This ArcGIS Pro Python tutorial is the fourth installment in a series introducing Python in ArcGIS Pro. It assumes you’re a comfortable GIS user, but have no programming experience. Start at the first post in the series to get the full introduction, or, if you’re just here for the tutorial, see post three to start at the beginning, and return here to finish.
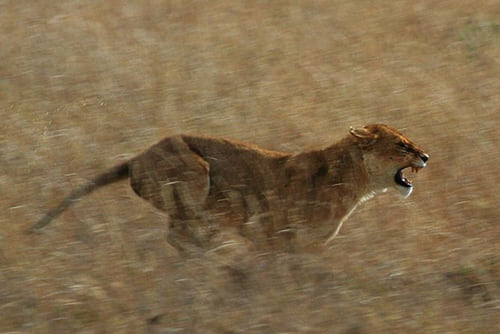
Meet your IDE
As mentioned in the previous post, you’ll likely use two different Python environments for your ArcGIS Pro work. The first is the Python window. This is a good place to test bits of code and see immediate results on a map. You can write entire scripts in the Python window, but you’ll likely find this easier to do outside of ArcGIS Pro in an IDE.
An IDE, or integrated development environment, is an application that provides an environment in which to write, debug, and run code. IDEs flag potential errors, allow you to run code one line at a time, and generally offer a more robust environment for creating scripts.
In this part of the tutorial, you’ll create a script in the PyCharm IDE to extract information from a feature class without opening it in Pro. Before you begin, download Pycharm. Choose the free Community version and follow the setup prompts.
A Python 3 interpreter (a program that reads and executes Python code) was installed on your machine when you downloaded ArcGIS Pro, but in order to access it, you first need to point PyCharm to the location. You’ll do that by configuring the interpreter settings.
- Open PyCharm (if it didn’t open automatically after the download, go to the Windows Start menu. In your list of programs, looks for JetBrains PyCharm Community Edition). Several pop-up windows may appear. Accept default settings and agreements as necessary until you see the Welcome screen.
- Click Configure and then Settings (you can also access settings from the File menu within PyCharm).
- The Settings window will open to the Appearance tab. I’ve used the IntelliJ theme to capture screenshots for this blog; you can optionally change your theme here to match mine. Select the theme from the drop-down list and click Apply.
- Click Project Interpreter from the list at left.
- In the top-right corner of the page, click the gear-shaped Configure button and then Add.
- In the pop-up window, click the System Interpreter tab, and click Browse (…) next to the default path.
- Navigate to C:\Program Files\ArcGIS\Pro\bin\Python\envs\arcgispro-py3 and choose python.exe. Click OK three times, or until you’re back at the Welcome screen. It will take a few minutes for the interpreter to update.
Now, back to where we left off in our story…
The species that roam our streets (rogue zoo animals, continued)
You’re a GIS analyst for the city of Redlands, where it’s been a hectic morning. Last night, a bunch of animals were released from the zoo, and today the authorities are scrambling to ensure public safety. You’ve been working in ArcGIS Pro using the Python window to identify schools within 1 kilometer of reported animal sightings. Just as you’re backing up your work to a Python file and brainstorming how you could refine your new script, there’s a knock on your office door. Unfortunately, it’s not the local press dropping by to ask you about how you’ve harnessed the power of GIS to help save children from wild zoo animals. It’s your boss, who wants you to work on something different now. Animal control is requesting a list of the species sighted by one of the field workers, Jimmy, who is not responding to calls.
Since you just looked at the attribute table for Jimmy’s field sightings in ArcGIS Pro, you know the three species of animals he saw, and you relay that information. ‘But what if they come back later in the day and want information about another agent?’ you wonder. ‘Or if the next dataset Jimmy sends in is longer and hard to skim through visually?’
This calls for a script. This time, you’ll do your work outside of ArcGIS Pro, in the PyCharm IDE. This way, you’ll be able to edit it later with ease, and since the results you need will be text, it’s not important to look at a map.
- Create a new folder for your zoo-related Python scripts in a convenient location, such as your C drive, and name it PythonZoo.
- Return to the PyCharm welcome screen, if it’s still open, or open PyCharm again.
- Click Open (if you’re on a window other than the Welcome screen, click File > Open). Browse to and select your PythonZoo folder and click OK.
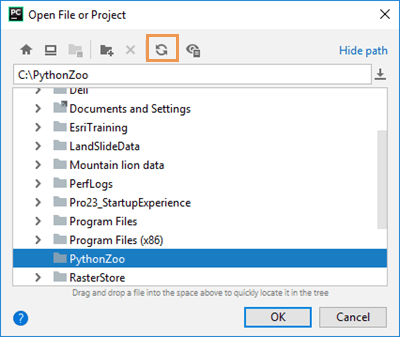
A PythonZoo project opens in PyCharm. Like ArcGIS Pro, PyCharm is a project-based work environment. When you open an empty folder, PyCharm automatically adds new configuration files to turn the folder into a Python project. Now, you can start adding scripts to the project.
- From the Project folder tree at left, under Project, right-click PythonZoo, hover over New, and choose Python file. Name the file SpeciesID and click OK.
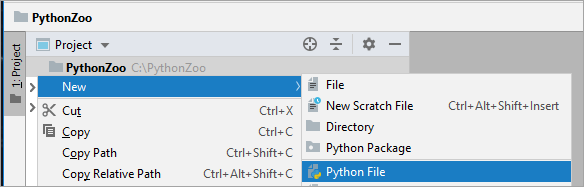
A new editor window opens. Now, you’re working in the SpeciesID.py file within the PythonZoo project. Python scripts have a .py extension, but they are essentially just text files with a special extension that tells the Python interpreter what they are.
You’ll start your script by importing the arcpy module. A module is a collection of related Python functions, classes, and objects you use to accomplish a certain type of task: in the case of ArcPy, scripting for ArcGIS Pro.
The ArcPy module is automatically imported when you work in ArcGIS Pro, but not in PyCharm. ArcPy is one of thousands of Python modules in existence. If all of these were automatically imported, even the simplest script would take a very, very long time to run. That’s why most modules must be explicitly imported to each script.
- Click inside the editor window. Type
import arcpy
and press Enter.
Next, you’ll add a second module, NumPy. While ArcPy provides most of the ArcGIS Pro-tailored functionality you need, you can combine this functionality with other modules to diversify your options. In this script, you’ll use NumPy to create a list of unique values (i.e., a comprehensive list of species) from Jimmy’s field sighting data.
- Type
import numpy
and press Enter.
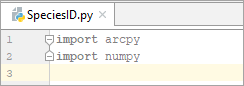
Next, you’ll set the ArcPy environment. The environment is the location on your computer where the Python interpreter will look for referenced files and store outputs by default. In this case, it’s the ZooEscape geodatabase. When you were working in the ZooEscape project in ArcGIS Pro, you didn’t have to specify that, but in PyCharm, you do.
- Press Enter again to skip a line (leaving blank lines makes your code more readable). Then on line 4 of the script, type
arcpy.env.workspace = r“”
You’ll paste your local path to the ZooEscape geodatabase between the quotation marks. There are several ways to find it; you’ll copy it from the geodatabase settings in ArcGIS Pro.
- Open the ZooEscape project you used in the last tutorial in ArcGIS Pro. In the Catalog, right-click ZooEscape.gdb and click Properties. In the Database Properties window, copy the full path from the Name box. Close ArcGIS Pro.
- Return to PyCharm and paste the path between the quotation marks. At the end of the line, press Enter.

