves. The ArcGIS Maps SDK for Swift makes it easy to add customized symbols to your app by utilizing the PictureMarkerSymbol and Renderer classes. Doing so can save you time and help you create an app that users will be drawn to.
You can check out the code on my GitHub to review the examples in this blog in depth. Once you’re ready to try it out for yourself, you can create a developer account for free and download ArcGIS Maps SDK for Swift and get started with our tutorials and documentation. For more help with styling a feature layer, check out the tutorial!
Credit Notice: SF Symbols is a registered trademark of Apple Inc.
Article Discussion: