Recently, Rene and I delivered a webinar about using TypeScript with the ArcGIS API for JavaScript. There is also a follow-up GeoDev Webinar Blog that contains all the questions from the participants with some helpful answers. We received such positive feedback that we wanted to write a blog on the same topic so that we can share this information with a wider audience.
In this blog, you’ll learn how to get started using TypeScript with the ArcGIS API for JavaScript, and what the benefits are to using TypeScript for building your web applications. We’ll cover how to migrate an app from JavaScript to TypeScript, the API typings, and how to use our typings to help you build your own applications. Then we will end with some resources for custom widget development, which requires TypeScript.
TypeScript Development Resources
There are a lot of resources to help you on your way. This section will assume that you have already found places to learn the ins and outs of TypeScript itself.
The best place to start is with the TypeScript Setup guide page. Here you can learn about prerequisites, installing TypeScript and the ArcGIS API for JavaScript Typings, and a walk-through about how to write your first TypeScript application. We recommend a couple of blogs for more background information:
- Using TypeScript With EsriJS 4
- Creating a custom tile layer with TypeScript
- Introducing a cli for the ArcGIS API for JavaScript
- Improved TypeScript development with ArcGIS API for JavaScript
There have also been a couple of recorded sessions about Using TypeScript with the ArcGIS API for JavaScript, one from the 2017 Esri Developer Summit and one from the 2018 Esri Developer Summit. There are enough differences between the two sessions that is well worth your time to watch both.
Lastly, here is the recent webinar recording about Using TypeScript with the ArcGIS API for JavaScript, with the associated webinar slides and the TypeScript examples that Rene demoed.
What is TypeScript?
TypeScript is a superset of JavaScript that adds optional static typing. Thus, existing JavaScript applications are also valid TypeScript applications. While JavaScript is a loosely typed language, TypeScript offers the benefits of a strongly typed language, which can be compiled into JavaScript.
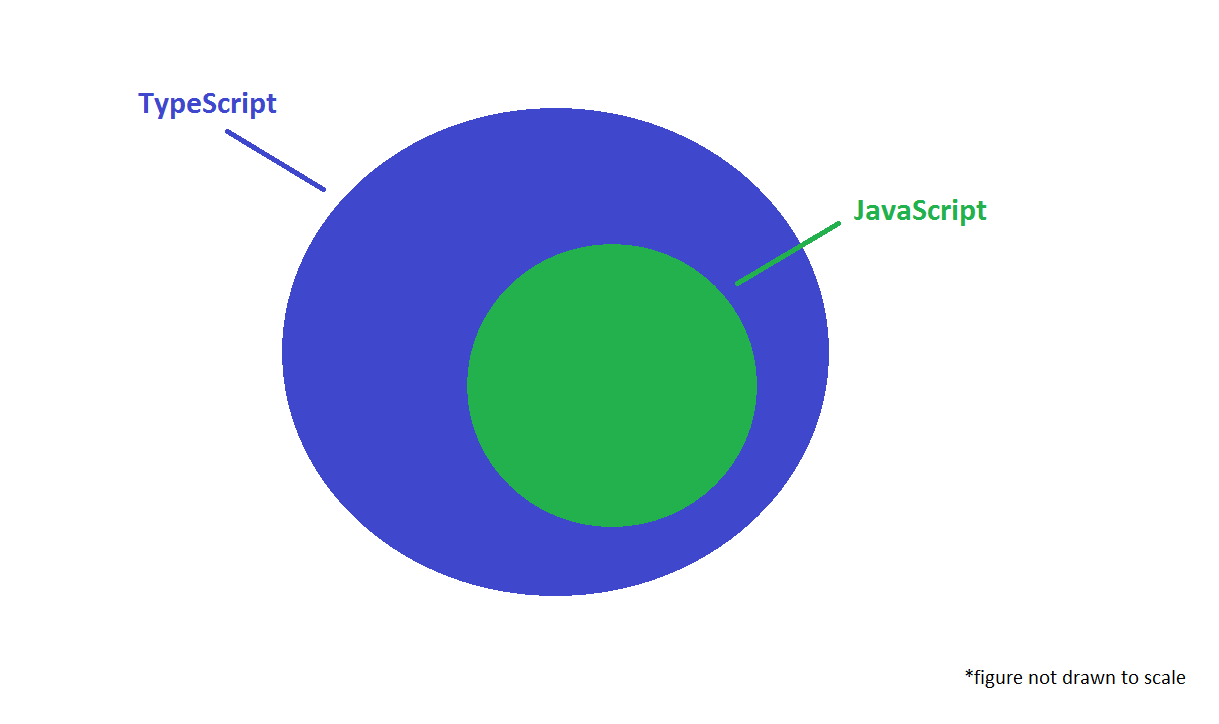
Why use TypeScript?
There are countless blogs and articles about how exciting TypeScript is, like this blog post from Sitepen detailing some of the language’s main features. Internally, we use it a lot (at the time of writing, approximately 88% of our API is written in TypeScript). Here we will distill the hype into three main bullet points:
1. TypeScript adds type
support to JavaScript
With TypeScript, you have the option to add type
support to your code. This allows you to specify the type of value a variable can hold. Adding types to your code makes it easier to maintain and easier to read. In the code snippet below, the function accepts two parameters. The first must be of type string
, and the second must be of type boolean
.
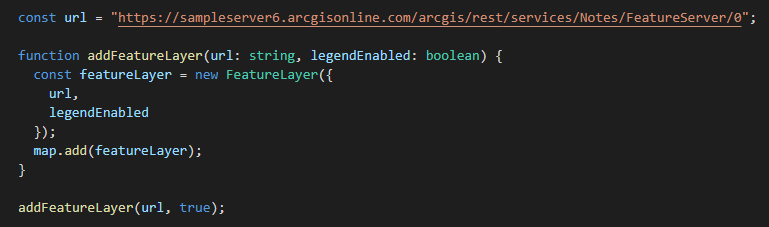
2. Enhanced IDE support
While there are several IDEs that can be configured to work with TypeScript, we use Visual Studio Code for our code examples in this blog. In the example above, we passed the appropriate types to the function. However, if we did not pass the appropriate types to the function, our IDE would give us a compile-time error indicating that an invalid type was passed to the function (we tried to pass off an integer
for a string
). This typed environment also allows IDEs to perform refactoring and other productivity operations.
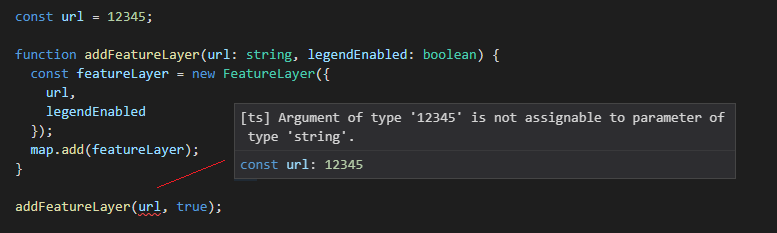
Fun fact: the compiler that gives us this TypeScript intellisense in our IDE is itself written in TypeScript.
3. Makes use of the latest JavaScript features
Lastly, TypeScript makes use of the latest features of JavaScript, such as classes, async/await functions, dynamic imports (aka. lazy loading), and more.
Setting Up TypeScript
There is some basic setup required to get started with TypeScript. Our TypeScript Setup guide does a good job of detailing the necessary steps. Here we will cover the highlights, but for more detailed steps, please refer back to the guide.
First, you will need to install node and npm on your machine. Once you have done that you will want to set up a basic folder that may look something like this.
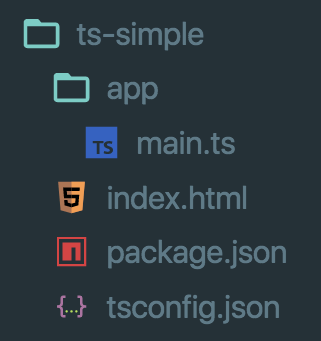
This folder structure represents the minimum to get started writing a TypeScript application. The second step is to install the the ArcGIS API for JavaScript Typings by running the following commands in the root of your application:
npm init --yes
npm install --save @types/arcgis-js-api
The first command will create a package.json
in your folder. The second command will install the typings for the JavaScript API from npm into a node_modules
folder. We’ll take a closer look at actually writing TypeScript in a moment, but first, we’ll take a look at the tsconfig.json. This file will tell the TypeScript compiler how to compile your code to JavaScript.
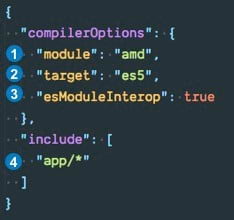
This is the bare minimum configuration you would need to compile TypeScript for use with the ArcGIS API for JavaScript. In this configuration we are doing a few things.
- Telling the compiler to output the JavaScript as AMD modules, that can be consumed by the ArcGIS API for JavaScript.
- We want the output JavaScript to es5 for optimal browser compatibility.
- Turn on esModuleInterop so that you can write your import statements for modules in the JavaScript API as
import WebMap from “esri/WebMap”
instead ofimport WebMap = require(“esri/WebMap”)
. - Finally, you want to tell the compiler where your TypeScript files are located.
If you wanted to use more advanced features, you could update your configuration.
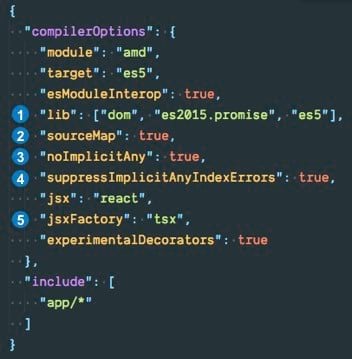
This configuration is more typical of what you would use for an application.
- List of library files to include your compiled code. This is useful if you want to use features like Promise or Geolocation.
- Source maps are useful during debugging, so that you can debug the source TypeScript files instead of the compiled JavaScript.
- The any type is useful when you do not know the type for a variable or when working with dynamic content. This tells the compiler, they are allowed, but you must declare them.
- This goes hand in hand with the next option, which will allow to index objects that have not been explicitly typed.
- These final options for JSX and decorators are used when you build custom widgets with the JavaScript API.
Migrate an app from JavaScript to TypeScript
As was previously discussed, TypeScript is a superset of JavaScript. This can ease the transition for developers that want to begin writing TypeScript today.
Here is a small sample of a basic application written in JavaScript using the ArcGIS API for JavaScript. This may be familiar to many of you already building apps with the API. We use a require function to load modules of the API as needed.
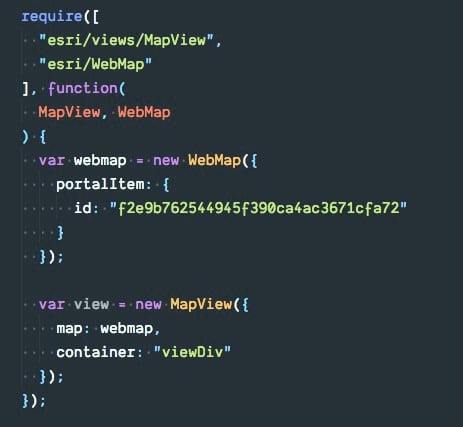
Below is a sample of the same code above, but in TypeScript.
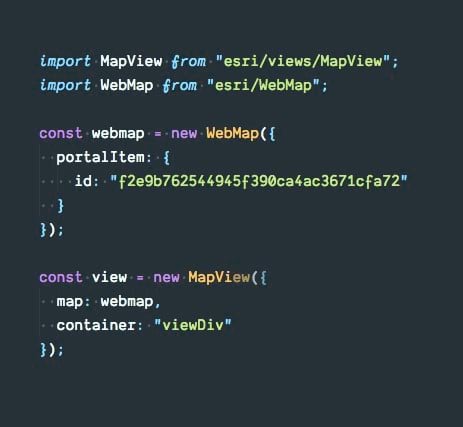
The main difference in this code snippet is that instead of using the require method to load modules as needed, we can now use the import statement to load modules. The other difference is that we now use the const keyword instead of the var keyword. You could also use the let keyword here. The main difference is that const must be initialized with a value and you cannot change that value, whereas let does not need to be initialized with a value and you can change its value.
Widget Development
Not only does TypeScript improve the readability and scalability of your code, it is also required for creating custom widgets with the ArcGIS API for JavaScript. While custom widget development is beyond the scope of this blog, we would like to share some resources with you to help you on your way.
The best place to start is to look at the Widget Development Requirements guide. Here you can learn about prerequisites, like installing the ArcGIS API for JavaScript Typings, and getting familiar with JSX and the Accessor class.
We recommend that you review or bookmark some documentation and a couple of tutorials that will help get you started:
- Guide: Widget Development
- Guide: Implementing Accessor
- API Reference: Base Widget Class
- API Reference: Decorators module
- Tutorial 1: Create a Custom Widget
- Tutorial 2: Custom Recent Widget
There have also been a couple of recorded sessions about widget development that we highly recommend as well:
- Building Your Own Widget with the ArcGIS API for JavaScript (Dev Summit 2018)
- Building a Widget Using the ArcGIS API for JavaScript (GeoDev Webinar 2018)
- ArcGIS API for JavaScript: Customizing Widgets (UC 2018)
Conclusion
TypeScript is an exciting programming language that adds a lot of value to web development. Internally, we use TypeScript heavily in developing the ArcGIS API for JavaScript. This blog explained how to get started using TypeScript with the ArcGIS API for JavaScript, and what the benefits are to using TypeScript for building your web applications. Stay tuned for more exciting developments!
Commenting is not enabled for this article.