If you’re creating web applications with ArcGIS API for JavaScript then you probably ran into those situations where you couldn’t remember how to set that UniqueValueRenderer. So you went to the documentation, copy pasted the example code and then customized it for your data. In this blog post we want to show you a workflow where you make use of code snippets to avoid having to leave the editor and also to write less code (we created a plugin with code snippets for Visual Studio Code, but you can actually create your own code snippets for any other editor).
Another workflow where code snippets come in handy, is when debugging. Besides setting breakpoints and pausing on exceptions (we mainly use Google Chrome for debugging), you can also get access to the view, navigate the layers in your map, get the camera position in 3D or figure out when everything was loaded with just a few code snippets in the console.
So let’s get started!
Development code snippets
These are the code snippets that you use during development, representing boilerplate code, a verbose syntax that you can never remember, or maybe just a simple syntax that you have to repeat over and over again in your code (ok, that might be subject to some refactoring if you have to repeat it that often…).
So let me show you an example. Something I can never remember is the code for adding labels in 3D. Instead of having to memorize all the properties that I need to set on the LabelClass and then type them, I can just type a prefix like labeling3d
, press Tab
and the code snippet will show up with placeholders for my custom values:
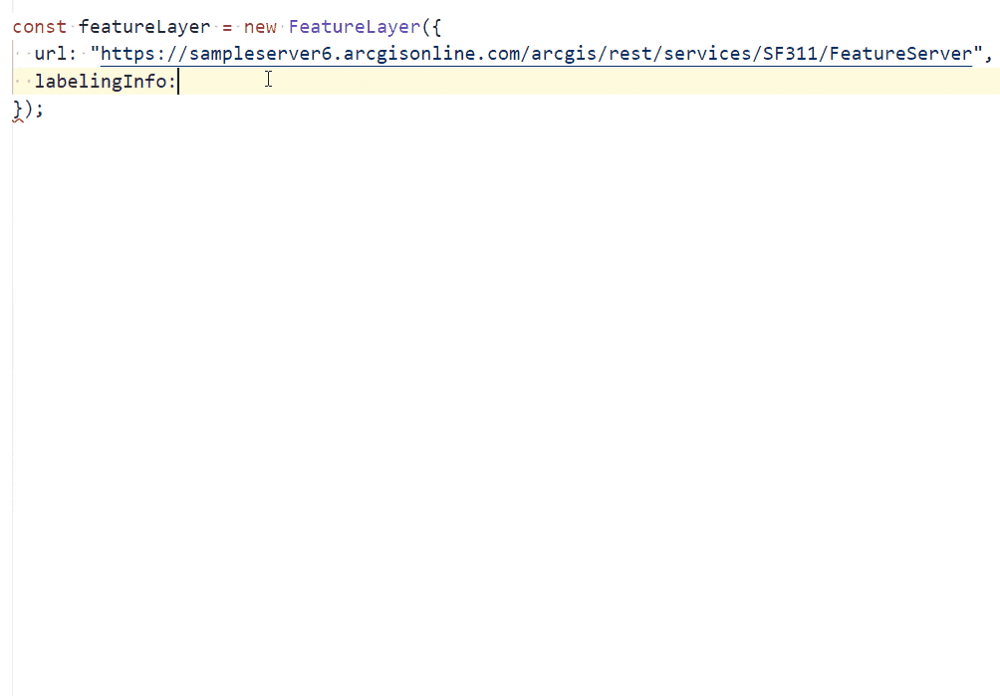
Visual Studio Code is very popular among web developers (most of us in the ArcGIS API for JavaScript team use it), so Kelly and I thought we should create an extension for VSCode with all these code snippets that we use quite often.
Aaand there it is: 🎉ArcGIS API for JavaScript Snippets VSCode Extension 🎉
How to use it? In VSCode, go to Extensions, search for arcgis api for javascript
and press the install button. Check out the list of code snippets that we added there. In your app, type in the prefix of the code snippet you want to add and press Tab
. That should create the code snippet with the placeholders just like in the animated gif above.
You can have a look at all the snippets in the Github repository. If you want to suggest code snippets to us, please create an issue or open up a pull request. If you want to learn how to create your own code snippets, but not necessarily add them to our extension then read more about it in this VSCode tutorial.
Code snippets for the browser console
Sometimes a layer doesn’t show up in the app or you’d like to get that nice viewpoint to reuse it somewhere else, or maybe you even want to change things interactively (light settings, quality mode, etc.) just to try things out. That’s when these code snippets are useful. And for those that use Google Chrome for debugging, you can even store these code snippets in the developer tools in the Snippets
tab.
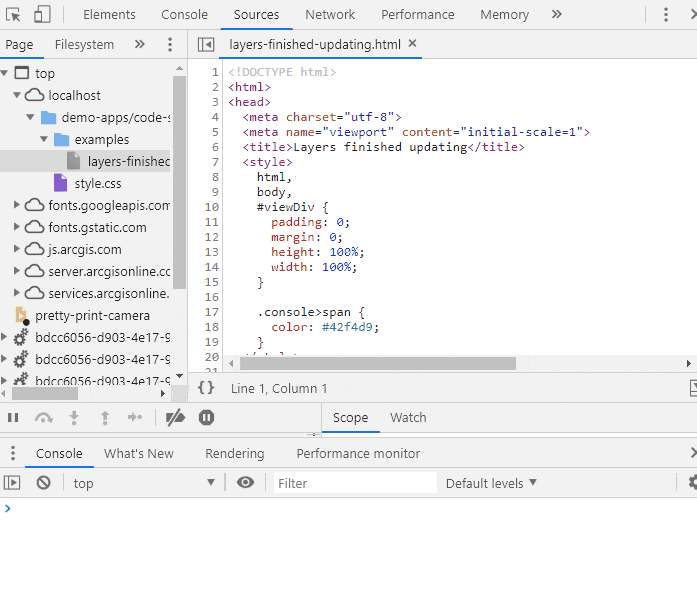
The most useful code snippet is the one for getting access to the view. That will grant you super powers, like access to the layers and their views and so on:
const view = require("esri/views/View").views.getItemAt(0);
Now you can for example display all the layers in your map with their corresponding index:
view.map.allLayers.forEach((layer, index) => {
console.log(`${index} -> ${layer.title}`);
});
If a layer doesn’t display, you can get access to it and check if the LayerView was created successfully:
const layer = view.map.allLayers.getItemAt(index);
view.whenLayerView(layer)
.then((layerView) => console.log(layerView))
// if there were problems with the layerview, you'll get an error here
.catch(console.error);
You can explore more code snippets like this in this file. If you want to learn how to add them to the Snippets
section in Chrome developer tools, you will probably find this tutorial useful. A similar environment for Mozilla Firefox is Scratchpad. You can learn more about it here.
And that’s about it! As a summary, in this blog post we presented:
- a new VSCode extension with common ArcGIS API for JavaScript code snippets that are useful while developing applications.
- a list of code snippets that are useful while debugging ArcGIS API for JavaScript applications directly in the browser console.
Have fun coding!
Commenting is not enabled for this article.