A couple of things have happened. The import statement for ArcPy is colored in because you’ve called (used) the module. Depending on the length of your path, you may also notice a grey underline beneath it. When you hover over it, a screentip appears telling you the line is too long because it’s over 120 characters. This is a non-critical suggestion, and won’t prevent your script from running. If the underline were red, it would signify a potentially critical problem.
Before each step of your script, you’ll make a note using commented pseudocode. Pseudocode has two purposes: it guides your work as you code, and it tells anyone who reads your code later what each section is doing. You’ll use the # sign at the beginning of each pseudocode line so that the interpreter will ignore it, but you can read it.
- Press Enter to arrive at line 6 and type –
# Create a new function which returns a list of species by identifying unique values in the attribute table.

Now, you’ll create a new function. In the previous tutorial, all the functions you used were already made for you. However, it’s also a common practice to make your own functions to fulfill your specific needs. Here, you’ll create a function that takes the name of an attribute table and one of its fields as inputs and returns a list of unique values as the output.
- On line 7, type the following and press Enter-
def getSpecies(table,field):
The def
keyword signifies that you’re defining a function. It’s followed by the name of the function and the parameters, or arguments, that it requires. When you press Enter, the next line is automatically indented. This is where you’ll write the inner workings of the function, or, in other words, what will happen behind the scenes every time you call getSpecies.
- On line 8, type the following and press Enter-
data = arcpy.da.TableToNumPyArray(table, [field])
Here, you’re converting the attribute table to a Python class NumPy can use, called an array, and assigning that array to the variable data
. You’re embedding a function from ArcPy within your own, new function. The information needed to use this ArcPy function came from its help page.
Now, you’ll use the return
keyword to give your function an output – a list of unique species.
- On line 9, type the following and press Enter-
return numpy.unique(data[field]).tolist()
Now that you’ve created a NumPy array (data
) which contains the information you need (field
), you can use the unique function to find unique values. This function automatically returns an array, but adding .tolist() converts it to a list, a more common data type.

- Your function is done. Press Enter to create a blank line on line 10. Next, you’ll call the new function.
- On line 11, begin by typing the following pseudocode explanation and press Enter-
# Call the new function and assign the output to a variable.
- On line 12, type the following and press Enter-
speciesList = getSpecies("FieldSightings_Jimmy", "Species")
You can now use getSpecies
as you would any other built-in function. You simply fill in the required arguments (the name of a table followed by the name of a field it contains). Here, you’re assigning the output to a new variable, speciesList
, which can be printed.

- Press Enter to leave line 13 blank. On line 14, type your last pseudocode line and press Enter-
# Print the variable containing the list of species.
- On line 15 type the following and press Enter-
print(speciesList)
Your script is now ready to run.
- From the Run menu, click Run… On the Run dialog that appears, click SpeciesID.
The Run window appears beneath the Editor. After a few moments, a list of three species will appear, followed by the text Process finished with exit code 0, meaning the script ran successfully.

If you didn’t get the expected results, check your work against this code snippet (you’ll still have to fill in the path to your local geodatabase, though):
- You can now close PyCharm; your changes were automatically saved.
You now have a short script in your arsenal that automates a task: identifying the species of zoo animals that Jimmy saw. It’s a simple task, yes, but one that could scale. Imagine if you wanted to extract unique values from an attribute table that was thousands of records long, or if you had dozens of datasets. By changing the inputs or adding some loops to this script, you could handle either of those situations quickly. That’s the power of automation – in the real word just as much as in the fictional universe where the Redlands Zoo exists.
Back to reality
That’s it for your whirlwind tour of the Python window and an IDE. This is just a small example of what you can accomplish with these tools and is by no means comprehensive. But my hope is that this blog mini-series has gotten you excited to practice, gain a thorough understanding of these concepts, and then go beyond them.
If you thought this exercise was fun, I encourage you to come up with an equally bogus scenario and try to write a script around it. Remember, I just started learning Python recently myself, and I was able to put this tutorial together using the resources I outline in a previous post. If I can do it, so can you!
Commenting is not enabled for this article.